Suggested Videos
Part 1 - LINQ to SQL
In this video, we will discuss performing Insert Update and Delete using LINQ to SQL. We will continue with the example, we worked with in Part 1. In Part 1, we discussed performing a Select using LINQ to SQL. Please watch Part 1 before proceeding.
Insert using LINQ to SQL
Update using LINQ to SQL
Delete using LINQ to SQL
Now let us continue with the example that we worked with in Part 1.
Step 1 : Drag and drop 4 button controls on the webform. Change the Text & ID properties of the 4 button controls as shown below.
Button 1 : Text = Get Data, ID = btnGetData
Button 2 : Text = Insert, ID = btnInsert
Button 3 : Text = Update, ID = btnUpdate
Button 4 : Text = Delete, ID = btnDelete
Double click on each of the button controls to generate their respective click event handler methods.
At this point, the design of the WebForm should be as shown below.
Step 2 : Copy and paste the following code in the code-behind file.
Part 1 - LINQ to SQL
In this video, we will discuss performing Insert Update and Delete using LINQ to SQL. We will continue with the example, we worked with in Part 1. In Part 1, we discussed performing a Select using LINQ to SQL. Please watch Part 1 before proceeding.
Insert using LINQ to SQL
using (SampleDataContext dbContext = new SampleDataContext())
{
Employee newEmployee = new
Employee
{
FirstName = "Tim",
LastName = "T",
Gender = "Male",
Salary = 55000,
DepartmentId = 1
};
dbContext.Employees.InsertOnSubmit(newEmployee);
dbContext.SubmitChanges();
}
Update using LINQ to SQL
using (SampleDataContext dbContext = new SampleDataContext())
{
Employee employee = dbContext.Employees.SingleOrDefault(x
=> x.ID == 8);
employee.Salary = 65000;
dbContext.SubmitChanges();
}Delete using LINQ to SQL
using (SampleDataContext dbContext = new SampleDataContext())
{
Employee employee = dbContext.Employees.SingleOrDefault(x
=> x.ID == 8);
dbContext.Employees.DeleteOnSubmit(employee);
dbContext.SubmitChanges();
}
Now let us continue with the example that we worked with in Part 1.
Step 1 : Drag and drop 4 button controls on the webform. Change the Text & ID properties of the 4 button controls as shown below.
Button 1 : Text = Get Data, ID = btnGetData
Button 2 : Text = Insert, ID = btnInsert
Button 3 : Text = Update, ID = btnUpdate
Button 4 : Text = Delete, ID = btnDelete
Double click on each of the button controls to generate their respective click event handler methods.
At this point, the design of the WebForm should be as shown below.

Step 2 : Copy and paste the following code in the code-behind file.
using System;
using System.Linq;
namespace Demo
{
public partial class
WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{}
private void GetData()
{
SampleDataContext dbContext = new SampleDataContext();
GridView1.DataSource = dbContext.Employees;
GridView1.DataBind();
}
protected void btnGetData_Click(object sender,
EventArgs e)
{
GetData();
}
protected void btnInsert_Click(object sender,
EventArgs e)
{
using (SampleDataContext dbContext = new SampleDataContext())
{
Employee newEmployee = new Employee
{
FirstName = "Tim",
LastName = "T",
Gender = "Male",
Salary = 55000,
DepartmentId = 1
};
dbContext.Employees.InsertOnSubmit(newEmployee);
dbContext.SubmitChanges();
}
GetData();
}
protected void btnUpdate_Click(object sender,
EventArgs e)
{
using (SampleDataContext dbContext = new SampleDataContext())
{
Employee employee = dbContext.Employees.SingleOrDefault(x
=> x.ID == 8);
employee.Salary = 65000;
dbContext.SubmitChanges();
}
GetData();
}
protected void btnDelete_Click(object sender,
EventArgs e)
{
using (SampleDataContext dbContext = new SampleDataContext())
{
Employee employee = dbContext.Employees.SingleOrDefault(x
=> x.ID == 8);
dbContext.Employees.DeleteOnSubmit(employee);
dbContext.SubmitChanges();
}
GetData();
}
}
}
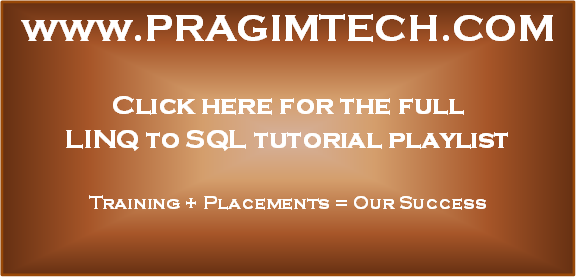
Hello Sir,
ReplyDeleteYour videos are giving me the best my knowledge.Please, make some videos on
Design Patterns in .Net.
Thanks a ton Sir.
Your viedos are really very helpful. Can you please make some viedos on windows Azure
ReplyDeleteplease can you explain iqueryable vs ienumerable c#
ReplyDeleteI'm getting this error while inserting data from my form to database using LinQ
ReplyDeleteDBContext..InsertOnSubmit()
When I enter the object of the class(Employee) It shows the error.
Error 1 The best overloaded method match for 'System.Data.Linq.Table.InsertOnSubmit(Linqtosql1.tblArticle)' has some invalid arguments
Please do reply how to recover it.
Seems very basic. You are using a magic number to edit or delete the record, however, getting ID from gridview is way difficult. Please add some code to show how to retrieve the ID from selected row on gridview control.
ReplyDelete