Suggested Videos
Part 6 - AccessDataSource in asp.net
Part 7 - Formatting asp.net gridview control
Part 8 - Formatting gridview using rowdatabound event
Please watch Part 8 of the asp.net gridview tutorial before proceeding with this video. I have tblEmployee table, as shown below.
Now, we want to retrieve and display above data in a gridview control. The data in the gridview control, should be formatted as shown in the image below. Notice that, based on the country culture, we need to format and display AnnualSalary column with correct currency symbol. The important thing is that, Culture column should not visible to the end user in the gridview control.
WebForm1.aspx HTML:
<div style="font-family:Arial">
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]"></asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="EmployeeId" DataSourceID="SqlDataSource1"
onrowdatabound="GridView1_RowDataBound">
<Columns>
<asp:BoundField DataField="EmployeeId" HeaderText="EmployeeId"
InsertVisible="False" ReadOnly="True" SortExpression="EmployeeId" />
<asp:BoundField DataField="FirstName" HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="AnnualSalary" HeaderText="AnnualSalary"
SortExpression="AnnualSalary" />
<asp:BoundField DataField="Country" HeaderText="Country"
SortExpression="Country" />
<asp:BoundField DataField="CountryCulture" HeaderText="CountryCulture"
SortExpression="CountryCulture" />
</Columns>
</asp:GridView>
</div>
RowDataBound event of the GridView control can be used to achieve this very easily. RowDataBound event is raised every time, a row from the datasource is bound to row in the gridview control. In row RowDataBound event retrieve country culture information and turn off the visibility of CountryCulture cell. Make sure to hide CountryCulture header table cell as well.
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
// If the row being databound is a header row
if (e.Row.RowType == DataControlRowType.Header)
{
// Hide CountryCulture header table cell
e.Row.Cells[4].Visible = false;
}
// If the row being databound is a data row
else if (e.Row.RowType == DataControlRowType.DataRow)
{
// Retrieve the unformatted salary
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
// Retrieve the culture
string countryCulture = e.Row.Cells[4].Text;
// Turn off the visibility of CountryCulture cell
e.Row.Cells[4].Visible = false;
// Format the currency using specific country culture
string formattedString = string.Format
(new System.Globalization.CultureInfo(countryCulture), "{0:c}", salary);
// Finally set the formatted currency as text for
// display purpose in the gridview control
e.Row.Cells[2].Text = formattedString;
}
}
Run the application now, and notice that, the data is displayed as expected. If you are using Google chrome browser, right click on the page, and select "View Page Source". Notice that, in the page source, the table cell (TD) for countryCulture is not rendered.
You can also use, "Display:None" style to achieve the same thing. There are 4 steps to follow.
Step 1. First, add the following CSS class. It's always a good practise, to define all the styles in a stylesheet. Add a stylesheet to your asp.net project, and copy and paste the following style class.
.DisplayNone
{
display: none
}
Step 2. Drag and drop the stylesheet on the webform. This should generate a reference to the stylesheet, using "link" element as shown below.
<link href="Styles/Site.css" rel="stylesheet" type="text/css" />
Step 3. Make the following changes to the boundcolumn, that displays "CountryCulture". Notice that, ItemStyle-CssClass and HeaderStyle-CssClass is set to "DisplayNone"
<asp:BoundField DataField="CountryCulture" HeaderText="CountryCulture"
SortExpression="CountryCulture" ItemStyle-CssClass="DisplayNone"
HeaderStyle-CssClass="DisplayNone" />
Step 4: Finally in the code-behind file, we can get rid of the code that turns off the visibility of "CountryCulture" cell in both the header and datarow. The modified code is shown below
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string countryCulture = e.Row.Cells[4].Text;
string formattedString = string.Format
(new System.Globalization.CultureInfo(countryCulture), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
}
Run the application now, and notice that, the data is displayed as expected. Now, right click on the page, and select "View Page Source". Notice that, in the page source, the table cell (TD) for countryCulture is rendered, with class="DisplayNone". So, when we use "display: none" the data gets rendered, but will not be visible in the control to the end user.
What is the difference between Visible=False and Display:None?
Visible=False will not render data, where as Display:None will render data , but will not be visible in the control.
Part 6 - AccessDataSource in asp.net
Part 7 - Formatting asp.net gridview control
Part 8 - Formatting gridview using rowdatabound event
Please watch Part 8 of the asp.net gridview tutorial before proceeding with this video. I have tblEmployee table, as shown below.

Now, we want to retrieve and display above data in a gridview control. The data in the gridview control, should be formatted as shown in the image below. Notice that, based on the country culture, we need to format and display AnnualSalary column with correct currency symbol. The important thing is that, Culture column should not visible to the end user in the gridview control.

WebForm1.aspx HTML:
<div style="font-family:Arial">
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]"></asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="EmployeeId" DataSourceID="SqlDataSource1"
onrowdatabound="GridView1_RowDataBound">
<Columns>
<asp:BoundField DataField="EmployeeId" HeaderText="EmployeeId"
InsertVisible="False" ReadOnly="True" SortExpression="EmployeeId" />
<asp:BoundField DataField="FirstName" HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="AnnualSalary" HeaderText="AnnualSalary"
SortExpression="AnnualSalary" />
<asp:BoundField DataField="Country" HeaderText="Country"
SortExpression="Country" />
<asp:BoundField DataField="CountryCulture" HeaderText="CountryCulture"
SortExpression="CountryCulture" />
</Columns>
</asp:GridView>
</div>
RowDataBound event of the GridView control can be used to achieve this very easily. RowDataBound event is raised every time, a row from the datasource is bound to row in the gridview control. In row RowDataBound event retrieve country culture information and turn off the visibility of CountryCulture cell. Make sure to hide CountryCulture header table cell as well.
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
// If the row being databound is a header row
if (e.Row.RowType == DataControlRowType.Header)
{
// Hide CountryCulture header table cell
e.Row.Cells[4].Visible = false;
}
// If the row being databound is a data row
else if (e.Row.RowType == DataControlRowType.DataRow)
{
// Retrieve the unformatted salary
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
// Retrieve the culture
string countryCulture = e.Row.Cells[4].Text;
// Turn off the visibility of CountryCulture cell
e.Row.Cells[4].Visible = false;
// Format the currency using specific country culture
string formattedString = string.Format
(new System.Globalization.CultureInfo(countryCulture), "{0:c}", salary);
// Finally set the formatted currency as text for
// display purpose in the gridview control
e.Row.Cells[2].Text = formattedString;
}
}
Run the application now, and notice that, the data is displayed as expected. If you are using Google chrome browser, right click on the page, and select "View Page Source". Notice that, in the page source, the table cell (TD) for countryCulture is not rendered.
You can also use, "Display:None" style to achieve the same thing. There are 4 steps to follow.
Step 1. First, add the following CSS class. It's always a good practise, to define all the styles in a stylesheet. Add a stylesheet to your asp.net project, and copy and paste the following style class.
.DisplayNone
{
display: none
}
Step 2. Drag and drop the stylesheet on the webform. This should generate a reference to the stylesheet, using "link" element as shown below.
<link href="Styles/Site.css" rel="stylesheet" type="text/css" />
Step 3. Make the following changes to the boundcolumn, that displays "CountryCulture". Notice that, ItemStyle-CssClass and HeaderStyle-CssClass is set to "DisplayNone"
<asp:BoundField DataField="CountryCulture" HeaderText="CountryCulture"
SortExpression="CountryCulture" ItemStyle-CssClass="DisplayNone"
HeaderStyle-CssClass="DisplayNone" />
Step 4: Finally in the code-behind file, we can get rid of the code that turns off the visibility of "CountryCulture" cell in both the header and datarow. The modified code is shown below
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string countryCulture = e.Row.Cells[4].Text;
string formattedString = string.Format
(new System.Globalization.CultureInfo(countryCulture), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
}
Run the application now, and notice that, the data is displayed as expected. Now, right click on the page, and select "View Page Source". Notice that, in the page source, the table cell (TD) for countryCulture is rendered, with class="DisplayNone". So, when we use "display: none" the data gets rendered, but will not be visible in the control to the end user.
What is the difference between Visible=False and Display:None?
Visible=False will not render data, where as Display:None will render data , but will not be visible in the control.
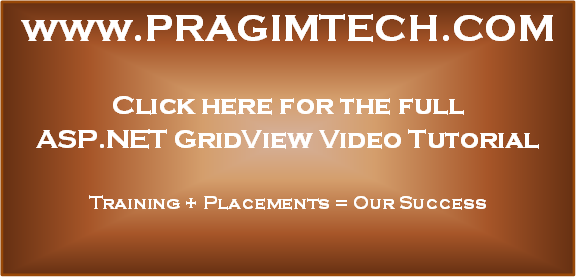
Thank you so much, it's very useful.
ReplyDelete