Suggested Videos
Part 37 - Angular component communication | Text | Slides
Part 38 - Call child component methods and properties | Text | Slides
Part 39 - Angular route guards | Text | Slides
In this video we will discuss
Create a route with parameters : To create a route with parameter include a FORWARD SLASH, a COLON and a place holder for the parameter. The example below, creates a route with parameter id.
{ path: 'employees/:id', component: EmployeeDetailsComponent }
You can have more than one parameter in a route. The following route definition creates a route with 2 parameters - id and name.
{ path: 'employees/:id/:name', component: EmployeeDetailsComponent }
Activating the route with parameters : One way to activate the route is by using the routerLink directive.
Code Explanation:
Angular CLI command to generate EmployeeDetailsComponent
ng g c employees/employee-details --no-spec --flat
list-employees.component.css
list-employees.component.ts
list-employees.component.html
app.module.ts
Part 37 - Angular component communication | Text | Slides
Part 38 - Call child component methods and properties | Text | Slides
Part 39 - Angular route guards | Text | Slides
In this video we will discuss
- Creating a route with parameters and
- Activating the route
Create a route with parameters : To create a route with parameter include a FORWARD SLASH, a COLON and a place holder for the parameter. The example below, creates a route with parameter id.
{ path: 'employees/:id', component: EmployeeDetailsComponent }
You can have more than one parameter in a route. The following route definition creates a route with 2 parameters - id and name.
{ path: 'employees/:id/:name', component: EmployeeDetailsComponent }
Activating the route with parameters : One way to activate the route is by using the routerLink directive.
<a [routerLink]="['employees',2]">
Get Employee with Id 2
</a>
Code Explanation:
- Notice in this example we are binding routerLink directive to an array.
- This array is called link parameters array.
- The first element in the array is the path of the route to the destination component.
- The second element in the array is the route parameter, in our case the employee Id.
constructor(private _router: Router) { }
onClick(employeeId: number) {
this._router.navigate(['/employees', employeeId]);
}
Angular CLI command to generate EmployeeDetailsComponent
ng g c employees/employee-details --no-spec --flat
list-employees.component.css
.pointerCursor {
cursor: pointer;
}
list-employees.component.ts
import { Component, OnInit } from '@angular/core';
import { Employee } from '../models/employee.model';
import { EmployeeService } from './employee.service';
import { Router } from '@angular/router';
@Component({
templateUrl: './list-employees.component.html',
styleUrls: ['./list-employees.component.css']
})
export class ListEmployeesComponent implements OnInit {
employees: Employee[];
constructor(private _employeeService: EmployeeService,
private _router: Router) { }
ngOnInit() {
this.employees = this._employeeService.getEmployees();
}
onClick(employeeId: number) {
this._router.navigate(['/employees', employeeId]);
}
}
list-employees.component.html
<div *ngFor="let employee of employees">
<div (click)="onClick(employee.id)" class="pointerCursor">
<app-display-employee [employee]="employee">
</app-display-employee>
</div>
</div>
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { FormsModule } from '@angular/forms';
import { BsDatepickerModule } from 'ngx-bootstrap/datepicker';
import { SelectRequiredValidatorDirective } from './shared/select-required-validator.directive';
import { AppComponent } from './app.component';
import { ListEmployeesComponent } from './employees/list-employees.component';
import { CreateEmployeeComponent } from './employees/create-employee.component';
import { ConfirmEqualValidatorDirective } from './shared/confirm-equal-validator.directive';
import { EmployeeService } from './employees/employee.service';
import { DisplayEmployeeComponent } from './employees/display-employee.component';
import {
CreateEmployeeCanDeactivateGuardService } from './employees/create-employee-can-deactivate-guard.service';
import { EmployeeDetailsComponent } from './employees/employee-details.component';
const appRoutes: Routes = [
{ path: 'list', component: ListEmployeesComponent },
{
path: 'create',
component:
CreateEmployeeComponent,
canDeactivate:
[CreateEmployeeCanDeactivateGuardService]
},
{
path: 'employees/:id', component: EmployeeDetailsComponent
},
{ path: '', redirectTo: '/list', pathMatch: 'full' }
];
@NgModule({
declarations: [
AppComponent,
ListEmployeesComponent,
CreateEmployeeComponent,
SelectRequiredValidatorDirective,
ConfirmEqualValidatorDirective,
DisplayEmployeeComponent,
EmployeeDetailsComponent
],
imports: [
BrowserModule,
FormsModule,
BsDatepickerModule.forRoot(),
RouterModule.forRoot(appRoutes)
],
providers: [EmployeeService,
CreateEmployeeCanDeactivateGuardService],
bootstrap: [AppComponent]
})
export class AppModule { }
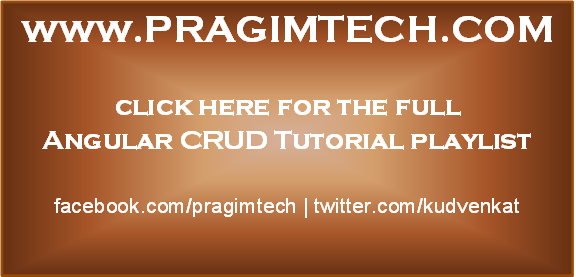
No comments:
Post a Comment
It would be great if you can help share these free resources