Suggested Videos
Part 7 - jQuery attribute selector
Part 8 - jQuery attribute value selector
Part 9 - jQuery case insensitive attribute selector
In this video we will discuss the difference between $(input) and $(:input) selectors
$(':input') selects all input, textarea, select and button elements where as $('input') just selects elements with an input tag.
Consider the web page below
The following is the HTML of the above page
Now we want to get the text value from all the textboxes. On this page we have 2 textboxes
1. First Name
2. Last Name
jQuery code to get textbox value using $(input)
jQuery code to get textbox value using $(:input)
Which one is better for performance $('input[type="text"]') or $(':input[type="text"]')
$('input[type="text"]') is better for performance over $(':input[type="text"]').
This is because $(':input[type="text"]') needs to scan all input elements, textarea, select etc, where as $('input[type="text"]') scans only input elements.
So if you want to find elements with an input tag, it is always better to use $('input[type="text"]') over $(':input[type="text"]')
Part 7 - jQuery attribute selector
Part 8 - jQuery attribute value selector
Part 9 - jQuery case insensitive attribute selector
In this video we will discuss the difference between $(input) and $(:input) selectors
$(':input') selects all input, textarea, select and button elements where as $('input') just selects elements with an input tag.
Consider the web page below

The following is the HTML of the above page
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
</head>
<body style="font-family:Arial">
First Name : <input type="text" value="John" />
<br /><br />
Last Name : <input type="text" value="Major" />
<br /><br />
Gender :
<input type="radio" name="gender" checked="checked" value="Male">Male
<input type="radio" name="gender" value="Female">Female
<br /><br />
Skills :
<input type="checkbox" name="skills" checked="checked"
value="JavaScript" />JavaScript
<input type="checkbox" name="skills" checked="checked"
value="jQuery" />jQuery
<input type="checkbox" name="skills" value="C#" />C#
<br /><br />
Country:
<select>
<option selected="selected" value="USA">USA</option>
<option value="India">India</option>
<option value="UK">UK</option>
</select>
<br /><br />
Summary :
<br />
<textarea>
I am a Senior Dot Net Developer with 10
years experience
</textarea>
<br /><br />
<input type="submit" value="submit" />
</body>
</html>
Now we want to get the text value from all the textboxes. On this page we have 2 textboxes
1. First Name
2. Last Name
jQuery code to get textbox value using $(input)
<script type="text/javascript">
$(document).ready(function () {
$('input[type="text"]').each(function () {
alert($(this).val());
});
});
</script>
jQuery code to get textbox value using $(:input)
<script type="text/javascript">
$(document).ready(function () {
$(':input[type="text"]').each(function () {
alert($(this).val());
});
});
</script>
Which one is better for performance $('input[type="text"]') or $(':input[type="text"]')
$('input[type="text"]') is better for performance over $(':input[type="text"]').
This is because $(':input[type="text"]') needs to scan all input elements, textarea, select etc, where as $('input[type="text"]') scans only input elements.
So if you want to find elements with an input tag, it is always better to use $('input[type="text"]') over $(':input[type="text"]')
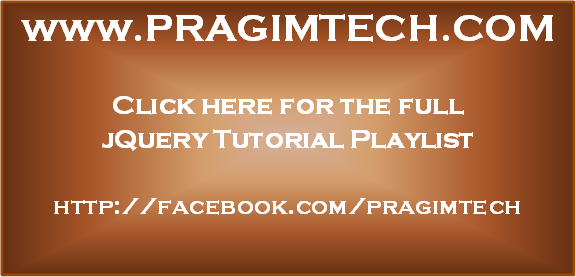
Please change the code $(':input[type="text"]') as $(':input').
ReplyDeleteThanku bro !!
DeleteThat code didn't work and i checked hundred times and in the end i saw your comment and edit that code,,,, it really worked!! Thanks man!!