Suggested Videos
Part 57 - Razor views in mvc
Part 58 - Razor views in mvc continued
Part 59 - Layout view in mvc
In this video, we will discuss the advantage of using _ViewStart.cshtml. Please watch Part 59, before proceeding.
In Part 59, we discussed that, to associate a view with a layout file, we have to set Layout property on each and every view. This violates DRY (Don't Repeat Yourself) principle, and has the following disadvantages
1. Redundant code.
2. Maintenance overhead. To use a different layout file, all the views need to be updated.
What is _ViewStart.cshtml file?
ASP.NET MVC 3, has introduced _ViewStart.cshtml. Specify the Layout property in this file and place it in the Views folder. All the views will then use the layout file that is specified in _ViewStart.cshtml. This eliminates the need to specify Layout property on each and every view, and making them more cleaner and maintainable.
If you want a set of views in a specific folder, to use a different layout file, then you can include another _ViewStart.cshtml file in that specific folder.
When I use _ViewStart.cshtml file, can I still set Layout property on individual views?
Yes, if you want to use a layout file that is different from what is specified in _ViewStart.cshtml
Where else can I specify a layout file?
Layout file can also be specified in a controller action method or in an action filter.
In Controller Action Method:
Specify which layout to use when returning a view inside a controller action
public ActionResult Create()
{
return View("Create", "_Layout");
}
We will discuss action filters in a later video session.
Can we write some logic in "_ViewStart.cshtml" to dynamically specify which layout file to use?
Yes, the following code will change the layout file to use based on the browser type.
If the browser is google chrome,
then "_Layout.cshtml" layout file is used
Else
"_DifferentLayout.cshtml" layout file is used
Code in "_ViewStart.cshtml" file
@{
Layout = Request.Browser.IsBrowser("Chrome") ? "~/Views/Shared/_Layout.cshtml" : "~/Views/Shared/_DifferentLayout.cshtml" ;
}
All partial views in my application are now using the layout file specified in "_ViewStart.cshtml". How do I prevent these partial views from using a layout file?
Details action method below, returns "_Employee" partial view, and is using the layout file specified in "_ViewStart.cshtml"
public ActionResult Details(int id)
{
Employee employee = db.Employees.Single(e => e.Id == id);
return View("_Employee", employee);
}
To prevent this partial view from using the layout file, specified in "_ViewStart.cshtml", return "PartialViewResult" from the controller action method as shown below.
public PartialViewResult Details(int id)
{
Employee employee = db.Employees.Single(e => e.Id == id);
return PartialView("_Employee", employee);
}
What will be the layout file extension, if VB.NET is my programming language?
.vbhtml
In our next video, we will discuss using named sections in a layout file.
Part 57 - Razor views in mvc
Part 58 - Razor views in mvc continued
Part 59 - Layout view in mvc
In this video, we will discuss the advantage of using _ViewStart.cshtml. Please watch Part 59, before proceeding.
In Part 59, we discussed that, to associate a view with a layout file, we have to set Layout property on each and every view. This violates DRY (Don't Repeat Yourself) principle, and has the following disadvantages
1. Redundant code.
2. Maintenance overhead. To use a different layout file, all the views need to be updated.
What is _ViewStart.cshtml file?
ASP.NET MVC 3, has introduced _ViewStart.cshtml. Specify the Layout property in this file and place it in the Views folder. All the views will then use the layout file that is specified in _ViewStart.cshtml. This eliminates the need to specify Layout property on each and every view, and making them more cleaner and maintainable.

If you want a set of views in a specific folder, to use a different layout file, then you can include another _ViewStart.cshtml file in that specific folder.
When I use _ViewStart.cshtml file, can I still set Layout property on individual views?
Yes, if you want to use a layout file that is different from what is specified in _ViewStart.cshtml
Where else can I specify a layout file?
Layout file can also be specified in a controller action method or in an action filter.
In Controller Action Method:
Specify which layout to use when returning a view inside a controller action
public ActionResult Create()
{
return View("Create", "_Layout");
}
We will discuss action filters in a later video session.
Can we write some logic in "_ViewStart.cshtml" to dynamically specify which layout file to use?
Yes, the following code will change the layout file to use based on the browser type.
If the browser is google chrome,
then "_Layout.cshtml" layout file is used
Else
"_DifferentLayout.cshtml" layout file is used
Code in "_ViewStart.cshtml" file
@{
Layout = Request.Browser.IsBrowser("Chrome") ? "~/Views/Shared/_Layout.cshtml" : "~/Views/Shared/_DifferentLayout.cshtml" ;
}
All partial views in my application are now using the layout file specified in "_ViewStart.cshtml". How do I prevent these partial views from using a layout file?
Details action method below, returns "_Employee" partial view, and is using the layout file specified in "_ViewStart.cshtml"
public ActionResult Details(int id)
{
Employee employee = db.Employees.Single(e => e.Id == id);
return View("_Employee", employee);
}
To prevent this partial view from using the layout file, specified in "_ViewStart.cshtml", return "PartialViewResult" from the controller action method as shown below.
public PartialViewResult Details(int id)
{
Employee employee = db.Employees.Single(e => e.Id == id);
return PartialView("_Employee", employee);
}
What will be the layout file extension, if VB.NET is my programming language?
.vbhtml
In our next video, we will discuss using named sections in a layout file.
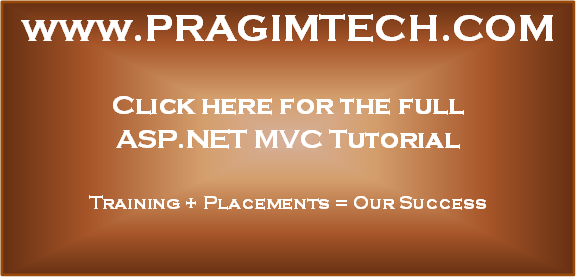
Hi Sir,
ReplyDeleteYour doing a great job. I am very much Thank full to you. In this section i.e. part-60-viewstart-in-aspnet-mvc.html, As you told we can specify layout file path in viewstart, so that maintenance will be easy. But I have a question, why can't we specifying layout file path in web.config. If we specify in web.config, we can read that entry in Razor View right. So, the problem will be resolved right. Can you give me reply for this.
you are doing a good job Venkat, well done..
ReplyDelete