Suggested Videos
Part 6 - ViewData and ViewBag in mvc
Part 7 - Models in MVC
Part 8 - Data access in MVC using entity framework
In this video we will discuss, generating hyperlinks using actionlink html helper, for navigation between MVC pages.
Please watch Part 8, before proceeding.
We want to display all the employees in a bulletted list as shown below. Notice that all the employee names are rendered as hyperlinks.
When the hyperlink is clicked, the user will be redirected to employee details page, displaying the full details of the employee as shown below.
Copy and paste the following Index() action method in EmployeeController class. This method retrieves the list of employees, which is then passed on to the view for rendering.
public ActionResult Index()
{
EmployeeContext employeeContext = new EmployeeContext();
List<Employee> employees = employeeContext.Employees.ToList();
return View(employees);
}
At the moment, we don't have a view that can display the list of employees. To add the view
1. Right click on the Index() action method
2. Set
View name = Index
View engine = Razor
Select, Create a stronlgy-typed view checkbox
Select "Employee" from "Model class" dropdownlist
3. Click Add
At this point, "Index.cshtml" view should be generated. Copy and paste the following code in "Index.cshtml".
@model IEnumerable<MVCDemo.Models.Employee>
@using MVCDemo.Models;
<div style="font-family:Arial">
@{
ViewBag.Title = "Employee List";
}
<h2>Employee List</h2>
<ul>
@foreach (Employee employee in @Model)
{
<li>@Html.ActionLink(employee.Name, "Details", new { id = employee.EmployeeId })</li>
}
</ul>
</div>
Points to Remember:
1. @model is set to IEnumerable<MVCDemo.Models.Employee>
2. We are using Html.ActionLink html helper to generate links
Copy and paste the following code in Details.cshtml
@Html.ActionLink("Back to List", "Index")
Part 6 - ViewData and ViewBag in mvc
Part 7 - Models in MVC
Part 8 - Data access in MVC using entity framework
In this video we will discuss, generating hyperlinks using actionlink html helper, for navigation between MVC pages.
Please watch Part 8, before proceeding.
We want to display all the employees in a bulletted list as shown below. Notice that all the employee names are rendered as hyperlinks.

When the hyperlink is clicked, the user will be redirected to employee details page, displaying the full details of the employee as shown below.
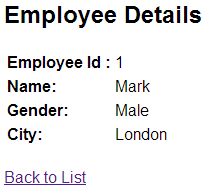
Copy and paste the following Index() action method in EmployeeController class. This method retrieves the list of employees, which is then passed on to the view for rendering.
public ActionResult Index()
{
EmployeeContext employeeContext = new EmployeeContext();
List<Employee> employees = employeeContext.Employees.ToList();
return View(employees);
}
At the moment, we don't have a view that can display the list of employees. To add the view
1. Right click on the Index() action method
2. Set
View name = Index
View engine = Razor
Select, Create a stronlgy-typed view checkbox
Select "Employee" from "Model class" dropdownlist
3. Click Add
At this point, "Index.cshtml" view should be generated. Copy and paste the following code in "Index.cshtml".
@model IEnumerable<MVCDemo.Models.Employee>
@using MVCDemo.Models;
<div style="font-family:Arial">
@{
ViewBag.Title = "Employee List";
}
<h2>Employee List</h2>
<ul>
@foreach (Employee employee in @Model)
{
<li>@Html.ActionLink(employee.Name, "Details", new { id = employee.EmployeeId })</li>
}
</ul>
</div>
Points to Remember:
1. @model is set to IEnumerable<MVCDemo.Models.Employee>
2. We are using Html.ActionLink html helper to generate links
Copy and paste the following code in Details.cshtml
@Html.ActionLink("Back to List", "Index")
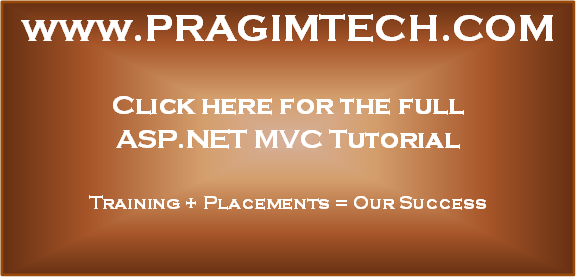
hello Venkat, Hope you are doing good. I have a question in this session.
ReplyDeleteHow can we achieve "@model IEnumerable" in MVC2 using aspx page.
Hello Venkat What is there in Employee Context form. Without using Database Can do this tutor with hard coded data
ReplyDeleteCan you please explain about 'EmployeeContext ' ?
ReplyDeleteEmployeeContext class derives from DbContext class, and is responsible for establishing a connection to the database
DeleteAnita Kane this ur ans
ReplyDeletepublic ActionResult Employee()
{
Employee emp = new Employee();
emp.EmpID = "0120";
emp.EmpBank = "State Bank of India";
emp.EmpCity = "New Delhi";
emp.EmpName = "ABCD XYZ";
emp.EmpPhoneNo = "9999999999";
return View(emp);
}
Sir, can you tell me how to get list of employee using "groupby city" for same example.
ReplyDeleteSir,Without using entity framework,can we inherit DB Context Class in my class.. Please explain me about DB Context Class and Db Sets??
ReplyDeleteDear sir,
ReplyDeleteMany thanks for your videos. It is quite useful. I am not a programmer - but these videos help me to shift my career to programmer. While following this video I am getting cannot implicitly convert type 'System.collection.Generic.List' to System.Web.MVC.ActionResult. It would be helpful if you can give your inputs - if possible. I using the same entity objects as you are doing.
Cheers
Sathya
Sir why you have used entity framework instead of ado.net
ReplyDeleteBecause it's newer than ADO plus you dont have to write a lot of code in worked with EF and Linq.
DeleteSir, thanks for such wonderful video.
ReplyDeleteI can please explain the difference between @Html.ActionLink() and @Html.RouteLink(); And which scinario use one over the another?
How the routevalue used in RouteLink
ReplyDeleteWhatever fields/properties are there in routeValue object, MVC will try to map it with the action paramters your link will invoke. So here, Details(int id) action expects id as input, so we will pass it in routeValue object. More values can be passed as well but their names has to be same as that of parameters and MVC will do the rest.
DeleteSir, when and why do we use IEnumerable ?
ReplyDeleteWhen you deal with collections you may have to use IEnumerable to loop through the collection. When you use IEnumerable it will handle all the enumerable types such as list and arrays. On the other hand you can use that particular type if you want. Something like this List
Delete