Suggested Videos
Part 2 - How to set asp.net chart control ChartType property dynamically
Part 3 - Creating asp.net chart data programmatically
Part 4 - How to bind data from database to chart control in asp.net
In Part 4 of this video series, we discussed retrieving data from the database and then programatically adding data to the chart control using AddXY() method. In this video we will discuss binding database data to the asp.net chart control using DataSource property. We will continue with the example we worked with in Part 4, so please watch Part 4 before proceeding with this video.
Binding data to Chart control using DataSource property is similar to how we bind data to any databound control (GridView, DropDownList etc) in asp.net.
Modify the code in the code-behind file as shown below. The code is commented and self explanatory.
Part 2 - How to set asp.net chart control ChartType property dynamically
Part 3 - Creating asp.net chart data programmatically
Part 4 - How to bind data from database to chart control in asp.net
In Part 4 of this video series, we discussed retrieving data from the database and then programatically adding data to the chart control using AddXY() method. In this video we will discuss binding database data to the asp.net chart control using DataSource property. We will continue with the example we worked with in Part 4, so please watch Part 4 before proceeding with this video.
Binding data to Chart control using DataSource property is similar to how we bind data to any databound control (GridView, DropDownList etc) in asp.net.
Modify the code in the code-behind file as shown below. The code is commented and self explanatory.
using System;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.UI.DataVisualization.Charting;
using System.Web.UI.WebControls;
namespace ChartsDemo
{
public partial class
WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{
if (!IsPostBack)
{
GetChartData();
GetChartTypes();
}
}
private void GetChartData()
{
string cs = ConfigurationManager.ConnectionStrings["CS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
// Query to retrieve
database data for the chart control
SqlCommand cmd = new SqlCommand
("Select
StudentName, TotalMarks from Students", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
// Specify the column
name that contains values for X-Axis
Chart1.Series["Series1"].XValueMember =
"StudentName";
// Specify the column
name that contains values for Y-Axis
Chart1.Series["Series1"].YValueMembers =
"TotalMarks";
// Set the datasource
Chart1.DataSource = rdr;
// Finally call
DataBind()
Chart1.DataBind();
}
}
private void GetChartTypes()
{
foreach (int chartType in Enum.GetValues(typeof(SeriesChartType)))
{
ListItem li = new ListItem(Enum.GetName(
typeof(SeriesChartType), chartType), chartType.ToString());
DropDownList1.Items.Add(li);
}
}
protected void DropDownList1_SelectedIndexChanged(object
sender, EventArgs e)
{
GetChartData();
this.Chart1.Series["Series1"].ChartType
= (SeriesChartType)Enum.Parse(
typeof(SeriesChartType), DropDownList1.SelectedValue);
}
}
}
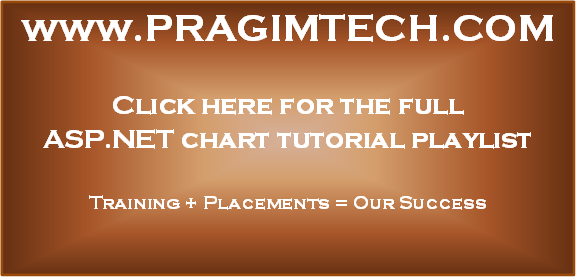
No comments:
Post a Comment
It would be great if you can help share these free resources