Suggested Videos
Part 5 - Views in an mvc application
Part 6 - ViewData and ViewBag in mvc
Part 7 - Models in MVC
The controller responds to URL request, gets data from a model and hands it over to the view. The view then renders the data. Model can be entities or business objects.
In part 7, we have built Employee entity.
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
In this video, we will discuss, retrieving data from a database table tblEmployee using entity framework. In a later video, we will discuss using business objects as our model.
Step 1: Install entity framework, if you don't have it installed already on your computer. At the time of this recording the latest version is 5.0.0.0. Using nuget package manager, is the easiest way to install. A reference to EntityFramework.dll is automatically added.
Open visual studio > Tools > Library Package Manager > Manage NuGet Packages for Solution
Step 2: Add EmployeeContext.cs class file to the Models folder. Add the following "using" declaration.
using System.Data.Entity;
Copy & paste the following code in EmployeeContext.cs
public class EmployeeContext : DbContext
{
public DbSet<Employee> Employees {get; set;}
}
EmployeeContext class derives from DbContext class, and is responsible for establishing a connection to the database. So the next step, is to include connection string in web.config file.
Step 3: Add a connection string, to the web.config file, in the root directory.
<connectionStrings>
<add name="EmployeeContext"
connectionString="server=.; database=Sample; integrated security=SSPI"
providerName="System.Data.SqlClient"/>
</connectionStrings>
Step 4: Map "Employee" model class to the database table, tblEmployee using "Table" attribute as shown below.
[Table("tblEmployee")]
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
Note: "Table" attribute is present in "System.ComponentModel.DataAnnotations.Schema" namespace.
Step 5: Make the changes to "Details()" action method in "EmployeeController" as shown below.
public ActionResult Details(int id)
{
EmployeeContext employeeContext = new EmployeeContext();
Employee employee = employeeContext.Employees.Single(x => x.EmployeeId == id);
return View(employee);
}
Step 6: Finally, copy and paste the following code in Application_Start() function, in Global.asax file. Database class is present "in System.Data.Entity" namespace. Existing databases do not need, database initializer so it can be turned off.
Database.SetInitializer<MVCDemo.Models.EmployeeContext>(null);
That's it, run the application and navigate to the following URL's and notice that the relevant employee details are displayed as expected
http://localhost/MVCDemo/Employee/details/1
http://localhost/MVCDemo/Employee/details/2
Part 5 - Views in an mvc application
Part 6 - ViewData and ViewBag in mvc
Part 7 - Models in MVC
The controller responds to URL request, gets data from a model and hands it over to the view. The view then renders the data. Model can be entities or business objects.
In part 7, we have built Employee entity.
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
In this video, we will discuss, retrieving data from a database table tblEmployee using entity framework. In a later video, we will discuss using business objects as our model.
Step 1: Install entity framework, if you don't have it installed already on your computer. At the time of this recording the latest version is 5.0.0.0. Using nuget package manager, is the easiest way to install. A reference to EntityFramework.dll is automatically added.
Open visual studio > Tools > Library Package Manager > Manage NuGet Packages for Solution
Step 2: Add EmployeeContext.cs class file to the Models folder. Add the following "using" declaration.
using System.Data.Entity;
Copy & paste the following code in EmployeeContext.cs
public class EmployeeContext : DbContext
{
public DbSet<Employee> Employees {get; set;}
}
EmployeeContext class derives from DbContext class, and is responsible for establishing a connection to the database. So the next step, is to include connection string in web.config file.
Step 3: Add a connection string, to the web.config file, in the root directory.
<connectionStrings>
<add name="EmployeeContext"
connectionString="server=.; database=Sample; integrated security=SSPI"
providerName="System.Data.SqlClient"/>
</connectionStrings>
Step 4: Map "Employee" model class to the database table, tblEmployee using "Table" attribute as shown below.
[Table("tblEmployee")]
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
Note: "Table" attribute is present in "System.ComponentModel.DataAnnotations.Schema" namespace.
Step 5: Make the changes to "Details()" action method in "EmployeeController" as shown below.
public ActionResult Details(int id)
{
EmployeeContext employeeContext = new EmployeeContext();
Employee employee = employeeContext.Employees.Single(x => x.EmployeeId == id);
return View(employee);
}
Step 6: Finally, copy and paste the following code in Application_Start() function, in Global.asax file. Database class is present "in System.Data.Entity" namespace. Existing databases do not need, database initializer so it can be turned off.
Database.SetInitializer<MVCDemo.Models.EmployeeContext>(null);
That's it, run the application and navigate to the following URL's and notice that the relevant employee details are displayed as expected
http://localhost/MVCDemo/Employee/details/1
http://localhost/MVCDemo/Employee/details/2
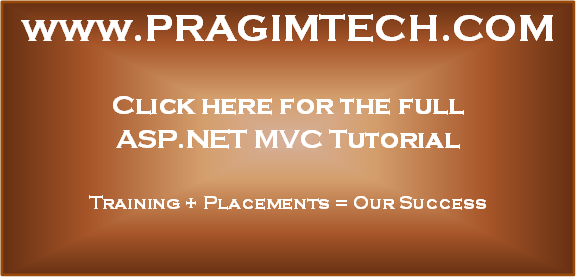
Sir,
ReplyDeleteI created the Employee Class in the following way :
[Table("tblEmployee")]
public class Employee
{
[Key]
public int EmpId { get; set; }
public byte EmpDeptId { get; set; }
public string EmpName { get; set; }
public DateTime EmpJoinDate { get; set; }
public char EmpGender { get; set; }
public string EmpCity { get; set; }
}
And Kept the EmpGender field in the Database Table as {nchar(1)}.
In this way I could not show the EmpGender in the view. But when I changed it from char to string it worked fine.
How can I solve it if I want to put Gender as character as I like to insert only 'M' or 'F'?
Thanks
u have to used checked attribute to true by using the value of EmpGender.
DeleteEx. input type="radio" name=
"empGender" checked='@Modal.EmpGender=='m'? 'true' : false '
Sir i created application as u discribe but i get Login fail Application pool error
ReplyDeleteHello Sir
Here i want to add two points
1- Use "Key" in the model class to make query on class's property
for example here we make query on Id bases so we have to use it with Id property
[Table("Employee")]
public class Employee
{
[Key]
public int EmpId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
2- First time when i run it then i get a log-in fail connection pooling error
so
- move in iis Manager.
- Open Application pool at left side of screen click it.
- then there is a pooling connection is defaultAppPool, select it.
- Click Advance setting link at right side menu bar.
- Change Identity property from ApplicationPoolIdentity to LocalSystem.
now run your application.
Note - 2nd option is applicable if you are using local server.
Great tutorial.
ReplyDeleteWhat are the changes we do for the above same with MySQL
Hi Venkat please can you help me in solving this as am following your video but am facing this issue...
ReplyDeleteOne or more validation errors were detected during model generation:
\tSystem.Data.Entity.Edm.EdmEntityType: : EntityType 'empDetails' has no key defined. Define the key for this EntityType.
\tSystem.Data.Entity.Edm.EdmEntitySet: EntityType: EntitySet 'Employees' is based on type 'empDetails' that has no keys defined.
Can you make sure to set primary key on the table.
Deleteused [key]
Deleteabove your primary key and imports appropriate namespace
I have same issue
DeleteServer Error in '/' Application.
One or more validation errors were detected during model generation:
learn_MVC.Models.Details: : EntityType 'Details' has no key defined. Define the key for this EntityType.
Detailss: EntityType: EntitySet 'Detailss' is based on type 'Details' that has no keys defined.
Description: An unhandled exception occurred during the execution of the current web request. Please review the stack trace for more information about the error and where it originated in the code.
Exception Details: System.Data.Entity.ModelConfiguration.ModelValidationException: One or more validation errors were detected during model generation:
learn_MVC.Models.Details: : EntityType 'Details' has no key defined. Define the key for this EntityType.
Detailss: EntityType: EntitySet 'Detailss' is based on type 'Details' that has no keys defined.
kindly someone help me
Add attribute on [Key] top of Primary column defined for class
Delete[Table("tblEmployee")]
public class Employee
{
[Key]
public int EmployeeId { get; set; }
Hello Venkat thanks for the great tutorial, I followed all the steps in this lesson but I changed the parameter name instead of id to E_Id
ReplyDeleteit didn't work and gave me error message "The parameters dictionary contains a null entry for parameter 'id' of non-nullable type" when Ichanged the parameter name back to id it worked. I did all my best in troubleshooting this issue and finally found that there is a relation between this parameter name and the parameter name in the RouteConfig.cs file both parameter names have to have the same name. is it make any sence? is there any work arround?
Hello Mr. Venkat
ReplyDeleteI received this error when I run program "An error occurred while getting provider information from the database. This can be caused by Entity Framework using an incorrect connection string. Check the inner exceptions for details and ensure that the connection string is correct."
Can you explain where the problem is ?
hi sir ,even am getting same error asOne or more validation errors were detected during model generation:
ReplyDelete\tSystem.Data.Entity.Edm.EdmEntityType: : EntityType 'Employee' has no key defined. Define the key for this EntityType.
\tSystem.Data.Entity.Edm.EdmEntitySet: EntityType: EntitySet 'Employees' is based on type 'Employee' that has no keys defined.
how to fix it
Please help me
ReplyDeleteServer Error in '/MvcDemo_ModelExample1' Application.
Sequence contains no elements
Description: An unhandled exception occurred during the execution of the current web request. Please review the stack trace for more information about the error and where it originated in the code.
Exception Details: System.InvalidOperationException: Sequence contains no elements
Source Error:
Line 17:
Line 18: EmployeeContext employeeContext = new EmployeeContext();
Line 19: Employee employee = employeeContext.Employees.Single(x => x.EmployeeId == id);
Line 20:
Line 21:
Source File: c:\Users\raihan\Documents\Visual Studio 2012\Projects\MvcDemo2\MvcDemo_ModelExample1\Controllers\EmployeeController.cs Line: 19
Hi Mr. Venkat,
ReplyDeleteI received this error when I run program "An error occurred while getting provider information from the database. This can be caused by Entity Framework using an incorrect connection string. Check the inner exceptions for details and ensure that the connection string is correct."
Could you please provide solution to the above error?
i follow all the step but vs-10 giving the following warning i search a lot but fail.
ReplyDelete"Warning 1 The element 'entityFramework' has invalid child element 'providers'. List of possible elements expected: 'contexts'. C:\Users\Waleed\documents\visual studio 2010\Projects\MVC4web\MVC4web\Web.config 49 6 MVC4web
"
in the web.config root directory file
tag is under line for warning
kindly help me as soon as possible i am waiting for you positive reply..
Entity Framework Problem, now update version is 6.0.1 and it installed by Nuget manager bt this framework did not work correctly Give the Following Error
ReplyDeleteAn exception of type 'System.Data.Entity.Core.ProviderIncompatibleException' occurred in EntityFramework.dll but was not handled in user code
Additional information: An error occurred while getting provider information from the An exception of type 'System.Data.Entity.Core.ProviderIncompatibleException' occurred in EntityFramework.dll but was not handled in user code
Additional information: An error occurred while getting provider information from the database. This can be caused by Entity Framework using an incorrect connection string. Check the inner exceptions for details and ensure that the connection string is correct.
Hi Guys people who are not able to move ahead with the database connection.
ReplyDeletePlease note that when you install Entity Framework now from Nuget. It Downloads the Newest version which is EF 6.0.2. You need to do additional configuration changes to make it work, So i would prefer to suggest install from Nuget by Nuget Package manager condole and download EF 5.0 which is supported by this tuotrial.
Steps:Go to Tools -> Library Package Manager->Package Manager Console and Type PM> Install-Package EntityFramework -Version 5.0.0
Hope this helps you all :)
This Works
DeleteFollowing error shows when i tried this method
DeleteInstall-Package : Already referencing a newer version of 'EntityFramework'.
At line:1 char:1
+ Install-Package EntityFramework -Version 5.0.0
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : NotSpecified: (:) [Install-Package], InvalidOperationException
+ FullyQualifiedErrorId :
i got a same when run my code ,and after set primary key the error is same
ReplyDeleteOne or more validation errors were detected during model generation:
myfirstmvc.Models.employee: : EntityType 'employee' has no key defined. Define the key for this EntityType.
Employees: EntityType: EntitySet 'Employees' is based on type 'employee' that has no keys defined.
Employee employee = employeeContext.Employee.Single(x => x.EmployeeID == id);
ReplyDeleteAn exception of type 'System.Data.Entity.Core.ProviderIncompatibleException' occurred in EntityFramework.dll but was not handled in user code
Additional information: An error occurred while getting provider information from the database. This can be caused by Entity Framework using an incorrect connection string. Check the inner exceptions for details and ensure that the connection string is correct.
great videos except I am lost as to where he explains/creates the databases. I am now on part 10 after figuring out another way of creating a database table. But i would prefer some information on how he got the database. Kind of makes the videos more difficult when he introduces data that was never created in the video series covering MVC
ReplyDeleteHi Venkat .
ReplyDeleteThis is Venkat Krishnan .I am a junior Software Engineer(Trainee).I got this job by the help of your videos .Thanks for the wonderful Training.I have to develop the MVC application using MySql database . I have tried many ways to achieve that bt its still not connected with the database . Can you give me some Ideas to develop MVC using MySql ...
use this code it is working
ReplyDelete[Table("tblemp")]
public class Employee
{
[Key]
public int Employee_id { get; set; }
public string Name { get; set; }
public string City { get; set; }
public string Gender { get; set; }
}
Hi! I'm have an error: "An error occurred while getting provider information from the database. This can be caused by Entity Framework using an incorrect connection string. Check the inner exceptions for details and ensure that the connection string is correct."
in the line: Employee employee = employeeContext.Employees.Single(x => x.EmployeeID == id);
Can someone help me? Maybe someone have the same problem and know how to solve? I'm will be very grateful.
hiii venkat sir how ru???sir plzzzzzzzzzzzzzzzzzz explain the concepts abt entity framework 5.0(code first,model first,DB first) ,n-tire architecture and LINQ....actually u have used entity framework and LINQ concepts in MVC tutorial and u told tat u will explain this concepts long back but u didnt..sir plzzzzzz upload these video tutorials sir as soon as possible :)
ReplyDeletehi,this is a good tutorial but i am getting this error,,please help..
ReplyDelete"The parameters dictionary contains a null entry for parameter 'idd' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Details(Int32)' in 'Mvcsecond.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
Parameter name: parameters "
run your program as below format \\http://localhost/MvcEntityFramework/Employee/Details/1
DeleteHi everyone,
ReplyDeleteim getting this error
"The underlying provider failed on Open"
please help me
Hi Venkat,
ReplyDeleteI am following ur videos these days very oftenly. I've installed EF 6.0 from Nuget packages from Solution. With this i got EF 6.0 later i uninstalled and installed EF 5.0 as you explained in this video. I am getting the following error "Configuration Error". I removed the "" line of code from my root web.config file since i do have the sql provider in the next line. with this change, am getting the error "Login failed for user 'IIS APPPOOL\DefaultAppPool'". Its not able to connect to DB due to this configuration settings. Pls help me to resolve the issue. thanks in advance.
hi ,
ReplyDelete"The parameters dictionary contains a null entry for parameter 'EmployeeId' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Details(Int32)' in 'Mvcsecond.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
Parameter name: parameters "
though i entered url: http://localhost:49979/Employee/Details/1
how can i resolve this error
You have not added DbContext..
Deletepublic class EmployeeContext:DbContext
{
public DbSet Employees { get; set; }
}
if you get application pool error then go to your IIS server and then go to application pools go to your Defaultapppool there you can see identity right click on it and go to advanced settings change the identity name to your PC name and give your windows credentials(login and password) manually this will fix the error
ReplyDeleteHey Venkat, Can you please tell me if i am having 10 classes then how do i map my class to connection string as u mention the name of the class should match with the class name.
ReplyDeleteInvalid object name 'dbo.Employees'. when In my db table name is Employee
ReplyDeletemake sure the connection string name is :EmployeeContext" in web.config
ReplyDeleteI was facing same issue and resolve with this solution - downgrade the Entity Framework 6 of the created project to EF5 (Because ASP.NET MVC 4 scaffolding does not support Entity Framework 6 or later
ReplyDeletenuget package console command for EF5
Uninstall-package EntityFramework
Install-Package EntityFramework version 5.0.0
Happy Coding :)
hi sir
ReplyDeletei tried ur example but i got the below exception plz give some clarity on below exception
Exception Details: System.Data.SqlClient.SqlException: Cannot open database "Sample" requested by the login. The login failed.
Login failed for user 'NT AUTHORITY\SYSTEM'.
In MS SQL Server Management studio go to (in left panel)Security -> login -> NT AUTHORITY\SYSTEM.
Deleteclick properties -> server roles and check sysadmin.
It will work
Use SQl server Authentication instead of Windows Authentication
DeleteDoesn't work in VS 2012. Also this is a bad practice. You should use something like this:
ReplyDeleteusing(DatabaseEntities _entities = new DatabaseEntities())
{
//GetDetails code go here
}
I am Using Employees Table of Northwind Database and only using the fields given below not all :
ReplyDelete[Table("Employees")]
public class Employee
{
public int EmployeeID { get; set; }
public string LastName { get; set; }
public string city { get; set; }
public string country { get; set; }
}
while other things are the same as the video describes.
but I am getting this Exception on the line :
Employee employee = employeeContext.Employees.Single(x => x.EmployeeID == id);
Error Message : The underlying provider failed on Open.
Need guidance ........! what to do ?
Many people have troubles by codeline:
ReplyDeleteEmployee employee = employeeContext.Employees.Single(x => x.EmployeeId == id);
I get this message:
{Function evaluation disabled because a previous function evaluation timed out. You must continue execution to reenable function evaluation.}
I had copied the code exactly and followed the video step by step too.
If some people have a working version, can they share it with us?
I create the database by this script:
Create table tblEmployee
(
EmployeeId int primary key identity,
Name nvarchar(50),
Gender nvarchar(50),
City nvarchar(50)
)
GO
Insert into tblEmployee values ('Mark', 'Male', 'London')
Insert into tblEmployee values ('John', 'Male', 'Chennai')
Insert into tblEmployee values ('Mary', 'Female', 'New York')
Insert into tblEmployee values ('Mike', 'Male', 'Sydney')
GO
Select * from tblEmployee
Thanks a lot harry for the database script, it saved some time.
DeleteI saw at the Microsoft website that MVC4 will not support EF 6
ReplyDeleteUser DeepCoding placed a message at this website and I found the link from Microsoft website.
http://support2.microsoft.com/kb/2816241
Hello sir,
ReplyDeletewhile try this i am getting this error .
The ADO.NET provider with invariant name 'Sql.Data.SqlClient,EntityFramework.SqlServer' is either not registered in the machine or application config file, or could not be loaded. See the inner exception for details.
Hello Venkat... Many of us having provider related error.
ReplyDeleteCould you please provide some information related to provider information in connection string.Getting error as:
An error occurred while getting provider information from the database. This can be caused by Entity Framework using an incorrect connection string. Check the inner exceptions for details and ensure that the connection string is correct.
in the connection string server parameter please specify the database server name correctly. In my case it was "SQLEXPRESS2014" so i gave it as server=.\SQLEXPRESS2014; and the error was gone. Hope this will help.
DeletePlease anyone write the connection strings for non windows authentication
ReplyDeleteThe parameters dictionary contains a null entry for parameter 'employeeid' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Details(Int32)' in 'Employee.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
ReplyDeleteParameter name: parameters.
When i ran this program am getting this error. Please let me know what is the mistake.
public class EmployeeController : Controller
{
public ActionResult Details(int employeeid)
{
EmployeeContext empcontext = new EmployeeContext();
Employee.Models.Employee employee = empcontext.Employees.Single(emp => emp.empid == employeeid );
return View(employee);
}
[Table("employee")]
public class Employee
{
public int empid { get; set; }
public string Name { get; set; }
public string Location { get; set; }
}
public class EmployeeContext:DbContext
{
public DbSet Employees { get; set; }
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Conventions.Remove();
}
}
This is because in the RouteConfig id is mentioned as the parameter and you are passing employeeid as parameter within the controller action method.Change it id might resolve the issue. Please check.
DeleteThis is part of my connection string:
ReplyDeleteconnectionString="server=XXXX-PC\SQLEXPRESS; database=SAMPLE; integrated security=SSPI"
the sample from the video didn't work for me as it installed EF 6 from MS.
I did it again using MVC 5 with VS2014 and Entity Framework 6
seems to be working.
I have this error ?
ReplyDeleteIllegal characters in path.
Description: An unhandled exception occurred during the execution of the current web request. Please review the stack trace for more information about the error and where it originated in the code.
Exception Details: System.ArgumentException: Illegal characters in path.
Source Error:
Line 23: //};
Line 24: EmployeeContext employeeContext = new EmployeeContext();
Line 25: Employee employee = employeeContext.Employees.Single(emp => emp.EmployeeId == id);
Line 26:
Line 27: return View(employee);
What is Wrong????
Error 3 The type 'mvcdemo1.Models.EmployeeContext' cannot be used as type parameter 'TContext' in the generic type or method 'System.Data.Entity.Database.SetInitializer(System.Data.Entity.IDatabaseInitializer)'. There is no implicit reference conversion from 'mvcdemo1.Models.EmployeeContext' to 'System.Data.Entity.DbContext'. C:\Users\Trust\Documents\Visual Studio 2012\Projects\mvcdemo1\mvcdemo1\Global.asax.cs 23 13 mvcdemo1
ReplyDeletei got this error please sort it out...
EmployeeContext should be inherited by DbContext.
DeleteI believe u have already figured it out.
Error 1 The type 'MVCDemo1.Models.EmployeeContext' cannot be used as type parameter 'TContext' in the generic type or method 'System.Data.Entity.Database.SetInitializer
ReplyDeletei got this Error msg
(System.Data.Entity.IDatabaseInitializer)'. There is no implicit reference conversion from 'MVCDemo1.Models.EmployeeContext' to 'System.Data.Entity.DbContext'. C:\Users\VirtualUser\documents\visual studio 2013\Projects\MVCDemo1\MVCDemo1\Global.asax.cs 19 13 MVCDemo1
The parameters dictionary contains a null entry for parameter 'id' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Details(Int32)' in 'MvcDemo.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
ReplyDeleteParameter name: parameters
To resolve most of the issues please do below changes(if you are working on MVC4.0 application)
ReplyDeleteSteps 1 :Go to Tools -> Library Package Manager->Package Manager Console and Type PM> Install-Package EntityFramework -Version 5.0.0(Tutorial video is supportable EF 5.0.0)
Steps 2 :Run below query on your database table:
grant select on dbo.tblname to public
Step 3: after updating EF if you are getting providers error .please comment below line from web.config file.
The parameters dictionary contains a null entry for parameter 'deptId' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Index(Int32)' in 'MVCdemo.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
ReplyDeleteParameter name: parameters
" Sir , when i am working with multiple tables, it gives me the above mentioned error.... sir, please find a solution for this.. thanks"
Can we use simple sql queries rather than linq queries in MVC
ReplyDeleteHi venkat when i click edit i got this error can you solve this
ReplyDeleteThe parameters dictionary contains a null entry for parameter 'id' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Edit(Int32)' in 'Mvcdemo1.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
Parameter name: parameters
i have been getting this error
ReplyDeleteSystem.Data.SqlClient.SqlException: Invalid object name 'dbo.Employees'.
in the line
Employee employee = employeeContext.Employees.Single(emp => emp.EmployeeId == id);
can someone please help me to understand what is really going went through most of the comments and found many having the trouble in the same line
i have been getting this error
ReplyDeleteSystem.Data.SqlClient.SqlException: Invalid object name 'dbo.Employees'.
in the line
Employee employee = employeeContext.Employees.Single(emp => emp.EmployeeId == id);
can someone please help me to understand what is really going went through most of the comments and found many having the trouble in the same line
Employee employee = employeeContext.Employees.Single(emp => emp.EmployeeId == id);
ReplyDeletethe code above is being highlighted and giving the error msg as below can someone please help me so that i can get a move on
System.Data.SqlClient.SqlException: Invalid object name 'dbo.Employees'.
I won't mind helping you if you, if you haven't been helped as yet of course, and if you don't mind sending me the code in your EmployeeContext class and in your Employee.cs class and one last thing the name of the table that you're referencing in your database. :)
DeleteI run into the same problem. My setup is the same as the video, excep the table name was Employee instead of tblEmployee. After it failed, I renamed the table to Employees and it worked. Now I am confused was there anerror in the instructions or something else caused DbContext to work differently as explained?
ReplyDeletewhen i run code on this line
ReplyDeleteEmployee employee = employeeContext.Employees.Single(x => x.EmployeeId == id);
this error shown "sequence contains no elements".
Please help me i am trying to learn all the tutorials but suck on this point.
Always getting error - Could not load file or assembly 'EntityFramework, Version=4.4.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089' or one of its dependencies. The located assembly's manifest definition does not match the assembly reference. (Exception from HRESULT: 0x80131040)
ReplyDeleteEven after updating the EF to 6.1.3
Invalid value for key 'attachdbfilename'. Error Comes. What should i do ?
ReplyDeletewhen i am trying to execute the code the error shows on line...
ReplyDeleteEmployee employee = employeeContext.Employees.Single(x => x.EmployeeId == id);..The underlying provider failed on Open.
please Help
Hi I am getting this Error
ReplyDeleteThe parameters dictionary contains a null entry for parameter 'id' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Details(Int32)' in 'Views.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
Parameter name: parameters
when i am trying to execute the code the error shows on line...
ReplyDeleteEmployee employee = employeeContext.Employees.Single(x => x.EmployeeId == id);..The underlying provider failed on Open.
please Help
"The system cannot find the file specified" error is coming while running my application,shows error at that line "Employee employee = employeecontext.Employees.Single(emp => emp.Employeeid == id);". DOnt kow what to do.
ReplyDeleteCan we use Access Database instead of SQL server database?
ReplyDeleteLine 27: //};
ReplyDeleteLine 28: EmployeeContext EmployeeContext = new EmployeeContext();
Line 29: Employee emp = EmployeeContext.Employees.Single(x => x.EmpId == id);//here its throwing an exception
Line 30: return View("employee", emp);
Line 31: //Employee employee = EmployeeContext.Employee.Single(emp => emp.empId == id);
Exception Details: System.Data.Entity.ModelConfiguration.ModelValidationException: One or more validation errors were detected during model generation:
MvcApplication1.Models.Employee: : EntityType 'Employee' has no key defined. Define the key for this EntityType.
Employees: EntityType: EntitySet 'Employees' is based on type 'Employee' that has no keys defined.
an exception of type 'system.data.entity.core.entityexception' occurred in entityframework.sqlserver.dll but was not handled in user code additional information: the underlying provider failed on open.
ReplyDeletepls help me sir
Additional information: The underlying provider failed on Open.
ReplyDeleteplz help ..
public ActionResult Details(string EmployeeCode)
ReplyDelete{
EmployeeContext employeecontext = new EmployeeContext();
Employee employee = employeecontext.Employees.Single(emp => emp.Employee_Code == EmployeeCode);
return View(employee);
//Employee employee = new Employee()
//{
// Employee_Code = "00620",
// //First_Name = "Alagu",
// Employee_Name = "Alagu Ganesh A",
// DOJ = "Feb 26, 2009",
// //Confirm_Date = "Feb 26, 2009",
// Actl_Confirm_Date = "Jul 01, 2011",
// Designation = "Team Lead - ERP Development",
// Department = "Corporate - Support",
// //Cost_Center = "Corporate - Support",
// //Grade_Code = "B6C2",
// Location = "CHENNAI",
// State_Name = "Tamil Nadu",
// Supervisor_Code = "00986",
// Supervisor_Name = "Suresh Gopal"
//};
//return View(employee);
}
Dear Venkat,
I'm Alagu Ganesh and I'm using your code but the input is not int its is string you can see that in this email.
I'm compile this and I'm getting this error.
"Sequence contains no elements"
Line 17: Employee employee = employeecontext.Employees.Single(emp => emp.Employee_Code == EmployeeCode);
please check and help me to fix this error.
your blog and your you-tube videos are very help full and I'm learning lot of new software language.
Thanks & regards,
Alagu Ganesh
when i try to run same code it will give error.what to do to fix this problem mr.venkat?
ReplyDelete"An exception of type 'System.Data.ProviderIncompatibleException' occurred in EntityFramework.dll but was not handled in user code"
Hi Venkat,
ReplyDeletei am facing below error.
An exception of type 'System.Data.Entity.Core.EntityException' occurred in EntityFramework.SqlServer.dll but was not handled in user code
Additional information: The underlying provider failed on Open.
Hi venkat how i can same thing with mysql database 5.6
ReplyDeleteI was facing the problem with "The underlying provider failed on Open" error. I finally figured it out, so I thought to share this info which may help others. It looks like this error is related to security/authentication problem. The connectionString has "integrated security=SSPI" in which it will authenticate using current Windows credentials. If you were using the default ASP.NET to test without IIS, it would work. However, previous tutorial instructed us to using Local IIS, and this caused the authentication issues since IIS is not using your current Windows credentials (but "anonymous", I think). Here is the fix that worked for me, using Northwind database on MS SQL server as example:
ReplyDelete1) Expand the database (Northwind).
2) Expand Security, and then Users
3) Make sure you have assigned a user to this database (e.g. username: user1 and password: pass1)
4) After that, go to your Web.Config file and change the connection to:
Hope it helps,
Some how my post was cut off at connection to:... Here is the connection string with username and password, and it should work updated the connectionString.
Delete"Data Source = yourSQLHostName;Initial Catalog = Northwind; User Id=user1;Password=pass1;"
Thank you sir for your tutorial i followed part 8 but i am getting an error :
ReplyDeleteThe model item passed into the dictionary is of type 'mvcdemo.Models.Employee', but this dictionary requires a model item of type 'System.Collections.Generic.IEnumerable`1[mvcdemo.Models.Employee]'.
i have tried many times but it's not working
Hello,
ReplyDeleteI'm stuck from last two days facing the exception.
I tried various solutions over the net, posted a question on stackoverflow but no luck please help me in this regard.
here is the link of question over stack over flow:
https://stackoverflow.com/questions/48548962/sql-server-browser-service-running-but-still-server-name-not-showing-in-vs2015
And Here is the error i'm facing (not the complete error because not let me write more than 4096 characters):
Message System.Data.Entity.Core.EntityException: The underlying provider failed on Open. ---> System.Data.SqlClient.SqlException: A network-related or instance-specific error occurred while establishing a connection to SQL Server. The server was not found or was not accessible. Verify that the instance name is correct and that SQL Server is configured to allow remote connections. (provider: SQL Network Interfaces, error: 25 - Connection string is not valid) ---> System.ComponentModel.Win32Exception: The parameter is incorrect --- End of inner exception stack trace
Hi I am facing the error:
ReplyDeleteThe parameters dictionary contains a null entry for parameter 'id' of non-nullable type 'System.Int32' for method 'System.Web.Mvc.ActionResult Details(Int32)' in 'MVCDemo1.Controllers.EmployeeController'. An optional parameter must be a reference type, a nullable type, or be declared as an optional parameter.
Parameter name: parameters
in Employee controller for this line of code I get the above error
Employee employee = employeeContext.Employees.Single(x => x.EmpId == id);
Hi venkatSir,
ReplyDeleteI am getting exception handler error at
Employee employee = EmployeeContext.Employees.Single(emp => emp.EmpId == id);
an exception of type 'system.data.entity.core.entityexception' occurred in entityframework.sqlserver.dll but was not handled in user code additional information: the underlying provider failed on open.
I am using visual studio 2012 and SSMS 2012 (My connection string was not created hence i added it explicitly as provided in part-8)
Model Class [Employees.cs]
ReplyDeleteusing System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations.Schema;
namespace WebApplication5.Models
{
[Table("Table")]
public class Employees
{
[key]
public int Id { get; set; }
public string Name { get; set; }
public string Gender { get; set;}
public string City { get; set; }
}
}
-------------
Controller Class [EmployeeController.cs]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using WebApplication5.Models;
namespace WebApplication5.Controllers
{
public class EmployeeController : Controller
{
EmployeeDBEntities objEmployeeDBEntities = new EmployeeDBEntities();
public ActionResult Index()
{
return View();
}
public ActionResult Details(int id)
{
var data = objEmployeeDBEntities.Tables.Where(x => x.Id == id).ToList();
EmployeeContext employeeContext = new EmployeeContext();
ViewBag.data = data[0];
return View(employeeContext);
}
}
}
------------------
Model Class [EmployeeContext.cs ]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace WebApplication5.Models
{
public class EmployeeContext :DbContext
{
public DbSet Employees { get; set; }
}
}
----------------------
Global.asax.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Routing;
using System.Data.Entity;
namespace WebApplication5
{
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
Database.SetInitializer(null);
AreaRegistration.RegisterAllAreas();
RouteConfig.RegisterRoutes(RouteTable.Routes);
}
}
}
-------------------------------------------------------------
Sr, can u plz explain how the selec query works here, i mean how column names are mapped
ReplyDeleteSystem.InvalidOperationException: 'The specified cast from a materialized 'System.String' type to the 'System.Int32' type is not valid.'
ReplyDeletesir i was getting this error plese help me solve it
Any one have problem when running the project with error 'NT AUTHORITY\IUSR' just Modify this line in your Web.config file like this in :
ReplyDeleteSir, Showing Entity Framework Exception in Employee Controller
ReplyDeleteThe underlying provider failed on Open.
please tell me the solution sir
please look the Connection String in web.config
using System;
ReplyDeleteusing System.Collections.Generic;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Web;
namespace DemoTwo.Models
{
[Table(tblEmployee)]
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
}
Severity Code Description Project File Line Suppression State
Error CS0103 The name 'tblEmployee' does not exist in the current context DemoTwo C:\Users\Mahesh\source\repos\DemoTwo\Models\Employee.cs 10 Active