Suggested Videos
Part 56 - Gridview paging using a dropdownlist
Part 57 - Export gridview to excel
Part 58 - Export gridview to pdf
In this video, we will discuss about generating a PDF document from gridview data and storing it in a folder on the web server. We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Please watch Part 58, before proceeding with this video.
Step 1: Create an asp.net web application project. Add a folder with name "PDFDocuments" to the project. We will be using this folder to store the dynamically generated pdf document.
Step 2: Drag and drop a gridview control and a button control on webform1.aspx. Autoformat the gridview control to use "BrownSugar" scheme. Double click on the button control, to generate click event handler method.
At this point the HTML on webform1.aspx should be as shown below.
<asp:GridView ID="GridView1" runat="server" BackColor="#DEBA84"
BorderColor="#DEBA84" BorderStyle="None" BorderWidth="1px"
CellPadding="3" CellSpacing="2">
<FooterStyle BackColor="#F7DFB5" ForeColor="#8C4510" />
<HeaderStyle BackColor="#A55129" Font-Bold="True"
ForeColor="White" />
<PagerStyle ForeColor="#8C4510" HorizontalAlign="Center" />
<RowStyle BackColor="#FFF7E7" ForeColor="#8C4510" />
<SelectedRowStyle BackColor="#738A9C" Font-Bold="True"
ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FFF1D4" />
<SortedAscendingHeaderStyle BackColor="#B95C30" />
<SortedDescendingCellStyle BackColor="#F1E5CE" />
<SortedDescendingHeaderStyle BackColor="#93451F" />
</asp:GridView>
<asp:Button ID="Button1" runat="server" Text="Button"
onclick="Button1_Click" />
Step 3: To generate PDF documents we will be using open source assembly - iTextSharp.dll. This assembly can be downloaded from http://sourceforge.net/projects/itextsharp/. After you download the assembly, add a reference to it, from your web application.
a) In Solution Explorer, right click on the "References" folder and select "Add Reference"
b) Browse to the folder where you have downloaded the assembly and Click OK.
Step 4: Add the following "USING" statements, in your code-behind file.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Data;
using System.Configuration;
using System.IO;
using iTextSharp.text;
using iTextSharp.text.html.simpleparser;
using iTextSharp.text.pdf;
Step 5: Copy and paste the following code. The code is well commented and is self-explanatory.
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridViewData();
}
}
private void BindGridViewData()
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlDataAdapter da = new SqlDataAdapter("Select * from tblEmployee", con);
DataSet ds = new DataSet();
da.Fill(ds);
GridView1.DataSource = ds;
GridView1.DataBind();
}
}
protected void Button1_Click(object sender, EventArgs e)
{
PdfPTable pdfTable = new PdfPTable(GridView1.HeaderRow.Cells.Count);
foreach (TableCell gridViewHeaderCell in GridView1.HeaderRow.Cells)
{
Font font = new Font();
font.Color = new BaseColor(GridView1.HeaderStyle.ForeColor);
PdfPCell pdfCell = new PdfPCell(new Phrase(gridViewHeaderCell.Text, font));
pdfCell.BackgroundColor = new BaseColor(GridView1.HeaderStyle.BackColor);
pdfTable.AddCell(pdfCell);
}
foreach (GridViewRow gridViewRow in GridView1.Rows)
{
foreach (TableCell gridViewCell in gridViewRow.Cells)
{
Font font = new Font();
font.Color = new BaseColor(GridView1.RowStyle.ForeColor);
PdfPCell pdfCell = new PdfPCell(new Phrase(gridViewCell.Text, font));
pdfCell.BackgroundColor = new BaseColor(GridView1.RowStyle.BackColor);
pdfTable.AddCell(pdfCell);
}
}
Document pdfDocument = new Document(PageSize.A4, 10f, 10f, 10f, 10f);
// Roate page using Rotate() function, if you want in Landscape
// pdfDocument.SetPageSize(PageSize.A4.Rotate());
// Using PageSize.A4_LANDSCAPE may not work as expected
// Document pdfDocument = new Document(PageSize.A4_LANDSCAPE, 10f, 10f, 10f, 10f);
PdfWriter.GetInstance(pdfDocument, new FileStream(Server.MapPath("~/PDFDocuments/Employees.pdf"), FileMode.Create));
pdfDocument.Open();
pdfDocument.Add(pdfTable);
pdfDocument.Close();
}
At this point, run the application and click on the button. The data gets exported to Employees.PDF document as expected and is stored in the folder "PDFDocuments". Notice that the PDF document, is generated using "Portrait" orientation.
To generate the PDF document in "Landscape" orientation, uncomment the following line
// pdfDocument.SetPageSize(PageSize.A4.Rotate());
To test, add a few other columns to tblEmployee table. You can use the script below.
Alter table tblEmployee
add Department nvarchar(50),
DateOfBirth Date,
DateOfJoining Date,
UserName nvarchar(50)
Update tblEmployee set Department = 'IT', DateOfBirth='03/03/1975',
DateOfJoining='03/08/2000', UserName='Mark' where EmployeeId=1
Update tblEmployee set Department = 'HR', DateOfBirth='08/03/1973',
DateOfJoining='07/09/2001', UserName='John' where EmployeeId=2
Update tblEmployee set Department = 'Payroll', DateOfBirth='02/01/1970',
DateOfJoining='08/02/2006', UserName='Mary' where EmployeeId=3
Update tblEmployee set Department = 'Payroll', DateOfBirth='01/04/1978',
DateOfJoining='01/05/2004', UserName='Mike' where EmployeeId=4
Update tblEmployee set Department = 'IT', DateOfBirth='03/01/1970',
DateOfJoining='02/02/2003', UserName='Pam' where EmployeeId=5
Update tblEmployee set Department = 'IT', DateOfBirth='09/02/1971',
DateOfJoining='06/09/2002', UserName='David' where EmployeeId=6
Update tblEmployee set Department = 'HR', DateOfBirth='10/06/1973',
DateOfJoining='05/01/2001', UserName='Rob' where EmployeeId=7
Update tblEmployee set Department = 'IT', DateOfBirth='11/04/1972',
DateOfJoining='01/02/1999', UserName='Jaimie' where EmployeeId=8
Now, run the application and click on the button. The PDF document should be generated in landscape orientation.
Part 56 - Gridview paging using a dropdownlist
Part 57 - Export gridview to excel
Part 58 - Export gridview to pdf
In this video, we will discuss about generating a PDF document from gridview data and storing it in a folder on the web server. We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Please watch Part 58, before proceeding with this video.
Step 1: Create an asp.net web application project. Add a folder with name "PDFDocuments" to the project. We will be using this folder to store the dynamically generated pdf document.
Step 2: Drag and drop a gridview control and a button control on webform1.aspx. Autoformat the gridview control to use "BrownSugar" scheme. Double click on the button control, to generate click event handler method.
At this point the HTML on webform1.aspx should be as shown below.
<asp:GridView ID="GridView1" runat="server" BackColor="#DEBA84"
BorderColor="#DEBA84" BorderStyle="None" BorderWidth="1px"
CellPadding="3" CellSpacing="2">
<FooterStyle BackColor="#F7DFB5" ForeColor="#8C4510" />
<HeaderStyle BackColor="#A55129" Font-Bold="True"
ForeColor="White" />
<PagerStyle ForeColor="#8C4510" HorizontalAlign="Center" />
<RowStyle BackColor="#FFF7E7" ForeColor="#8C4510" />
<SelectedRowStyle BackColor="#738A9C" Font-Bold="True"
ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FFF1D4" />
<SortedAscendingHeaderStyle BackColor="#B95C30" />
<SortedDescendingCellStyle BackColor="#F1E5CE" />
<SortedDescendingHeaderStyle BackColor="#93451F" />
</asp:GridView>
<asp:Button ID="Button1" runat="server" Text="Button"
onclick="Button1_Click" />
Step 3: To generate PDF documents we will be using open source assembly - iTextSharp.dll. This assembly can be downloaded from http://sourceforge.net/projects/itextsharp/. After you download the assembly, add a reference to it, from your web application.
a) In Solution Explorer, right click on the "References" folder and select "Add Reference"
b) Browse to the folder where you have downloaded the assembly and Click OK.
Step 4: Add the following "USING" statements, in your code-behind file.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Data;
using System.Configuration;
using System.IO;
using iTextSharp.text;
using iTextSharp.text.html.simpleparser;
using iTextSharp.text.pdf;
Step 5: Copy and paste the following code. The code is well commented and is self-explanatory.
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridViewData();
}
}
private void BindGridViewData()
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlDataAdapter da = new SqlDataAdapter("Select * from tblEmployee", con);
DataSet ds = new DataSet();
da.Fill(ds);
GridView1.DataSource = ds;
GridView1.DataBind();
}
}
protected void Button1_Click(object sender, EventArgs e)
{
PdfPTable pdfTable = new PdfPTable(GridView1.HeaderRow.Cells.Count);
foreach (TableCell gridViewHeaderCell in GridView1.HeaderRow.Cells)
{
Font font = new Font();
font.Color = new BaseColor(GridView1.HeaderStyle.ForeColor);
PdfPCell pdfCell = new PdfPCell(new Phrase(gridViewHeaderCell.Text, font));
pdfCell.BackgroundColor = new BaseColor(GridView1.HeaderStyle.BackColor);
pdfTable.AddCell(pdfCell);
}
foreach (GridViewRow gridViewRow in GridView1.Rows)
{
foreach (TableCell gridViewCell in gridViewRow.Cells)
{
Font font = new Font();
font.Color = new BaseColor(GridView1.RowStyle.ForeColor);
PdfPCell pdfCell = new PdfPCell(new Phrase(gridViewCell.Text, font));
pdfCell.BackgroundColor = new BaseColor(GridView1.RowStyle.BackColor);
pdfTable.AddCell(pdfCell);
}
}
Document pdfDocument = new Document(PageSize.A4, 10f, 10f, 10f, 10f);
// Roate page using Rotate() function, if you want in Landscape
// pdfDocument.SetPageSize(PageSize.A4.Rotate());
// Using PageSize.A4_LANDSCAPE may not work as expected
// Document pdfDocument = new Document(PageSize.A4_LANDSCAPE, 10f, 10f, 10f, 10f);
PdfWriter.GetInstance(pdfDocument, new FileStream(Server.MapPath("~/PDFDocuments/Employees.pdf"), FileMode.Create));
pdfDocument.Open();
pdfDocument.Add(pdfTable);
pdfDocument.Close();
}
At this point, run the application and click on the button. The data gets exported to Employees.PDF document as expected and is stored in the folder "PDFDocuments". Notice that the PDF document, is generated using "Portrait" orientation.
To generate the PDF document in "Landscape" orientation, uncomment the following line
// pdfDocument.SetPageSize(PageSize.A4.Rotate());
To test, add a few other columns to tblEmployee table. You can use the script below.
Alter table tblEmployee
add Department nvarchar(50),
DateOfBirth Date,
DateOfJoining Date,
UserName nvarchar(50)
Update tblEmployee set Department = 'IT', DateOfBirth='03/03/1975',
DateOfJoining='03/08/2000', UserName='Mark' where EmployeeId=1
Update tblEmployee set Department = 'HR', DateOfBirth='08/03/1973',
DateOfJoining='07/09/2001', UserName='John' where EmployeeId=2
Update tblEmployee set Department = 'Payroll', DateOfBirth='02/01/1970',
DateOfJoining='08/02/2006', UserName='Mary' where EmployeeId=3
Update tblEmployee set Department = 'Payroll', DateOfBirth='01/04/1978',
DateOfJoining='01/05/2004', UserName='Mike' where EmployeeId=4
Update tblEmployee set Department = 'IT', DateOfBirth='03/01/1970',
DateOfJoining='02/02/2003', UserName='Pam' where EmployeeId=5
Update tblEmployee set Department = 'IT', DateOfBirth='09/02/1971',
DateOfJoining='06/09/2002', UserName='David' where EmployeeId=6
Update tblEmployee set Department = 'HR', DateOfBirth='10/06/1973',
DateOfJoining='05/01/2001', UserName='Rob' where EmployeeId=7
Update tblEmployee set Department = 'IT', DateOfBirth='11/04/1972',
DateOfJoining='01/02/1999', UserName='Jaimie' where EmployeeId=8
Now, run the application and click on the button. The PDF document should be generated in landscape orientation.
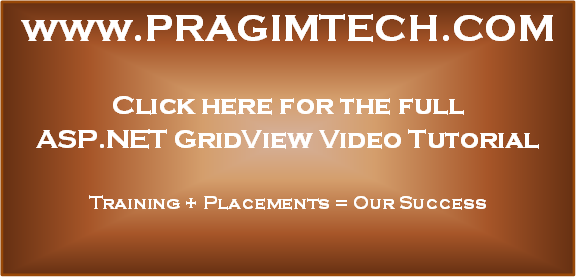
No comments:
Post a Comment
It would be great if you can help share these free resources