Suggested Videos
Part 36 - Difference between Html.TextBox and Html.TextBoxFor
Part 37 - Generating a radiobuttonlist control in mvc using HTML helpers
Part 38 - CheckBoxList in asp.net mvc
In this video we will discuss implementing ListBox in asp.net mvc. We will be using table "tblCity" for this demo.
Please refer to Part 38, if you need the sql script to create and populate this table. Please watch Part 38, before proceeding.
The generated listbox should be as shown below. Notice that for each city in table "tblCity", there is an entry in the listbox.
For this demo, we will be using the "City" entity that we generated using ADO.NET entity framework, in Part 38.
The first step is to create a ViewModel class. In asp.net mvc, view model's are used as techniques to shuttle data between the controller and the view. Right click on the "Models" folder, and add a class file with name=CitiesViewModel.cs. Copy and paste the following code. This class is going to be the Model for the view.
public class CitiesViewModel
{
public IEnumerable<string> SelectedCities { get; set; }
public IEnumerable<SelectListItem> Cities { get; set; }
}
Right click on the "Controllers" folder, and add a "HomeController". Include the following 2 namespaces in "HomeController"
using MVCDemo.Models;
using System.Text;
Copy and paste the following code.
[HttpGet]
public ActionResult Index()
{
SampleDBContext db = new SampleDBContext();
List<SelectListItem> listSelectListItems = new List<SelectListItem>();
foreach (City city in db.Cities)
{
SelectListItem selectList = new SelectListItem()
{
Text = city.Name,
Value = city.ID.ToString(),
Selected = city.IsSelected
};
listSelectListItems.Add(selectList);
}
CitiesViewModel citiesViewModel = new CitiesViewModel()
{
Cities = listSelectListItems
};
return View(citiesViewModel);
}
[HttpPost]
public string Index(IEnumerable<string> selectedCities)
{
if (selectedCities == null)
{
return "No cities selected";
}
else
{
StringBuilder sb = new StringBuilder();
sb.Append("You selected - " + string.Join(",", selectedCities));
return sb.ToString();
}
}
Right click on the "Index" action method in "HomeController" and select "Add View" from the context menu. Set
View Name = Index
View Engine = Razor
and click "Add".
Copy and paste the following code in "Index.cshtml"
@model MVCDemo.Models.CitiesViewModel
@{
ViewBag.Title = "Index";
}
<div style="font-family:Arial">
<h2>Index</h2>
@using (Html.BeginForm())
{
@Html.ListBoxFor(m => m.SelectedCities, Model.Cities, new { size = 4 })
<br />
<input type="submit" value="Submit" />
}
</div>
Note: To select multiple items from the listbox, hold down the CTRL Key.
Part 36 - Difference between Html.TextBox and Html.TextBoxFor
Part 37 - Generating a radiobuttonlist control in mvc using HTML helpers
Part 38 - CheckBoxList in asp.net mvc
In this video we will discuss implementing ListBox in asp.net mvc. We will be using table "tblCity" for this demo.
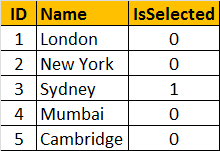
Please refer to Part 38, if you need the sql script to create and populate this table. Please watch Part 38, before proceeding.
The generated listbox should be as shown below. Notice that for each city in table "tblCity", there is an entry in the listbox.
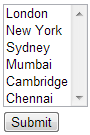
For this demo, we will be using the "City" entity that we generated using ADO.NET entity framework, in Part 38.
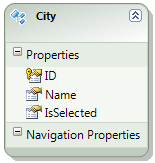
The first step is to create a ViewModel class. In asp.net mvc, view model's are used as techniques to shuttle data between the controller and the view. Right click on the "Models" folder, and add a class file with name=CitiesViewModel.cs. Copy and paste the following code. This class is going to be the Model for the view.
public class CitiesViewModel
{
public IEnumerable<string> SelectedCities { get; set; }
public IEnumerable<SelectListItem> Cities { get; set; }
}
Right click on the "Controllers" folder, and add a "HomeController". Include the following 2 namespaces in "HomeController"
using MVCDemo.Models;
using System.Text;
Copy and paste the following code.
[HttpGet]
public ActionResult Index()
{
SampleDBContext db = new SampleDBContext();
List<SelectListItem> listSelectListItems = new List<SelectListItem>();
foreach (City city in db.Cities)
{
SelectListItem selectList = new SelectListItem()
{
Text = city.Name,
Value = city.ID.ToString(),
Selected = city.IsSelected
};
listSelectListItems.Add(selectList);
}
CitiesViewModel citiesViewModel = new CitiesViewModel()
{
Cities = listSelectListItems
};
return View(citiesViewModel);
}
[HttpPost]
public string Index(IEnumerable<string> selectedCities)
{
if (selectedCities == null)
{
return "No cities selected";
}
else
{
StringBuilder sb = new StringBuilder();
sb.Append("You selected - " + string.Join(",", selectedCities));
return sb.ToString();
}
}
Right click on the "Index" action method in "HomeController" and select "Add View" from the context menu. Set
View Name = Index
View Engine = Razor
and click "Add".
Copy and paste the following code in "Index.cshtml"
@model MVCDemo.Models.CitiesViewModel
@{
ViewBag.Title = "Index";
}
<div style="font-family:Arial">
<h2>Index</h2>
@using (Html.BeginForm())
{
@Html.ListBoxFor(m => m.SelectedCities, Model.Cities, new { size = 4 })
<br />
<input type="submit" value="Submit" />
}
</div>
Note: To select multiple items from the listbox, hold down the CTRL Key.
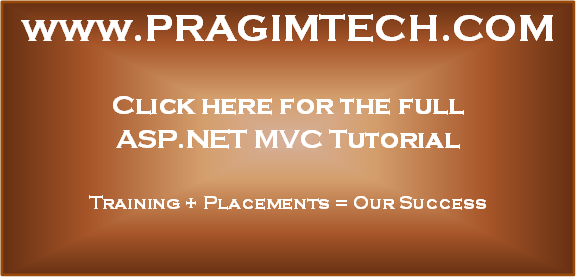
Your videos are very awesome and all the concepts are explained so nicely. Your tutorials make studying very interesting. Thank you so much.
ReplyDeleteHI, I want my ListBox to select only one value. I want to restrict it to multiple selection
ReplyDeleteThen go for DropDownList
DeleteHi
ReplyDeleteThese videos are great, i just wanted to know that in case of radio button and List box control when we click on submit button how mvc will know which all value will be posted back to server.. coz here in List box in controller action you are recieving Ienumerable of selected cities which are seems to be little confusing ..how mvc controller action [HTTPPOST] will recieve the selected values....
hi iam beginner in asp .net mvc.This is very understandable tutorial. so how can i insert the multiple selected value in the database. please teach me with complete tutorial. thank you in advance
ReplyDeletei want to display name instead of the id
ReplyDeletehello sir i want to display name instead of id on this video also how can i pass model to controller in .cshtml
ReplyDeletesir got the error stating = Cannot implicitly convert type 'System.Collections.Generic.IEnumerable' to 'bool'
ReplyDeletepleasee help me what should i do..