Suggested Video Tutorials
JavaScript Tutorial
ASP.NET Tutorial
WCF Tutorial
In this video we will discuss different options available to add JavaScript to ASP.NET server controls.
Adding JavaScript to ImageButton in ASP.NET :
When the mouse is moved over the ImageButton, we want the button to look as shown in the image below.
When the mouse is not over the ImageButton, we want the button to look as shown in the image below.
To download the images
If you are using Internet Explorer, right click on the image and select "Save picture as" option
If you are using Google Chrome, right click on the image and select "Save image as" option
Add a new folder to your web application project. Name it Images. Copy the 2 images you have downloaded in this folder. Add a web page to your project. At this point your Solution Explorer should look as shown below.
Adding JavaScript to ASP.NET server control declaratively : Copy and paste the following HTML in WebForm1.aspx. In this example we are declaratively adding the JavaScript that we want to execute in response to onmouseover and onmouseout events. onmouseover and onmouseout attributes does not correspond to any of the ImageButton control properties, so they will be passed through to the browser as is.
The JavaScript that we want to execute in response to onmouseover and onmouseout events can also be present in separate JavaScript functions as shown below.
Adding JavaScript to ASP.NET server control at runtime :
ASPX : Notice that in the declarative markup we removed onmouseover and onmouseout attributes
ASPX.CS :
Adding JavaScript to ASP.NET Button onclick client event declaratively : With Button, LinkButton and ImageButton controls, OnClick attribute corresponds to server side click event. For this reason you cannot use OnClick attribute to add JavaScript that you want to execute in response to client side click event. The following code throws an error.
With Button, LinkButton and ImageButton controls to associate JavaScript that you want to execute in response to client side click event use OnClientClick attribute as shown below.
Adding JavaScript to ASP.NET Button onclick client event at runtime : If you are adding JavaScript at runtime, then you will have to use onclick.
ASPX :
ASPX.CS
JavaScript Tutorial
ASP.NET Tutorial
WCF Tutorial
In this video we will discuss different options available to add JavaScript to ASP.NET server controls.
Adding JavaScript to ImageButton in ASP.NET :
When the mouse is moved over the ImageButton, we want the button to look as shown in the image below.

When the mouse is not over the ImageButton, we want the button to look as shown in the image below.

To download the images
If you are using Internet Explorer, right click on the image and select "Save picture as" option
If you are using Google Chrome, right click on the image and select "Save image as" option
Add a new folder to your web application project. Name it Images. Copy the 2 images you have downloaded in this folder. Add a web page to your project. At this point your Solution Explorer should look as shown below.

Adding JavaScript to ASP.NET server control declaratively : Copy and paste the following HTML in WebForm1.aspx. In this example we are declaratively adding the JavaScript that we want to execute in response to onmouseover and onmouseout events. onmouseover and onmouseout attributes does not correspond to any of the ImageButton control properties, so they will be passed through to the browser as is.
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="/Images/blueButton.png"
onmouseover="this.src='/Images/greenButton.png'"
onmouseout="this.src='/Images/blueButton.png'" />
The JavaScript that we want to execute in response to onmouseover and onmouseout events can also be present in separate JavaScript functions as shown below.
<form id="form1" runat="server">
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="/Images/blueButton.png"
onmouseover="changeImageOnMouseOver();" onmouseout="changeImageOnMouseOut();" />
<script type="text/javascript">
function changeImageOnMouseOver()
{
document.getElementById("ImageButton1").src = "/Images/greenButton.png";
}
function changeImageOnMouseOut()
{
document.getElementById("ImageButton1").src = "/Images/blueButton.png";
}
</script>
</form>
Adding JavaScript to ASP.NET server control at runtime :
ASPX : Notice that in the declarative markup we removed onmouseover and onmouseout attributes
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="/Images/blueButton.png"/>
ASPX.CS :
protected void Page_Load(object sender, EventArgs
e)
{
ImageButton1.Attributes.Add("onmouseover", "this.src='/Images/greenButton.png'");
ImageButton1.Attributes.Add("onmouseout", "this.src='/Images/blueButton.png'");
}
Adding JavaScript to ASP.NET Button onclick client event declaratively : With Button, LinkButton and ImageButton controls, OnClick attribute corresponds to server side click event. For this reason you cannot use OnClick attribute to add JavaScript that you want to execute in response to client side click event. The following code throws an error.
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="/Images/blueButton.png"
OnClick="return
confirm('Are you sure you want to submit')"/>
With Button, LinkButton and ImageButton controls to associate JavaScript that you want to execute in response to client side click event use OnClientClick attribute as shown below.
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="/Images/blueButton.png"
OnClientClick="return
confirm('Are you sure you want to submit')"/>
Adding JavaScript to ASP.NET Button onclick client event at runtime : If you are adding JavaScript at runtime, then you will have to use onclick.
ASPX :
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="/Images/blueButton.png"/>
ASPX.CS
protected void Page_Load(object sender, EventArgs
e)
{
ImageButton1.Attributes.Add("onclick",
"return confirm('Are you sure you want
to submit');");
}
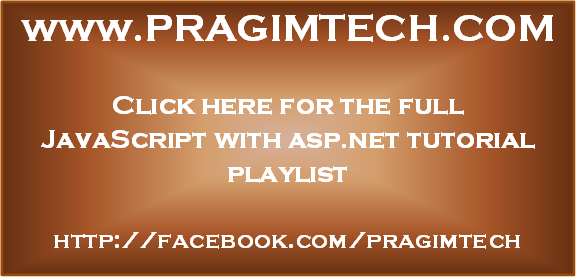
ImageUrl="/Images/blueButton.png" is not showing image, but if i write ImageUrl="~/Images/blueButton.png" is working as expected, pls tell me diffrence b/w these two,
ReplyDeletethanks...
Hi krishna,
DeleteI think "~" sign is a special character that is usually used to set URL paths for Asp.net Server controls and this character instructs the Asp.net run time to resolve the relative path of the server control.
In short and Simple "/Images/blueButton.png" is Searching a folder under WebForm1.aspx but for this you should go to one directory up to the root directory i.e. "~/Images/blueButton.png". "~" sign refers to the root directory.
I hope this is useful for you.
Thanks
~ special character defines the root directory of your application
ReplyDeleteSIR, Can you please make videos on WPF?
ReplyDelete