Suggested Videos
Part 15 - Conversion Operators in LINQ
Part 16 - Cast and OfType operators in LINQ
Part 17 - AsEnumerable and AsQueryable in LINQ
GroupBy operator belong to Grouping Operators category. This operator takes a flat sequence of items, organize that sequence into groups (IGrouping<K,V>) based on a specific key and return groups of sequences.
In short, GroupBy creates and returns a sequence of IGrouping<K,V>
Let us understand GroupBy with examples.
We will use the following Employee class in this demo
Example 1: Get Employee Count By Department
Output:
Example 2: Get Employee Count By Department and also each employee and department name
Output:
Example 3: Get Employee Count By Department and also each employee and department name. Data should be sorted first by Department in ascending order and then by Employee Name in ascending order.
Output:
Part 15 - Conversion Operators in LINQ
Part 16 - Cast and OfType operators in LINQ
Part 17 - AsEnumerable and AsQueryable in LINQ
GroupBy operator belong to Grouping Operators category. This operator takes a flat sequence of items, organize that sequence into groups (IGrouping<K,V>) based on a specific key and return groups of sequences.
In short, GroupBy creates and returns a sequence of IGrouping<K,V>
Let us understand GroupBy with examples.
We will use the following Employee class in this demo
public class Employee
{
public int ID
{ get; set;
}
public string Name
{ get; set;
}
public string Gender
{ get; set;
}
public string Department
{ get; set;
}
public int Salary
{ get; set;
}
public static List<Employee> GetAllEmployees()
{
return new List<Employee>()
{
new Employee { ID = 1, Name = "Mark", Gender = "Male",
Department = "IT", Salary = 45000
},
new Employee { ID = 2, Name = "Steve", Gender = "Male",
Department = "HR", Salary = 55000
},
new Employee { ID = 3, Name = "Ben", Gender = "Male",
Department = "IT", Salary = 65000
},
new Employee { ID = 4, Name = "Philip", Gender = "Male",
Department = "IT", Salary = 55000
},
new Employee { ID = 5, Name = "Mary", Gender = "Female",
Department = "HR", Salary = 48000
},
new Employee { ID = 6, Name = "Valarie", Gender = "Female",
Department = "HR", Salary = 70000
},
new Employee { ID = 7, Name = "John", Gender = "Male",
Department = "IT", Salary = 64000
},
new Employee { ID = 8, Name = "Pam", Gender = "Female",
Department = "IT", Salary = 54000
},
new Employee { ID = 9, Name = "Stacey", Gender = "Female",
Department = "HR", Salary = 84000
},
new Employee { ID = 10, Name = "Andy", Gender = "Male",
Department = "IT", Salary = 36000
}
};
}
}
Example 1: Get Employee Count By Department
var employeeGroup = from
employee in Employee.GetAllEmployees()
group employee by employee.Department;
foreach (var group in employeeGroup)
{
Console.WriteLine("{0} -
{1}", group.Key, group.Count());
}
Output:

Example 2: Get Employee Count By Department and also each employee and department name
var employeeGroup = from
employee in Employee.GetAllEmployees()
group employee by employee.Department;
foreach (var group in employeeGroup)
{
Console.WriteLine("{0} -
{1}", group.Key, group.Count());
Console.WriteLine("----------");
foreach (var employee
in group)
{
Console.WriteLine(employee.Name + "\t" + employee.Department);
}
Console.WriteLine(); Console.WriteLine();
}
Output:

Example 3: Get Employee Count By Department and also each employee and department name. Data should be sorted first by Department in ascending order and then by Employee Name in ascending order.
var employeeGroup = from
employee in Employee.GetAllEmployees()
group employee by employee.Department into eGroup
orderby eGroup.Key
select new
{
Key = eGroup.Key,
Employees = eGroup.OrderBy(x
=> x.Name)
};
foreach (var group in employeeGroup)
{
Console.WriteLine("{0} -
{1}", group.Key, group.Employees.Count());
Console.WriteLine("----------");
foreach (var employee
in group.Employees)
{
Console.WriteLine(employee.Name + "\t" + employee.Department);
}
Console.WriteLine(); Console.WriteLine();
}
Output:

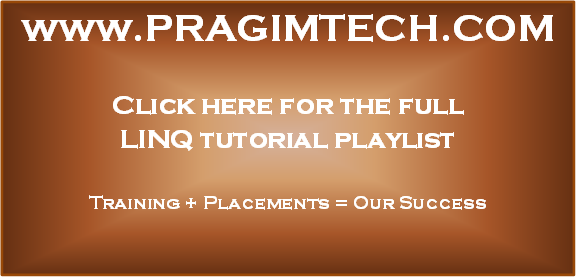
PLease how to convert this expression into lambda expression
ReplyDeletevar employeeGroup = from employee in Employee.GetAllEmployees()
group employee by employee.Department into eGroup
orderby eGroup.Key
select new
{
Key = eGroup.Key,
Employees = eGroup.OrderBy(x => x.Name)
};
Employee.GetAllEmployees().GroupBy(x => x.Department).OrderBy(c => c.Key)
Delete.Select(x => new
{
Key=x.Key,
employee = x.OrderBy(c => c.Name)
});
check more on csharphy.blogpost.in
var result = Employee.GetAll().GroupBy(g => g.Department).Select(p => new {Department = p.Key,Person=p});
Deleteforeach (var item in result)
{
Console.WriteLine("Department : {0}",item.Department);
Console.WriteLine();
foreach (var person in item.Person)
{
Console.WriteLine("ID : {0}", person.ID);
Console.WriteLine("Name : {0}", person.Name);
Console.WriteLine("Gender : {0}", person.Gender);
Console.WriteLine("Salary : {0}", person.Salary);
Console.WriteLine("Department : {0}", person.Department);
Console.WriteLine();
}
Check my Blog :www.cfetch.blogspot.com For more..
var result = Employee.GetAllEmployees().GroupBy(x=>x.Department).OrderBy(x=>x.Key);
ReplyDeleteforeach(var Group in result)
{
Console.WriteLine( Group.Key + " - "+ Group.Count());
Console.WriteLine("-----------------------------------------");
foreach(var g in Group.OrderBy(x=>x.Name))
{
Console.WriteLine("\t" + g.Name);
}
}
var groupedList = Employee.GetAllEmployees().GroupBy(s => s.Department).OrderBy(x=>x.Key);
ReplyDeleteforeach (var group in groupedList)
{
Console.WriteLine(group.Key + "\t"+group.Count());
Console.WriteLine("-------------------");
group.OrderBy(x=>x.Name).ToList().ForEach(x => Console.WriteLine(x.Name));
Console.WriteLine();
}