Suggested Videos
Part 60 - ViewStart in asp.net mvc
Part 61 - Named sections in layout files in mvc
Part 62 - Implementing search functionality in asp.net mvc
In this video we will discuss, implementing pagination in an asp.net mvc application. Please watch Part 62, before proceeding.
By the end of this video, the index page should support both search functionality and pagination as shown below.
Step 1: Install PagedList.Mvc using NuGet package manager. PagedList.Mvc is dependent on PagedList. Installing PagedList.Mvc will automatically install PagedList package as well.
Step 2: Include the following using statements in HomeController.cs file
using PagedList.Mvc;
using PagedList;
Modify the Index() action method as shown below. Notice that we are passing page parameter to this function. This parameter is used for specifying the page number. This parameter can be null, and that's the reason we have chosen a nullable integer. We convert the list, to a paged list, using ToPagedList(). Also, notice that, we are using null-coalescing operator. If the "page" parameter is null, then 1 is passed as the page number, else, the value contained in the "page" parameter is used as the page number.
public ActionResult Index(string searchBy, string search, int? page)
{
if (searchBy == "Gender")
{
return View(db.Employees.Where(x => x.Gender == search || search == null).ToList().ToPagedList(page ?? 1, 3));
}
else
{
return View(db.Employees.Where(x => x.Name.StartsWith(search) || search == null).ToList().ToPagedList(page ?? 1, 3));
}
}
Step 3: Make the following modifications to Index.cshtml view
a) Include the following 2 using statements on the view.
@using PagedList.Mvc;
@using PagedList;
b) The model for the view should be IPagedList<Employee>.
@model IPagedList<MVCDemo.Models.Employee>
c) Since, we have changed the model of the view, from IEnumerable<MVCDemo.Models.Employee> to IPagedList<MVCDemo.Models.Employee>, change the section that displays table headings as shown below.
<tr>
<th>
@Html.DisplayNameFor(model => model.First().Name)
</th>
<th>
@Html.DisplayNameFor(model => model.First().Gender)
</th>
<th>
@Html.DisplayNameFor(model => model.First().Email)
</th>
<th>Action</th>
</tr>
d) Finally to display page numbers for paging
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }))
e) If you want to display the pager, only if there are more than 1 page
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }), new PagedListRenderOptions() { Display = PagedListDisplayMode.IfNeeded })
f) If you want to display, the current active page and the total number of pages
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }), new PagedListRenderOptions() { Display = PagedListDisplayMode.IfNeeded, DisplayPageCountAndCurrentLocation = true })
g) If you want to display the number of rows displayed, of the total number of rows available.
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }), new PagedListRenderOptions() { Display = PagedListDisplayMode.IfNeeded, DisplayItemSliceAndTotal = true })
Part 60 - ViewStart in asp.net mvc
Part 61 - Named sections in layout files in mvc
Part 62 - Implementing search functionality in asp.net mvc
In this video we will discuss, implementing pagination in an asp.net mvc application. Please watch Part 62, before proceeding.
By the end of this video, the index page should support both search functionality and pagination as shown below.
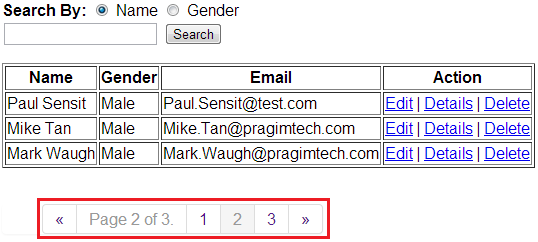
Step 1: Install PagedList.Mvc using NuGet package manager. PagedList.Mvc is dependent on PagedList. Installing PagedList.Mvc will automatically install PagedList package as well.

Step 2: Include the following using statements in HomeController.cs file
using PagedList.Mvc;
using PagedList;
Modify the Index() action method as shown below. Notice that we are passing page parameter to this function. This parameter is used for specifying the page number. This parameter can be null, and that's the reason we have chosen a nullable integer. We convert the list, to a paged list, using ToPagedList(). Also, notice that, we are using null-coalescing operator. If the "page" parameter is null, then 1 is passed as the page number, else, the value contained in the "page" parameter is used as the page number.
public ActionResult Index(string searchBy, string search, int? page)
{
if (searchBy == "Gender")
{
return View(db.Employees.Where(x => x.Gender == search || search == null).ToList().ToPagedList(page ?? 1, 3));
}
else
{
return View(db.Employees.Where(x => x.Name.StartsWith(search) || search == null).ToList().ToPagedList(page ?? 1, 3));
}
}
Step 3: Make the following modifications to Index.cshtml view
a) Include the following 2 using statements on the view.
@using PagedList.Mvc;
@using PagedList;
b) The model for the view should be IPagedList<Employee>.
@model IPagedList<MVCDemo.Models.Employee>
c) Since, we have changed the model of the view, from IEnumerable<MVCDemo.Models.Employee> to IPagedList<MVCDemo.Models.Employee>, change the section that displays table headings as shown below.
<tr>
<th>
@Html.DisplayNameFor(model => model.First().Name)
</th>
<th>
@Html.DisplayNameFor(model => model.First().Gender)
</th>
<th>
@Html.DisplayNameFor(model => model.First().Email)
</th>
<th>Action</th>
</tr>
d) Finally to display page numbers for paging
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }))
e) If you want to display the pager, only if there are more than 1 page
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }), new PagedListRenderOptions() { Display = PagedListDisplayMode.IfNeeded })
f) If you want to display, the current active page and the total number of pages
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }), new PagedListRenderOptions() { Display = PagedListDisplayMode.IfNeeded, DisplayPageCountAndCurrentLocation = true })
g) If you want to display the number of rows displayed, of the total number of rows available.
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchBy = Request.QueryString["searchBy"], search = Request.QueryString["search"] }), new PagedListRenderOptions() { Display = PagedListDisplayMode.IfNeeded, DisplayItemSliceAndTotal = true })
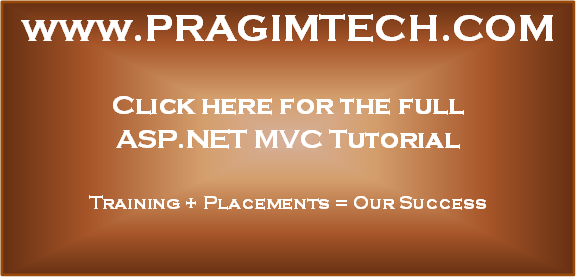
How do i do to implement PagedList with an object list? I have a list instead an enumeration. Sorry for my english.Thanks
ReplyDeleteWhere does the styling come from? I get the bullets for the page as ul's, but no styling in the pretty boxes you have.
ReplyDeletePageList.css also added to your project when you install PagedList.mvc. Please add the css to your view
DeleteIt can be an IIS issue. to resolve - 1) Right click on the project. 2) Web -> Server[section] -> Change IIS Local to IIS Express
DeleteWhen I Implemented paging as explained in tutorial , the page links are coming vertically whereas in the demo its coming horizontal...
ReplyDeleteDont know where i am missing something?
Please add the css to your view
Delete@Manis, you have to reference PagedList.css file in the view page that's css file is located in Content folder of the project,
ReplyDeletei need read,write,edit,update all of them in single VIEW in MVC. its not move to the next page when i click the edit,create like that. could you please help me
ReplyDeleteI have a question about paging. When I implements paging at the bottom of the web page I see the item in a vertical bullet list. How can I fix that problem. I work with Entity Framework 5 and download the latest paging program from nuget solutions. Do I have a version conflict?
ReplyDeleteI have a problem when paging
ReplyDeletei have 4 data
I assign 3 data for the each page.
but when I went looking data number 4 in page 2.
Its always reset and come back into dozens of pages again
what should i do?
Hi I have a big question you should read carefully it is very important about this page list if we have hundred records so fine if there thousands or millions records and we use this paged list library and take 100 records by one page so it will hit the db and make the pagination divided by 100 records so when we click to next paging it will again hit the db and then collect the all records data and again it will perform his operation to make pagination it is very worst performance because it will hit every time when click for next paging. I hope you got my point and it is too important so kindly suggest me other solution because it is not useful for big records data
ReplyDeletei am calling function through Jquery ajax but want to use mvc pagination.
ReplyDeletewhen i using the same method the null refrence accuring.
Severity Code Description Project File Line Suppression State
ReplyDeleteError CS1929 'IHtmlHelper>' does not contain a definition for 'PagedListPager' and the best extension method overload 'HtmlHelper.PagedListPager(HtmlHelper, IPagedList, Func, PagedListRenderOptions)' requires a receiver of type 'HtmlHelper' Sample.SchedularTask E:\Downloads\CINCAI_SampleMT\Sample.SchedularTask\Views\Schedules\Index.cshtml 164 Active
search value is not passing to the second page
ReplyDeleteview
@Html.TextBox("searchTearm", "", new { id = "search" })
@Html.PagedListPager(Model, page => Url.Action("Index", new { page, searchTearm = Request.QueryString["searchTearm"] }), new PagedListRenderOptions() { Display = PagedListDisplayMode.IfNeeded, DisplayPageCountAndCurrentLocation = true })
controller
public ActionResult Index(int? page, string searchTearm)
{
//int pageSize = 5;
//int pageIndex = 1;
//pageIndex = page.HasValue ? Convert.ToInt32(page) : 1;
//var searchTearm = Request.QueryString["searchTearm"].ToString();
//var searchTearm = "searchTearm";
ProductBusinessLayer productbusinesslayer = new ProductBusinessLayer();
//Product objProduct = new Product();
//products.ToPagedList(pageIndex, pageSize);
//List products = productbusinesslayer.Products.ToList(ToPagedList(page ?? 1, 3));
// List products = productbusinesslayer.Products.Where(x => x.Name.StartsWith(searchTearm)).ToList();
if (searchTearm == null)
{
return View(productbusinesslayer.Products.ToList().ToPagedList(page ?? 1, 5));
}
else
{
return View(productbusinesslayer.Products.Where(x => x.Name.StartsWith(searchTearm) || searchTearm == null).ToList().ToPagedList(page ?? 1, 5));
}
}