Suggested Videos
Part 3 - Creating asp.net chart data programmatically
Part 4 - How to bind data from database to chart control in asp.net
Part 5 - ASP.NET Chart DataSource
In Part 5 of this video series, we discussed binding data to the chart control using DataSource property. In this video we will discuss binding data to chart control using DataBindTable() method. We will continue with the example we worked with in Part 5, so please watch Part 5 before proceeding with this video.
Steps to use DataBindTable() method
Step 1 : When the DataBindTable() method is called, it automatically creates and adds a new series to the chart control. So, there is no need to specify the series in the HTML markup of the chart control. Delete the Series element from ChartControl.
<asp:Series Name="Series1" ChartArea="ChartArea1">
</asp:Series>
Step 2 : Modify the code in the code-behind file as shown below. The code is commented and self explanatory.
Part 3 - Creating asp.net chart data programmatically
Part 4 - How to bind data from database to chart control in asp.net
Part 5 - ASP.NET Chart DataSource
In Part 5 of this video series, we discussed binding data to the chart control using DataSource property. In this video we will discuss binding data to chart control using DataBindTable() method. We will continue with the example we worked with in Part 5, so please watch Part 5 before proceeding with this video.
Steps to use DataBindTable() method
Step 1 : When the DataBindTable() method is called, it automatically creates and adds a new series to the chart control. So, there is no need to specify the series in the HTML markup of the chart control. Delete the Series element from ChartControl.
<asp:Chart ID="Chart1" runat="server" Width="350px">
<Titles>
<asp:Title Text="Total marks of students">
</asp:Title>
</Titles>
<Series>
</Series>
<ChartAreas>
<asp:ChartArea Name="ChartArea1">
<AxisX Title="Student Name">
</AxisX>
<AxisY Title="Total Marks">
</AxisY>
</asp:ChartArea>
</ChartAreas>
</asp:Chart>
Step 2 : Modify the code in the code-behind file as shown below. The code is commented and self explanatory.
using System;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.UI.DataVisualization.Charting;
using System.Web.UI.WebControls;
namespace ChartsDemo
{
public partial class
WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{
if (!IsPostBack)
{
GetChartData();
GetChartTypes();
}
}
private void GetChartData()
{
string cs = ConfigurationManager.ConnectionStrings["CS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
// Query to retrieve
the 2 columns (1 column for X-AXIS and
// the other for
Y-AXIS) of data required for the chart control
SqlCommand cmd = new SqlCommand
("Select
StudentName, TotalMarks from Students", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
// Pass the
datareader object that contains the chart data and
// specify the column
to be used for X-AXIS values. The other
// column values will
be automatically used for Y-AXIS
Chart1.DataBindTable(rdr, "StudentName");
}
}
private void GetChartTypes()
{
foreach (int chartType in Enum.GetValues(typeof(SeriesChartType)))
{
ListItem li = new ListItem(Enum.GetName(
typeof(SeriesChartType), chartType), chartType.ToString());
DropDownList1.Items.Add(li);
}
}
protected void DropDownList1_SelectedIndexChanged(object
sender, EventArgs e)
{
GetChartData();
// Retrieve the series by index and
then
// set the ChartType property value
this.Chart1.Series[0].ChartType = (SeriesChartType)Enum.Parse(
typeof(SeriesChartType), DropDownList1.SelectedValue);
// Alternatively, FirstOrDefault()
linq method can also be used
//
this.Chart1.Series.FirstOrDefault().ChartType = (SeriesChartType)
// Enum.Parse(typeof(SeriesChartType),
DropDownList1.SelectedValue);
}
}
}
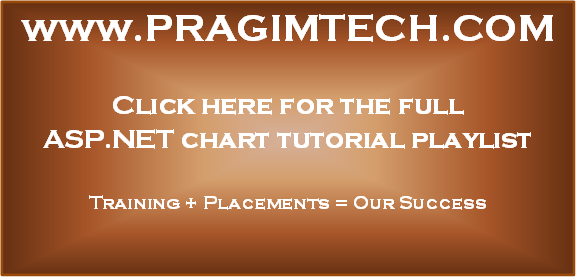
No comments:
Post a Comment
It would be great if you can help share these free resources