Suggested Videos
Part 1 - Adding JavaScript to ASP.NET controls
Part 2 - JavaScript confirm on GridView delete
Part 3 - JavaScript to select all checkboxes in GridView
In this video we will discuss how to change GridView row color when CheckBox is checked. We want to achieve this using JavaScript.
The background color of the rows that are selected should be grey, and the rows that are not selected should be white.
We will continue with the example that we worked with in Part 3 of JavaScript with ASP.NET tutorial.
Modify HeaderCheckBoxClick() and ChildCheckBoxClick() JavaScript functions as shown below.
The following code makes the text in a GridView row bold on mouseover and normal on mouseout
Part 1 - Adding JavaScript to ASP.NET controls
Part 2 - JavaScript confirm on GridView delete
Part 3 - JavaScript to select all checkboxes in GridView
In this video we will discuss how to change GridView row color when CheckBox is checked. We want to achieve this using JavaScript.
The background color of the rows that are selected should be grey, and the rows that are not selected should be white.

We will continue with the example that we worked with in Part 3 of JavaScript with ASP.NET tutorial.
Modify HeaderCheckBoxClick() and ChildCheckBoxClick() JavaScript functions as shown below.
<script type="text/javascript" language="javascript">
function HeaderCheckBoxClick(checkbox)
{
var gridView = document.getElementById("GridView1");
for (i = 1; i < gridView.rows.length;
i++)
{
gridView.rows[i].cells[0].getElementsByTagName("INPUT")[0].checked
= checkbox.checked;
// Depending on whether the
checkbox in the header is checked or
// unchecked change the background
color of the data rows in the GridView
if (checkbox.checked)
{
gridView.rows[i].style.background
= '#CCCCCC';
}
else
{
gridView.rows[i].style.background
= '#FFFFFF';
}
}
}
function ChildCheckBoxClick(checkbox)
{
var atleastOneCheckBoxUnchecked = false;
var gridView = document.getElementById("GridView1");
for (i = 1; i < gridView.rows.length;
i++)
{
// Depending on whether the
checkbox in the data row is checked or
// unchecked change the background
color of the rows in the GridView
if (gridView.rows[i].cells[0].getElementsByTagName("INPUT")[0].checked ==
false)
{
gridView.rows[i].style.background
= '#FFFFFF';
atleastOneCheckBoxUnchecked =
true;
}
else
{
gridView.rows[i].style.background
= '#CCCCCC';
}
}
gridView.rows[0].cells[0].getElementsByTagName("INPUT")[0].checked
= !atleastOneCheckBoxUnchecked;
}
</script>
The following code makes the text in a GridView row bold on mouseover and normal on mouseout
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs
e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
e.Row.Attributes.Add("onmouseover",
"this.style.fontWeight = 'bold';");
e.Row.Attributes.Add("onmouseout",
"this.style.fontWeight= 'normal';");
}
}
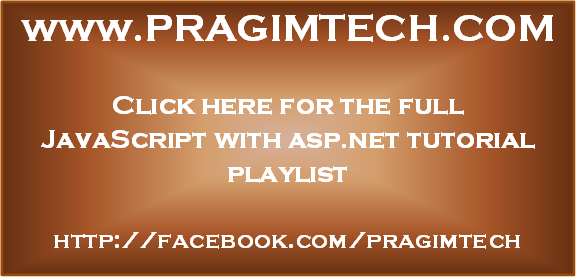
No comments:
Post a Comment
It would be great if you can help share these free resources