Suggested Videos
Part 39 - Angular route guards | Text | Slides
Part 40 - Angular route params | Text | Slides
Part 41 - Angular read route parameters | Text | Slides
In this video we will discuss how to subscribe to angular route parameter changes and then execute some code in response to those changes. In our previous video, we discussed there are 2 ways to read the route parameter values. We can either use the snapshot approach or observable approach. We discussed the snapshot approach in our previous video. In this video we will discuss the observable approach.
Snapshot approach works fine, if you navigate to another component before navigating from the current employee to the next employee. In our case we are always navigating back to the ListEmployeesComponent before navigating to view another employee details.
If you expect users to navigate from employee to employee directly, without navigating to another component first, then you need to use the observable approach. Notice, on the EmployeeDetailsComponent we now have "View Next Employee" button.
Here is what we want to do : Every time when we click this "View Next Employee" button, we want to display the next employee details on the page. Notice in this workflow, we are not navigating to another component before navigating from the current employee to the next employee. So the snapshot approach will not work. Let's try to use the snapshot approach and see what happens.
Modify the HTML in employee-details.component.html file to include "View Next Employee" button. Include the HTML for the button, in the panel-footer <div>
Modify the code in employee-details.component.ts file as shown below
At this point, run the project and notice that, every time we click "View Next Employee" button the route parameter value changes as expected, but the employee details displayed on the page does not change. This is because the code in ngOnInit() is executed only when the component is first loaded and initialised.
After that, every time we click "View Next Employee" button, only the route parameter value changes and the component is not reinitialised and hence the code in ngOnInit() is not executed. If you want to react to route parameter changes and execute some code every time the route parameter value changes, then you have to subscribe to the route parameter changes. So modify the code in ngOnInit() method as shown below.
With the above change in place, when we click "View Next Employee" button, the application works as expected.
Please note : paramMap is introduced in Angular 4. So if you are using Angular 4 or any version beyond it, then the above code works. If you are using Angular 2, then use params instead of paramMap as shown below.
Another important point to keep in mind : When we subscribe to an observable in a component, we also must write code to unsubscribe from the observable when the component is destroyed. However, for some observables we do not have to explicitly unsubscribe. The ActivatedRoute observable is one such observable. The angular Router destroys a routed component when it is no longer needed and the injected ActivatedRoute dies with it.
When to use snapshot over observable while reading route parameter values : Use snapshot approach if the route parameter value does not change and you only want to read the initial route parameter value. On the other hand, if you know the route parameter value changes, and if you want to react and execute some code in response to that change, then use the Observable approach.
Part 39 - Angular route guards | Text | Slides
Part 40 - Angular route params | Text | Slides
Part 41 - Angular read route parameters | Text | Slides
In this video we will discuss how to subscribe to angular route parameter changes and then execute some code in response to those changes. In our previous video, we discussed there are 2 ways to read the route parameter values. We can either use the snapshot approach or observable approach. We discussed the snapshot approach in our previous video. In this video we will discuss the observable approach.
Snapshot approach works fine, if you navigate to another component before navigating from the current employee to the next employee. In our case we are always navigating back to the ListEmployeesComponent before navigating to view another employee details.
If you expect users to navigate from employee to employee directly, without navigating to another component first, then you need to use the observable approach. Notice, on the EmployeeDetailsComponent we now have "View Next Employee" button.

Here is what we want to do : Every time when we click this "View Next Employee" button, we want to display the next employee details on the page. Notice in this workflow, we are not navigating to another component before navigating from the current employee to the next employee. So the snapshot approach will not work. Let's try to use the snapshot approach and see what happens.
Modify the HTML in employee-details.component.html file to include "View Next Employee" button. Include the HTML for the button, in the panel-footer <div>
<div
class="panel-footer">
<a class="btn
btn-primary" routerLink="/list">Back
to List</a>
<button class="btn
btn-primary pull-right" (click)="getNextEmployee()">
View Next Employee
</button>
</div>
Modify the code in employee-details.component.ts file as shown below
export
class EmployeeDetailsComponent implements
OnInit {
employee: Employee;
// Include a private field _id to keep track of the
route parameter value
private _id;
constructor(private
_route: ActivatedRoute,
private _employeeService: EmployeeService,
private _router: Router) { }
// Extract the route parameter value and retrieve
that specific
// empoyee details using the EmployeeService
ngOnInit() {
this._id = +this._route.snapshot.paramMap.get('id');
this.employee = this._employeeService.getEmployee(this._id);
}
// Everytime this method is called the employee id
value is
// incremented by 1 and then redirected to the same
route
// but with a different id parameter value
getNextEmployee() {
if (this._id < 3)
{
this._id = this._id
+ 1;
} else {
this._id = 1;
}
this._router.navigate(['/employees',
this._id]);
}
}
At this point, run the project and notice that, every time we click "View Next Employee" button the route parameter value changes as expected, but the employee details displayed on the page does not change. This is because the code in ngOnInit() is executed only when the component is first loaded and initialised.
After that, every time we click "View Next Employee" button, only the route parameter value changes and the component is not reinitialised and hence the code in ngOnInit() is not executed. If you want to react to route parameter changes and execute some code every time the route parameter value changes, then you have to subscribe to the route parameter changes. So modify the code in ngOnInit() method as shown below.
// The paramMap property returns an
Observable. So subscribe to it
// if you want to react and execute
some piece of code in response
// to the route parameter value changes
ngOnInit() {
this._route.paramMap.subscribe(params =>
{
this._id = +params.get('id');
this.employee = this._employeeService.getEmployee(this._id);
});
}
With the above change in place, when we click "View Next Employee" button, the application works as expected.
Please note : paramMap is introduced in Angular 4. So if you are using Angular 4 or any version beyond it, then the above code works. If you are using Angular 2, then use params instead of paramMap as shown below.
ngOnInit() {
this._route.params.subscribe(params =>
{
this._id = +params['id'];
this.employee = this._employeeService.getEmployee(this._id);
});
}
Another important point to keep in mind : When we subscribe to an observable in a component, we also must write code to unsubscribe from the observable when the component is destroyed. However, for some observables we do not have to explicitly unsubscribe. The ActivatedRoute observable is one such observable. The angular Router destroys a routed component when it is no longer needed and the injected ActivatedRoute dies with it.
When to use snapshot over observable while reading route parameter values : Use snapshot approach if the route parameter value does not change and you only want to read the initial route parameter value. On the other hand, if you know the route parameter value changes, and if you want to react and execute some code in response to that change, then use the Observable approach.
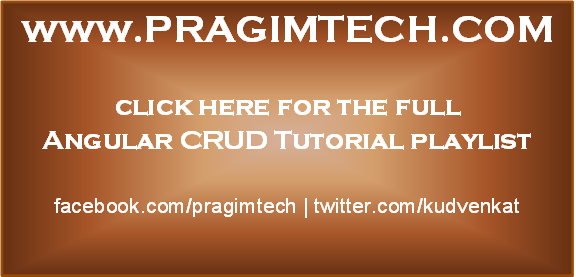
NB: //Use snapshot to get current selected employee id first, e.g from 2nd selected employee to 3rd employee directly if not it won't work properly
ReplyDelete