Suggested Videos
Part 6 - jQuery class selector
Part 7 - jQuery attribute selector
Part 8 - jQuery attribute value selector
In this video we will discuss, how to write a jQuery case-insensitive attribute value selector. Let us understand this with an example.
The following example, only selects DIV 1. This is because jQuery attribute value selector is case-sensitive.
Use the following code to make the jQuery attribute value selector case-insensitive
The above script should select all the 3 divs.
Now let us look at an example of making attribute contains selector [name*="value"], case-insensitive.
The following example, selects only DIV 1 element. This is because the attribute contains selector is case-sensitive.
To make attribute contains selector case-insensitive, use filter() method and regular expression as shown below.
OR
The above script should select all the 3 divs.
Visual Studio Keyboard Shortcuts
Convert Selected Text to Upper Case - CTRL + SHIFT + U
Convert Selected Text to Lower Case - CTRL + U
Part 6 - jQuery class selector
Part 7 - jQuery attribute selector
Part 8 - jQuery attribute value selector
In this video we will discuss, how to write a jQuery case-insensitive attribute value selector. Let us understand this with an example.
The following example, only selects DIV 1. This is because jQuery attribute value selector is case-sensitive.
<html>
<head>
<title></title>
<script src="Scripts/jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('div[title="DivTitle"]').css('border', '3px solid red');
});
</script>
</head>
<body>
<div title="DivTitle">
DIV 1
</div>
<br />
<div title="DIVTITLE">
DIV 2
</div>
<br />
<div title="divtitle">
DIV 3
</div>
</body>
</html>

Use the following code to make the jQuery attribute value selector case-insensitive
<script type="text/javascript">
$(document).ready(function () {
$('div[title]').filter(function () {
return $(this).attr('title').toLowerCase() == 'divtitle';
}).css('border', '3px solid red');
});
</script>
The above script should select all the 3 divs.

Now let us look at an example of making attribute contains selector [name*="value"], case-insensitive.
The following example, selects only DIV 1 element. This is because the attribute contains selector is case-sensitive.
<html>
<head>
<title></title>
<script src="Scripts/jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('div[title*="Div"]').css('border', '3px solid red');
});
</script>
</head>
<body>
<div title="DivTitle1">
DIV 1
</div>
<br />
<div title="DIVTITLE2">
DIV 2
</div>
<br />
<div title="divtitle3">
DIV 3
</div>
</body>
</html>
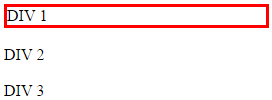
To make attribute contains selector case-insensitive, use filter() method and regular expression as shown below.
<script type="text/javascript">
$(document).ready(function () {
$('div[title]').filter(function () {
return (/Div/i).test($(this).attr('title'));
}).css('border', '3px solid red');
});
</script>
OR
<script type="text/javascript">
$(document).ready(function () {
$('div[title]').filter(function () {
return RegExp('Div', 'i').test($(this).attr('title'));
}).css('border', '3px solid red');
});
</script>
The above script should select all the 3 divs.

Visual Studio Keyboard Shortcuts
Convert Selected Text to Upper Case - CTRL + SHIFT + U
Convert Selected Text to Lower Case - CTRL + U
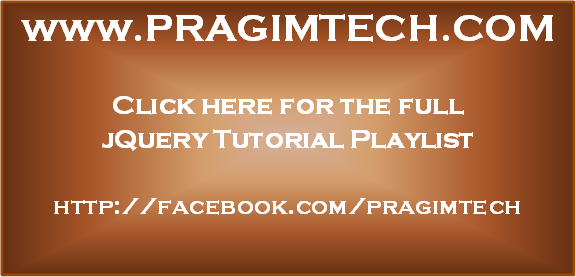
No comments:
Post a Comment
It would be great if you can help share these free resources