Suggested Videos
Part 161 - Binding treeview control to web.sitemap file
Part 162 - Binding asp.net treeview control to database table
Part 163 - Dynamically adding treenodes to treeview control
In this video, we will discuss, displaying organization employee chart using TreeView control.
We will use the following table tblEmployee in this demo
We want to display above data in a TreeView control as shown below. A check box should be displayed next to every TreeNode. On clicking the button, the selected employees must be added to the listbox.
SQL script to create and populate table tblEmployee
Create table tblEmployee
(
ID int identity primary key,
Name nvarchar(50),
ManagerId int foreign key references tblEmployee(ID)
)
Insert into tblEmployee values('David', NULL)
Insert into tblEmployee values('Sam', 1)
Insert into tblEmployee values('Pam', 1)
Insert into tblEmployee values('Mike', 1)
Insert into tblEmployee values('John', 2)
Insert into tblEmployee values('Tara', 2)
Insert into tblEmployee values('Todd', 4)
Stored procedure to retrieve data from table tblEmployee
Create Proc spGetEmployees
as
Begin
Select ID, Name, ManagerId from tblEmployee
End
ASPX HTML
<div style="font-family:Arial">
<table>
<tr>
<td style="border:1px solid black">
<asp:TreeView ID="TreeView1" ShowCheckBoxes="All"
runat="server">
</asp:TreeView>
</td>
<td>
<asp:Button ID="Button1" runat="server" onclick="Button1_Click"
Text=">>" />
</td>
<td>
<asp:ListBox ID="ListBox1" runat="server" Height="145px"
Width="100px">
</asp:ListBox>
</td>
</tr>
</table>
</div>
ASPX.CS Code:
using System;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace WebFormsDemo
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GetTreeViewItems();
}
}
private void GetTreeViewItems()
{
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
SqlConnection con = new SqlConnection(cs);
SqlDataAdapter da = new SqlDataAdapter("spGetEmployees", con);
DataSet ds = new DataSet();
da.Fill(ds);
ds.Relations.Add("ChildRows", ds.Tables[0].Columns["ID"],
ds.Tables[0].Columns["ManagerId"]);
foreach (DataRow level1DataRow in ds.Tables[0].Rows)
{
if (string.IsNullOrEmpty(level1DataRow["ManagerId"].ToString()))
{
TreeNode parentTreeNode = new TreeNode();
parentTreeNode.Text = level1DataRow["Name"].ToString();
parentTreeNode.Value = level1DataRow["ID"].ToString();
GetChildRows(level1DataRow, parentTreeNode);
TreeView1.Nodes.Add(parentTreeNode);
}
}
}
private void GetChildRows(DataRow dataRow, TreeNode treeNode)
{
DataRow[] childRows = dataRow.GetChildRows("ChildRows");
foreach (DataRow row in childRows)
{
TreeNode childTreeNode = new TreeNode();
childTreeNode.Text = row["Name"].ToString();
childTreeNode.Value = row["ID"].ToString();
treeNode.ChildNodes.Add(childTreeNode);
if (row.GetChildRows("ChildRows").Length > 0)
{
GetChildRows(row, childTreeNode);
}
}
}
protected void Button1_Click(object sender, EventArgs e)
{
ListBox1.Items.Clear();
GetSelectedTreeNodes(TreeView1.Nodes[0]);
}
private void GetSelectedTreeNodes(TreeNode parentTreeNode)
{
if (parentTreeNode.Checked)
{
ListBox1.Items.Add(parentTreeNode.Text + " - " + parentTreeNode.Value);
}
if (parentTreeNode.ChildNodes.Count > 0)
{
foreach (TreeNode childTreeNode in parentTreeNode.ChildNodes)
{
GetSelectedTreeNodes(childTreeNode);
}
}
}
}
}
Part 161 - Binding treeview control to web.sitemap file
Part 162 - Binding asp.net treeview control to database table
Part 163 - Dynamically adding treenodes to treeview control
In this video, we will discuss, displaying organization employee chart using TreeView control.
We will use the following table tblEmployee in this demo
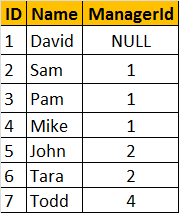
We want to display above data in a TreeView control as shown below. A check box should be displayed next to every TreeNode. On clicking the button, the selected employees must be added to the listbox.

SQL script to create and populate table tblEmployee
Create table tblEmployee
(
ID int identity primary key,
Name nvarchar(50),
ManagerId int foreign key references tblEmployee(ID)
)
Insert into tblEmployee values('David', NULL)
Insert into tblEmployee values('Sam', 1)
Insert into tblEmployee values('Pam', 1)
Insert into tblEmployee values('Mike', 1)
Insert into tblEmployee values('John', 2)
Insert into tblEmployee values('Tara', 2)
Insert into tblEmployee values('Todd', 4)
Stored procedure to retrieve data from table tblEmployee
Create Proc spGetEmployees
as
Begin
Select ID, Name, ManagerId from tblEmployee
End
ASPX HTML
<div style="font-family:Arial">
<table>
<tr>
<td style="border:1px solid black">
<asp:TreeView ID="TreeView1" ShowCheckBoxes="All"
runat="server">
</asp:TreeView>
</td>
<td>
<asp:Button ID="Button1" runat="server" onclick="Button1_Click"
Text=">>" />
</td>
<td>
<asp:ListBox ID="ListBox1" runat="server" Height="145px"
Width="100px">
</asp:ListBox>
</td>
</tr>
</table>
</div>
ASPX.CS Code:
using System;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace WebFormsDemo
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GetTreeViewItems();
}
}
private void GetTreeViewItems()
{
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
SqlConnection con = new SqlConnection(cs);
SqlDataAdapter da = new SqlDataAdapter("spGetEmployees", con);
DataSet ds = new DataSet();
da.Fill(ds);
ds.Relations.Add("ChildRows", ds.Tables[0].Columns["ID"],
ds.Tables[0].Columns["ManagerId"]);
foreach (DataRow level1DataRow in ds.Tables[0].Rows)
{
if (string.IsNullOrEmpty(level1DataRow["ManagerId"].ToString()))
{
TreeNode parentTreeNode = new TreeNode();
parentTreeNode.Text = level1DataRow["Name"].ToString();
parentTreeNode.Value = level1DataRow["ID"].ToString();
GetChildRows(level1DataRow, parentTreeNode);
TreeView1.Nodes.Add(parentTreeNode);
}
}
}
private void GetChildRows(DataRow dataRow, TreeNode treeNode)
{
DataRow[] childRows = dataRow.GetChildRows("ChildRows");
foreach (DataRow row in childRows)
{
TreeNode childTreeNode = new TreeNode();
childTreeNode.Text = row["Name"].ToString();
childTreeNode.Value = row["ID"].ToString();
treeNode.ChildNodes.Add(childTreeNode);
if (row.GetChildRows("ChildRows").Length > 0)
{
GetChildRows(row, childTreeNode);
}
}
}
protected void Button1_Click(object sender, EventArgs e)
{
ListBox1.Items.Clear();
GetSelectedTreeNodes(TreeView1.Nodes[0]);
}
private void GetSelectedTreeNodes(TreeNode parentTreeNode)
{
if (parentTreeNode.Checked)
{
ListBox1.Items.Add(parentTreeNode.Text + " - " + parentTreeNode.Value);
}
if (parentTreeNode.ChildNodes.Count > 0)
{
foreach (TreeNode childTreeNode in parentTreeNode.ChildNodes)
{
GetSelectedTreeNodes(childTreeNode);
}
}
}
}
}

When and where to use Global.asax page in asp.net?. Please can u answer this question as soon.
ReplyDeletePlease help help help!!!
ReplyDeleteI am building nodes dynamically from a DB table. When I run the code the node root node also appears which wont go away. I have tried everything. Looked up on the internet but haven't find a specific solution for this problem.
My ProductCategory table looks like this http://imgur.com/xUvKK28
here's the code in .cs file
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GetTreeViewItems();
}
}
private void GetTreeViewItems()
{
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
SqlConnection con = new SqlConnection(cs);
SqlDataAdapter da = new SqlDataAdapter("select * from ProductCategories where ParentId in (0,1,2)", con);
DataSet ds = new DataSet();
da.Fill(ds);
ds.Relations.Add("ChildRows", ds.Tables[0].Columns["ProductCategoryId"],
ds.Tables[0].Columns["ParentId"]);
foreach (DataRow level1DataRow in ds.Tables[0].Rows)
{
if (string.IsNullOrEmpty(level1DataRow["ParentId"].ToString()))
{
TreeNode parentTreeNode = new TreeNode();
parentTreeNode.Text = level1DataRow["ProductCategoryName"].ToString();
parentTreeNode.Value = level1DataRow["ProductCategoryId"].ToString();
parentTreeNode.NavigateUrl = "?catid=" + level1DataRow["ProductCategoryId"].ToString();
int i = (int)level1DataRow["ProductCategoryId"];
GetChildRows(level1DataRow, parentTreeNode);
TreeView1.Nodes.Add(parentTreeNode);
}
}
}
private void GetChildRows(DataRow dataRow, TreeNode treeNode)
{
DataRow[] childRows = dataRow.GetChildRows("ChildRows");
foreach (DataRow row in childRows)
{
TreeNode childTreeNode = new TreeNode();
childTreeNode.Text = row["ProductCategoryName"].ToString();
childTreeNode.Value = row["ProductCategoryId"].ToString();
childTreeNode.NavigateUrl = "?catid=" + row["ProductCategoryId"].ToString();
treeNode.ChildNodes.Add(childTreeNode);
if (row.GetChildRows("ChildRows").Length > 0)
{
GetChildRows(row, childTreeNode);
}
}
}
}