Suggested Videos
Part 3 - Visual Studio Keyboard Shortcuts
Part 4 - Command window
Part 5 - Immediate window
Watch window is a very useful debugging tool and can be used to evaluate variables and expressions and edit the value of a variables if required.
Let's understand using the watch window with an example. Create a new console application with name = ConsoleApplication1. Copy and paste the following code.
To get to the watch window,
1. Place a break point on PrintSum() function
2. Run the application in debug mode
3. Click on Debug - Windows - Watch - Watch1
OR
Use the keyboard shortcut - Ctrl + D, Ctrl + W
At this point, we should have the watch window displayed.
There are several ways to add variables and expressions to watch window
1. In the PrintSum() function, right click on "n1" variable and select "Add Watch" from the context menu.
2. In the watch window, type the name of the variable in Name column and press "Enter" key
3. Dragging and dropping or by copying and pasting.
If you are following along, the watch window should display the name, value and type of variables n1 and n2 as shown below.
To change the value of a variable in the watch window, simply type the new value in the value column of the watch window and press "Enter" key. For example, to change the value of n1 from 1 to 10. Simply type 10 in the value column and press Enter key.
Watch window can also be used to evaluate expressions. For example to check if n1 IS EQUAL TO n2.
Enter the expression n1==n2 in the name column of watch window and press Enter key. Notice that the expression return type and value is displayed.
When a value of a variable that is added to the watch window changes, the new value is reflected in the watch window. For example, at the moment
1. There is a breakpoint at the opening curly brace of the PrintSum() function
2. Now add "sum" variable to the watch window
3. Notice that the value of the "sum" variable is 0
4. Press F10 twice, and notice that the value of "sum" variable is changed to 15.
To delete all items from the watch window, right click on the watch window and select "Clear All" from the context menu.
You can also call a function from watch window. Simple type the name of the function, pass the required parameter values and press Enter key.
Part 3 - Visual Studio Keyboard Shortcuts
Part 4 - Command window
Part 5 - Immediate window
Watch window is a very useful debugging tool and can be used to evaluate variables and expressions and edit the value of a variables if required.
Let's understand using the watch window with an example. Create a new console application with name = ConsoleApplication1. Copy and paste the following code.
using System;
namespace ConsoleApplication1
{
class Program
{
static void Main()
{
int Sum = PrintSum(1, 2, 3);
Console.WriteLine(Sum);
}
static int PrintSum(int n1, int
n2, int n3)
{
int sum = n1 + n2 + n3;
return sum;
}
}
}
To get to the watch window,
1. Place a break point on PrintSum() function
2. Run the application in debug mode
3. Click on Debug - Windows - Watch - Watch1
OR
Use the keyboard shortcut - Ctrl + D, Ctrl + W
At this point, we should have the watch window displayed.
There are several ways to add variables and expressions to watch window
1. In the PrintSum() function, right click on "n1" variable and select "Add Watch" from the context menu.

2. In the watch window, type the name of the variable in Name column and press "Enter" key

3. Dragging and dropping or by copying and pasting.
If you are following along, the watch window should display the name, value and type of variables n1 and n2 as shown below.

To change the value of a variable in the watch window, simply type the new value in the value column of the watch window and press "Enter" key. For example, to change the value of n1 from 1 to 10. Simply type 10 in the value column and press Enter key.

Watch window can also be used to evaluate expressions. For example to check if n1 IS EQUAL TO n2.
Enter the expression n1==n2 in the name column of watch window and press Enter key. Notice that the expression return type and value is displayed.

When a value of a variable that is added to the watch window changes, the new value is reflected in the watch window. For example, at the moment
1. There is a breakpoint at the opening curly brace of the PrintSum() function
2. Now add "sum" variable to the watch window
3. Notice that the value of the "sum" variable is 0

4. Press F10 twice, and notice that the value of "sum" variable is changed to 15.

To delete all items from the watch window, right click on the watch window and select "Clear All" from the context menu.

You can also call a function from watch window. Simple type the name of the function, pass the required parameter values and press Enter key.

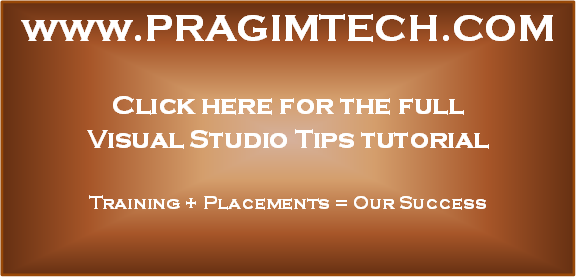
plz help me my website is host but i get to login its show me 'Login failed for user 'NT AUTHORITY\ANONYMOUS LOGON'. what is mean by this plz help me my mail is desaimandar2@gmail.com
ReplyDelete