Suggested Videos
Part 38 - Call child component methods and properties | Text | Slides
Part 39 - Angular route guards | Text | Slides
Part 40 - Angular route params | Text | Slides
In this video we will discuss, reading route parameter values in Angular 2 and later versions.
To read the route parameter value use Angular ActivatedRoute service.
constructor(private _route: ActivatedRoute) { }
ngOnInit() {
const id = +this._route.snapshot.params['id'];
}
Since Angular 4, params have been deprecated in favor of the new interface paramMap. So if you are using Angular 4 or above, use paramMap instead of params.
ngOnInit() {
const id = +this._route.snapshot.paramMap.get('id');
}
Introduce getEmployee() method in employee.service.ts file
getEmployee(id: number): Employee {
return this.listEmployees.find(e => e.id === id);
}
employee-details.component.html
employee-details.component.ts
employee-details.component.css
Part 38 - Call child component methods and properties | Text | Slides
Part 39 - Angular route guards | Text | Slides
Part 40 - Angular route params | Text | Slides
In this video we will discuss, reading route parameter values in Angular 2 and later versions.
To read the route parameter value use Angular ActivatedRoute service.
- There are 2 ways to read the route parameter value.
- We can either use the snapshot approach or observable approach.
- Snapshot approach works fine, if you navigate to another component before navigating from the current employee to the next employee. In our case we are always navigating back to the ListEmployeesComponent before navigating to view another employee details.
- If you expect users to navigate from employee to employee directly, without navigating to another component first, then you need to use the observable approach. We will discuss the observable approach in our next video.
constructor(private _route: ActivatedRoute) { }
ngOnInit() {
const id = +this._route.snapshot.params['id'];
}
Since Angular 4, params have been deprecated in favor of the new interface paramMap. So if you are using Angular 4 or above, use paramMap instead of params.
ngOnInit() {
const id = +this._route.snapshot.paramMap.get('id');
}
Introduce getEmployee() method in employee.service.ts file
getEmployee(id: number): Employee {
return this.listEmployees.find(e => e.id === id);
}
employee-details.component.html
<div
class="panel
panel-primary">
<div class="panel-heading">
<h3 class="panel-title">{{employee.name}}</h3>
</div>
<div class="panel-body">
<div class="col-xs-10">
<div class="row
vertical-align">
<div class="col-xs-4">
<img
class="imageClass"
[src]="employee.photoPath"
/>
</div>
<div class="col-xs-8">
<div
class="row">
<div
class="col-xs-6">
Gender
</div>
<div
class="col-xs-6">
: {{employee.gender}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Date of Birth
</div>
<div
class="col-xs-6">
: {{employee.dateOfBirth | date}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Contact Preference
</div>
<div
class="col-xs-6">
: {{employee.contactPreference}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Phone
</div>
<div
class="col-xs-6">
: {{employee.phoneNumber}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Email
</div>
<div
class="col-xs-6">
: {{employee.email}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Department
</div>
<div
class="col-xs-6"
[ngSwitch]="employee.department">
:
<span
*ngSwitchCase="1">Help
Desk</span>
<span
*ngSwitchCase="2">HR</span>
<span
*ngSwitchCase="3">IT</span>
<span
*ngSwitchCase="4">Payroll</span>
<span
*ngSwitchDefault>N/A</span>
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Is Active
</div>
<div
class="col-xs-6">
: {{employee.isActive}}
</div>
</div>
</div>
</div>
</div>
</div>
<div class="panel-footer">
<a class="btn
btn-primary" routerLink="/list">
Back to List
</a>
</div>
</div>
employee-details.component.ts
import
{ Component, OnInit } from '@angular/core';
import
{ Employee } from '../models/employee.model';
import
{ ActivatedRoute } from '@angular/router';
import
{ EmployeeService } from './employee.service';
@Component({
selector: 'app-employee-details',
templateUrl: './employee-details.component.html',
styleUrls: ['./employee-details.component.css']
})
export
class EmployeeDetailsComponent implements
OnInit {
employee: Employee;
constructor(private
_route: ActivatedRoute,
private _employeeService: EmployeeService) { }
ngOnInit() {
const id = +this._route.snapshot.paramMap.get('id');
this.employee = this._employeeService.getEmployee(id);
}
}
employee-details.component.css
.imageClass{
width:200px;
height:200px;
}
.vertical-align{
display: flex;
align-items: center;
}
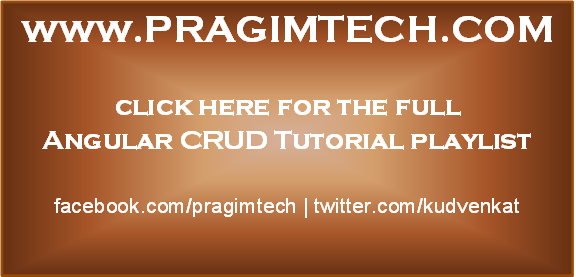
No comments:
Post a Comment
It would be great if you can help share these free resources