Suggested Videos
Part 5 - jQuery Element Selector
Part 6 - jQuery class selector
Part 7 - jQuery attribute selector
In this video we will discuss
Attribute Equals Selector [name="value"]
Attribute Not Equal Selector [name!="value"]
Attribute Contains Selector [name*="value"]
Attribute Contains Word Selector [name~="value"]
Attribute Contains Prefix Selector [name|="value"]
Attribute Starts With Selector [name^="value"]
Attribute Ends With Selector [name$="value"]
This is continuation to Part 7, please watch Part 7 before proceeding.
Selects all elements that have title attribute value equal to div1Title
$('[title="div1Title"]')
Selects all elements that have title attribute value not equal to div1Title
$('[title!="div1Title"]')
Selects all elements that have title attribute value containing the given substring - Title
$('[title*="Title"]')
Selects all elements that have title attribute value containing the given word - mySpan, delimited by spaces
$('[title~="mySpan"]')
Selects all elements that have title attribute value equal to myTitle or starting with myTitle followed by a hyphen (-)
$('[title|="myTitle"]')
Selects all elements that have title attribute value starting with div
$('[title^="div"]')
Selects all elements that have title attribute value ending with Heading
$('[title$="Heading"]')
Selects all elements that have title attribute value equal to div1Title and sets 5px solid red border
Selects all div elements that have title attribute value not equal to div1Title and sets 5px solid red border
THIS IS
EQUIVALENT TO
Selects all elements that have title attribute value containing the given substring - Title, and sets 5px solid red border
Selects all elements that have title attribute value containing the given word - mySpan, delimited by spaces, and sets 5px solid red border
Selects all elements that have title attribute value equal to myTitle or starting with myTitle followed by a hyphen (-) and sets 5px solid red border
Selects all elements that have title attribute value starting with div and sets 5px solid red border
Selects all elements that have title attribute value ending with Heading and sets 5px solid red border
Part 5 - jQuery Element Selector
Part 6 - jQuery class selector
Part 7 - jQuery attribute selector
In this video we will discuss
Attribute Equals Selector [name="value"]
Attribute Not Equal Selector [name!="value"]
Attribute Contains Selector [name*="value"]
Attribute Contains Word Selector [name~="value"]
Attribute Contains Prefix Selector [name|="value"]
Attribute Starts With Selector [name^="value"]
Attribute Ends With Selector [name$="value"]
This is continuation to Part 7, please watch Part 7 before proceeding.
Selects all elements that have title attribute value equal to div1Title
$('[title="div1Title"]')
Selects all elements that have title attribute value not equal to div1Title
$('[title!="div1Title"]')
Selects all elements that have title attribute value containing the given substring - Title
$('[title*="Title"]')
Selects all elements that have title attribute value containing the given word - mySpan, delimited by spaces
$('[title~="mySpan"]')
Selects all elements that have title attribute value equal to myTitle or starting with myTitle followed by a hyphen (-)
$('[title|="myTitle"]')
Selects all elements that have title attribute value starting with div
$('[title^="div"]')
Selects all elements that have title attribute value ending with Heading
$('[title$="Heading"]')
Selects all elements that have title attribute value equal to div1Title and sets 5px solid red border
<html>
<head>
<title></title>
<script src="Scripts/jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('[title="div1Title"]').css('border', '5px solid red');
});
</script>
</head>
<body>
<div title="div1Title">
DIV 1
</div>
<br />
<div title="div2Title">
DIV 2
</div>
<p title="myTitle-paragraph">
This is a paragraph
</p>
<p title="myTitleHeading">
This is a paragraph
</p>
<span title="div1Title">
SAPN 1
</span>
<br /><br />
<span title="mySpan
Heading">
SPAN 2
</span>
</body>
</html>
Selects all div elements that have title attribute value not equal to div1Title and sets 5px solid red border
<script type="text/javascript">
$(document).ready(function () {
$('div[title!="div1Title"]').css('border', '5px solid red');
});
</script>
THIS IS
$('div[title!="div1Title"]').css('border', '5px solid red');
$('div:not([title="div1Title"])').css('border', '5px solid red');
Selects all elements that have title attribute value containing the given substring - Title, and sets 5px solid red border
<script type="text/javascript">
$(document).ready(function () {
$('[title*="Title"]').css('border', '5px solid red');
});
</script>
Selects all elements that have title attribute value containing the given word - mySpan, delimited by spaces, and sets 5px solid red border
<script type="text/javascript">
$(document).ready(function () {
$('[title~="mySpan"]').css('border', '5px solid red');
});
</script>
Selects all elements that have title attribute value equal to myTitle or starting with myTitle followed by a hyphen (-) and sets 5px solid red border
<script type="text/javascript">
$(document).ready(function () {
$('[title|="myTitle"]').css('border', '5px solid red');
});
</script>
Selects all elements that have title attribute value starting with div and sets 5px solid red border
<script type="text/javascript">
$(document).ready(function () {
$('[title^="div"]').css('border', '5px solid red');
});
</script>
Selects all elements that have title attribute value ending with Heading and sets 5px solid red border
<script type="text/javascript">
$(document).ready(function () {
$('[title$="Heading"]').css('border', '5px solid red');
});
</script>
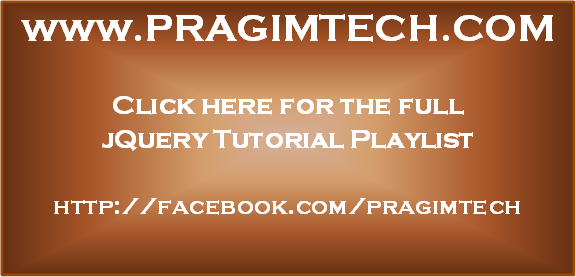
I'm still with you... Keep up the good work...
ReplyDeleteI am also still with you. You are definitely the best tutorial on the web. Regards Raphael p.s this was shared to me by my friend Amrindarjet Singh and I have shared it with other programmers.
ReplyDeleteThank you so much sir, I always give reference to your tutorials to every students or programmers.
ReplyDelete