Suggested Videos
Part 34 - Angular component input property change detection | Text | Slides
Part 35 - Angular input change detection using property setter | Text | Slides
Part 36 - Angular property setter vs ngonchanges | Text | Slides
In this video we will discuss, how to pass data from child component to parent component.
Component Input Property
Component Output Property
If you want to pass a number instead of a string as event data, then you specify the generic argument type as number instead of string.
@Output() notify: EventEmitter<number> = new EventEmitter<number>();
If you want to be able to pass any type of data, you can use 'any' as the generic argument type.
@Output() notify: EventEmitter<any> = new EventEmitter<any>();
You can only pass one value using EventEmitter. If you want to pass more than one value using EventEmitter use a custom type like Employee as the generic argument.
@Output() notify: EventEmitter<Employee> = new EventEmitter<Employee>();
We want the "notify" custom event to be raised when we click on any of the "Employee" panel shown below.
The child component DisplayEmployeeComponent is responsible for displaying Employee details in a Bootstrap Panel. In (display-employee.component.html) file, wire up the click event handler on the <div> element that has Bootstrap panel css classes panel and panel-primary.
<div class="panel panel-primary" (click)="handleClick()">
To raise the custom event, use the emit() method of the EventEmitter object. The event payload is passed as the argument to the emit() method. So in display-employee.component.ts file include handleClick() method as shown below.
handleClick() {
this.notify.emit(this.employee);
}
Here is the complete code in display-employee.component.ts file
Now, in the parent component (list-employees.component.html) bind to the custom event raised by the child component. Notice we are using event binding to bind to the notify event. We are handling the notify event in the parent component using handleNotify($event) method. $event is the data from the child component.
In list-employees.component.ts file, include handleNotify() method as shown below. This event handler method assigns the event data received from the child component to dataFromChild property. The view template (list-employees.component.html) binds to this dataFromChild property to display the employee name and gender.
handleNotify(eventData: Employee) {
this.dataFromChild = eventData;
}
Part 34 - Angular component input property change detection | Text | Slides
Part 35 - Angular input change detection using property setter | Text | Slides
Part 36 - Angular property setter vs ngonchanges | Text | Slides
In this video we will discuss, how to pass data from child component to parent component.
Component Input Property
- In Part 33 of Angular CRUD tutorial we discussed how to pass data from parent component to child component.
- To pass data from parent component to child component we use input properties.
- In the child component we create a property with @Input decorator.
- The parent component then binds to the child component's input property.
Component Output Property
- On the other hand, to pass data from child component to parent component we use output properties.
- The child component raises an event to pass data to the parent component.
- To create and raise an event, we use EventEmitter object.
- So the output property is an event defined using EventEmitter object and decorated with @Output decorator.
- To specify the type of data that we want to pass from the child component to parent component we use the EventEmitter generic argument.
@Output() notify:
EventEmitter<string> = new
EventEmitter<string>();
If you want to pass a number instead of a string as event data, then you specify the generic argument type as number instead of string.
@Output() notify: EventEmitter<number> = new EventEmitter<number>();
If you want to be able to pass any type of data, you can use 'any' as the generic argument type.
@Output() notify: EventEmitter<any> = new EventEmitter<any>();
You can only pass one value using EventEmitter. If you want to pass more than one value using EventEmitter use a custom type like Employee as the generic argument.
@Output() notify: EventEmitter<Employee> = new EventEmitter<Employee>();
We want the "notify" custom event to be raised when we click on any of the "Employee" panel shown below.

The child component DisplayEmployeeComponent is responsible for displaying Employee details in a Bootstrap Panel. In (display-employee.component.html) file, wire up the click event handler on the <div> element that has Bootstrap panel css classes panel and panel-primary.
<div class="panel panel-primary" (click)="handleClick()">
To raise the custom event, use the emit() method of the EventEmitter object. The event payload is passed as the argument to the emit() method. So in display-employee.component.ts file include handleClick() method as shown below.
handleClick() {
this.notify.emit(this.employee);
}
Here is the complete code in display-employee.component.ts file
import
{ Component, OnInit, Input, Output, EventEmitter } from
'@angular/core';
import
{ Employee } from '../models/employee.model';
@Component({
selector: 'app-display-employee',
templateUrl: './display-employee.component.html',
styleUrls: ['./display-employee.component.css']
})
export
class DisplayEmployeeComponent implements
OnInit {
@Input() employee: Employee;
@Output() notify: EventEmitter<Employee> = new
EventEmitter<Employee>();
constructor() { }
ngOnInit() {
}
handleClick() {
this.notify.emit(this.employee);
}
}
Now, in the parent component (list-employees.component.html) bind to the custom event raised by the child component. Notice we are using event binding to bind to the notify event. We are handling the notify event in the parent component using handleNotify($event) method. $event is the data from the child component.
<h1
*ngIf="dataFromChild">
{{ dataFromChild?.name + ' ' + dataFromChild?.gender }}
</h1>
<div
*ngFor="let employee of
employees">
<app-display-employee
[employee]="employee"
(notify)="handleNotify($event)">
</app-display-employee>
</div>
In list-employees.component.ts file, include handleNotify() method as shown below. This event handler method assigns the event data received from the child component to dataFromChild property. The view template (list-employees.component.html) binds to this dataFromChild property to display the employee name and gender.
handleNotify(eventData: Employee) {
this.dataFromChild = eventData;
}
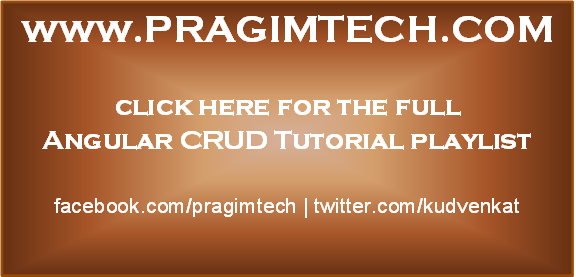
No comments:
Post a Comment
It would be great if you can help share these free resources