Suggested Videos
Part 35 - Angular input change detection using property setter | Text | Slides
Part 36 - Angular property setter vs ngonchanges | Text | Slides
Part 37 - Angular component communication | Text | Slides
In this video we will discuss how to call child component methods and properties from parent component. One way to do this is by using output properties. We discussed output properties in Part 37 of Angular CRUD tutorial. Another way, to pass data from the child component to the parent component is by using a template reference variable.
Let us understand this with an example. We want to do the same thing that we did with Output properties in Part 37. The child component DisplayEmployeeComponent is responsible for displaying each Employee details in a Bootstrap Panel.
Here is what we want to do. When we click on any of the employee panel we want to display that employee name and gender as shown in the image below. So we need to pass employee name and gender from the child component (DisplayEmployeeComponent) to parent component (ListEmployeesComponent).
Here is the child component class. Notice the class has employee property and getNameAndGender() method. We will be calling both of these from the parent component (ListEmployeesComponent) using a template reference variable.
Code in Parent Component View Template (list-employees.component.html)
Code Explanation :
Calling the child component property using template reference variable : Notice in the example below, we are calling the child component public property employee using the template reference variable childComponent.
Even now, when you click on an employee panel, you will see that employee's name and gender displayed by the <h1> element exactly the same way as before.
Summary : There are 2 ways to pass data from Child Component to Parent Component
Part 35 - Angular input change detection using property setter | Text | Slides
Part 36 - Angular property setter vs ngonchanges | Text | Slides
Part 37 - Angular component communication | Text | Slides
In this video we will discuss how to call child component methods and properties from parent component. One way to do this is by using output properties. We discussed output properties in Part 37 of Angular CRUD tutorial. Another way, to pass data from the child component to the parent component is by using a template reference variable.
Let us understand this with an example. We want to do the same thing that we did with Output properties in Part 37. The child component DisplayEmployeeComponent is responsible for displaying each Employee details in a Bootstrap Panel.
Here is what we want to do. When we click on any of the employee panel we want to display that employee name and gender as shown in the image below. So we need to pass employee name and gender from the child component (DisplayEmployeeComponent) to parent component (ListEmployeesComponent).

Here is the child component class. Notice the class has employee property and getNameAndGender() method. We will be calling both of these from the parent component (ListEmployeesComponent) using a template reference variable.
export class DisplayEmployeeComponent {
@Input() employee: Employee;
getNameAndGender(): string {
return this.employee.name + ' ' + this.employee.gender;
}
}
Code in Parent Component View Template (list-employees.component.html)
<h1 #h1Variable></h1>
<div *ngFor="let employee of employees">
<div (click)="h1Variable.innerHTML = childComponent.getNameAndGender()">
<app-employee-display [employee]="employee" #childComponent>
</app-employee-display>
</div>
</div>
Code Explanation :
- #childComponent is the template reference variable to the child component. Using this template variable we can call child component public property (employee) and method (getNameAndGender())
- <div (click)="handleClick(childComponent.getNameAndGender())">. Using the template reference variable we are calling the child component method getNameAndGender(). The value this method returns is assigned to the innerHTML property of the <h1> element. #h1Variable is the template reference variable for <h1> element.
Calling the child component property using template reference variable : Notice in the example below, we are calling the child component public property employee using the template reference variable childComponent.
<h1 #h1Variable></h1>
<div *ngFor="let employee of employees">
<div (click)="h1Variable.innerHTML = childComponent.employee.name + ' ' +
childComponent.employee.gender">
<app-employee-display [employee]="employee" #childComponent>
</app-employee-display>
</div>
</div>
Even now, when you click on an employee panel, you will see that employee's name and gender displayed by the <h1> element exactly the same way as before.
Summary : There are 2 ways to pass data from Child Component to Parent Component
- Output Properties
- Template Reference Variable
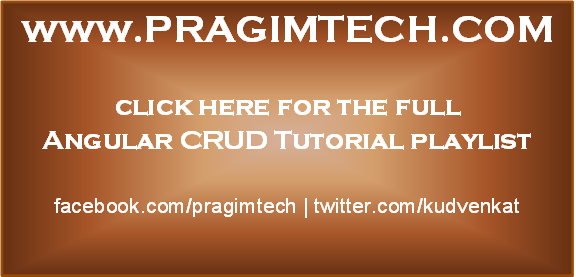
Superb Concept Explanation
ReplyDelete