Suggested Video Tutorials
Dot Net Basics
C#
Sql server
ado.net
asp.net
The following are some of the different data source controls that are available in asp.net
SqlDataSource - Use to work with SQL Server, OLE DB, ODBC, or Oracle databases
ObjectDataSource - Use to work business objects, that manages data
AccessDataSource - Use to work with Microsoft Access
XmlDataSource - Use to work with XML files
LinqDataSource - Enables us to use LINQ, to retrieve and modify data from a data object
EntityDataSource - Use to work with Entity Data Model
Prior to the introduction of data source controls, developers had to write a few lines of code to retrieve and bind data with data-bound controls like DataGrid, GridView, DataList etc. With the introduction of these data source controls, we don't have to write even a single line of code, to retrieve and bind data to a data-bound control.
We will discuss about, each of these data source controls in our upcoming videos.
To bind data to a data bound control, like gridview there are 2 ways
1. Without using data-source controls
2. Using data-source controls
If we are not using data source controls, then we have to write code to
1. Read connection string
2. Create connection object
3. Create SQL Command object
4. Execute the command
5. Retrieve and bind the results to the data-bound control.
Code to bind data to a gridview control, without using data-source controls:
string cs = ConfigurationManager.ConnectionStrings["DBConnectionString"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("Select * from tblProducts", con);
con.Open();
GridView1.DataSource = cmd.ExecuteReader();
GridView1.DataBind();
}
If we are using data source controls, we don't have to write even a single line of code. All, you have to do is, drag and drop a data-source control on the webform. Configure the data-source control to connect to a data source and retrieve data. Finally associate the data-source control, to a data-bound control using "DataSourceID" property.
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblProducts]">
</asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="Id" DataSourceID="SqlDataSource1">
<Columns>
<asp:BoundField DataField="Id" HeaderText="Id" InsertVisible="False"
ReadOnly="True" SortExpression="Id" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
<asp:BoundField DataField="Description" HeaderText="Description"
SortExpression="Description" />
</Columns>
</asp:GridView>
Points to remember:
1. "ConnectionString" property of the "SqlDataSource" control is used to determine the database it has to connect, to retrieve data
2. "SelectCommand" property specifies the command that needs to be executed.
3. DataSource control is associated, with the gridview control, using "DataSourceID" property of the GridView control.
Please Note: All databound controls has DataSourceID property. A few examples of databound controls include
1. DropDownList
2. CheckBoxList
3. Repeater
4. DataList etc...
Dot Net Basics
C#
Sql server
ado.net
asp.net
The following are some of the different data source controls that are available in asp.net
SqlDataSource - Use to work with SQL Server, OLE DB, ODBC, or Oracle databases
ObjectDataSource - Use to work business objects, that manages data
AccessDataSource - Use to work with Microsoft Access
XmlDataSource - Use to work with XML files
LinqDataSource - Enables us to use LINQ, to retrieve and modify data from a data object
EntityDataSource - Use to work with Entity Data Model
Prior to the introduction of data source controls, developers had to write a few lines of code to retrieve and bind data with data-bound controls like DataGrid, GridView, DataList etc. With the introduction of these data source controls, we don't have to write even a single line of code, to retrieve and bind data to a data-bound control.
We will discuss about, each of these data source controls in our upcoming videos.
To bind data to a data bound control, like gridview there are 2 ways
1. Without using data-source controls
2. Using data-source controls
If we are not using data source controls, then we have to write code to
1. Read connection string
2. Create connection object
3. Create SQL Command object
4. Execute the command
5. Retrieve and bind the results to the data-bound control.
Code to bind data to a gridview control, without using data-source controls:
string cs = ConfigurationManager.ConnectionStrings["DBConnectionString"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("Select * from tblProducts", con);
con.Open();
GridView1.DataSource = cmd.ExecuteReader();
GridView1.DataBind();
}
If we are using data source controls, we don't have to write even a single line of code. All, you have to do is, drag and drop a data-source control on the webform. Configure the data-source control to connect to a data source and retrieve data. Finally associate the data-source control, to a data-bound control using "DataSourceID" property.
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblProducts]">
</asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="Id" DataSourceID="SqlDataSource1">
<Columns>
<asp:BoundField DataField="Id" HeaderText="Id" InsertVisible="False"
ReadOnly="True" SortExpression="Id" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
<asp:BoundField DataField="Description" HeaderText="Description"
SortExpression="Description" />
</Columns>
</asp:GridView>
Points to remember:
1. "ConnectionString" property of the "SqlDataSource" control is used to determine the database it has to connect, to retrieve data
2. "SelectCommand" property specifies the command that needs to be executed.
3. DataSource control is associated, with the gridview control, using "DataSourceID" property of the GridView control.
Please Note: All databound controls has DataSourceID property. A few examples of databound controls include
1. DropDownList
2. CheckBoxList
3. Repeater
4. DataList etc...
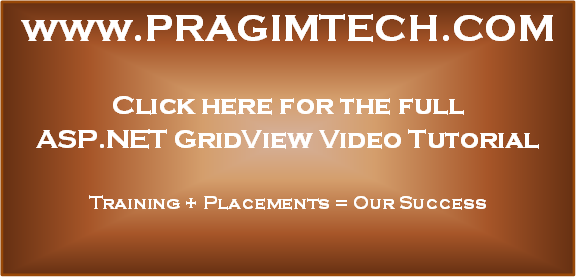
Thanks a lot dear venkat. Your Videos are much helpful. God bless you. *( Daniyal )*
ReplyDeleteHello Sir! Have you videos on ajax in asp.net? ( Daniyal)
ReplyDeleteHi sir can please upload crystal report,ssis,ssrs And ssas videos?
ReplyDeletei really enjoyed your tutorial.. you r the best
ReplyDelete