Suggested Videos
Part 11 - Using business objects as model in mvc
Part 12 - Creating a view to insert data
Part 13 - FormCollection in mvc
In this video we will discuss mapping asp.net request data to controller action simple parameter types. Please watch Part 13, before proceeding with this video.
To save form data, to a database table, we used the following code in Part 13. From Part 13, it should be clear that, FormCollection class will automatically receive the posted form values in the controller action method.
[HttpPost]
public ActionResult Create(FormCollection formCollection)
{
Employee employee = new Employee();
// Retrieve form data using form collection
employee.Name = formCollection["Name"];
employee.Gender = formCollection["Gender"];
employee.City = formCollection["City"];
employee.DateOfBirth =
Convert.ToDateTime(formCollection["DateOfBirth"]);
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.AddEmmployee(employee);
return RedirectToAction("Index");
}
The above controller "Create" action method can be re-written using simple types as shown below. Notice that, the create action method has got parameter names that match with the names of the form controls. To see the names of the form controls, right click on the browser and view page source. Model binder in mvc maps the values of these control, to the respective parameters.
[HttpPost]
public ActionResult Create(string name, string gender, string city, DateTime dateOfBirth)
{
Employee employee = new Employee();
employee.Name = name;
employee.Gender = gender;
employee.City = city;
employee.DateOfBirth = dateOfBirth;
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.AddEmmployee(employee);
return RedirectToAction("Index");
}
But do we really to do these mappings manually. The answer is no. In a later video session we will see how to automatically map the request data, without having to do it manually.
Please note that, the order of the parameters does not matter. What matters is the name of the parameter. If the parameter name is different from the form control name, then the form data will not be mapped as expected.
Part 11 - Using business objects as model in mvc
Part 12 - Creating a view to insert data
Part 13 - FormCollection in mvc
In this video we will discuss mapping asp.net request data to controller action simple parameter types. Please watch Part 13, before proceeding with this video.
To save form data, to a database table, we used the following code in Part 13. From Part 13, it should be clear that, FormCollection class will automatically receive the posted form values in the controller action method.
[HttpPost]
public ActionResult Create(FormCollection formCollection)
{
Employee employee = new Employee();
// Retrieve form data using form collection
employee.Name = formCollection["Name"];
employee.Gender = formCollection["Gender"];
employee.City = formCollection["City"];
employee.DateOfBirth =
Convert.ToDateTime(formCollection["DateOfBirth"]);
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.AddEmmployee(employee);
return RedirectToAction("Index");
}
The above controller "Create" action method can be re-written using simple types as shown below. Notice that, the create action method has got parameter names that match with the names of the form controls. To see the names of the form controls, right click on the browser and view page source. Model binder in mvc maps the values of these control, to the respective parameters.
[HttpPost]
public ActionResult Create(string name, string gender, string city, DateTime dateOfBirth)
{
Employee employee = new Employee();
employee.Name = name;
employee.Gender = gender;
employee.City = city;
employee.DateOfBirth = dateOfBirth;
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.AddEmmployee(employee);
return RedirectToAction("Index");
}
But do we really to do these mappings manually. The answer is no. In a later video session we will see how to automatically map the request data, without having to do it manually.
Please note that, the order of the parameters does not matter. What matters is the name of the parameter. If the parameter name is different from the form control name, then the form data will not be mapped as expected.
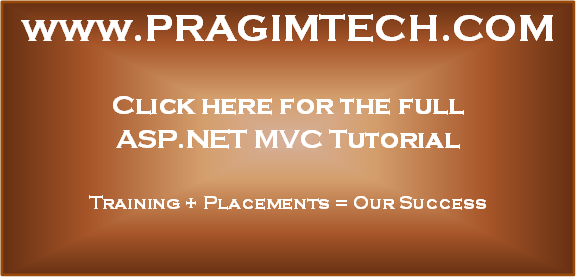
Awesome videos. Best tutorials I got from this place.Thanks a lot :-)
ReplyDeleteHi, your tutorials are realy good but i have a question guy.
ReplyDeleteIf i want load a DropDownList from SQL Server, how i can do it?
And after obtain ist value as you did it
Hello Sir,
ReplyDeleteIn tis video the parameter name are not matching exactly with Form post request control name,for e.g
the paramenter name is 'name' but control name is 'Name'
the parameter name is 'gender' but control name is 'Gender'
Could you throw some light on it.
May Bhagwan Ram Bless you and your loved ones!!
Its alright gardy, case insensitive match is okay :)
ReplyDelete