Suggested Videos
Part 6 - Stored procedures with output parameters in LINQ to SQL
Part 7 - What is SqlMetal
Part 8 - Lazy loading in LINQ to SQL
In this video we will discuss eager loading in LINQ to SQL. This is continuation to Part 8. Please watch Part 8 before proceeding.
What is Eager loading
Eager loading is the process whereby a query for one type of entity also loads related entities as part of the query.
In LINQ to SQL there are 2 ways we can eager load data
1. Using DataLoadOptions
2. Using Projection
Using DataLoadOptions to eager load related entities in LINQ to SQL :
DataLoadOptions is present in System.Data.Linq namespace
Run the application, and notice that there is only one query which retrieves all the departments and their related employee entities.
Using Projection to eager load related entities in LINQ to SQL :
Again, run the application, and notice that there is only one query which retrieves all the departments and their related employee entities.
Now let's do the same thing with the web application example we worked with in Part 8.
Using DataLoadOptions to eager load related entities in LINQ to SQL :
Using Projection to eager load related entities in LINQ to SQL :
Part 6 - Stored procedures with output parameters in LINQ to SQL
Part 7 - What is SqlMetal
Part 8 - Lazy loading in LINQ to SQL
In this video we will discuss eager loading in LINQ to SQL. This is continuation to Part 8. Please watch Part 8 before proceeding.
What is Eager loading
Eager loading is the process whereby a query for one type of entity also loads related entities as part of the query.
In LINQ to SQL there are 2 ways we can eager load data
1. Using DataLoadOptions
2. Using Projection
Using DataLoadOptions to eager load related entities in LINQ to SQL :
using (SampleDataContext dbContext = new SampleDataContext())
{
dbContext.Log = Console.Out;
// Load related Employee entities along with
the Department entity
DataLoadOptions loadOptions = new DataLoadOptions();
loadOptions.LoadWith<Department>(d
=> d.Employees);
dbContext.LoadOptions = loadOptions;
foreach (Department dept in dbContext.Departments)
{
Console.WriteLine(dept.Name);
foreach (Employee emp in dept.Employees)
{
Console.WriteLine("\t" + emp.FirstName +
" " + emp.LastName);
}
}
}
DataLoadOptions is present in System.Data.Linq namespace
Run the application, and notice that there is only one query which retrieves all the departments and their related employee entities.
Using Projection to eager load related entities in LINQ to SQL :
using (SampleDataContext dbContext = new SampleDataContext())
{
dbContext.Log = Console.Out;
var linqQuery = from dept
in dbContext.Departments
select new { Name = dept.Name,
Employees = dept.Employees };
foreach (var dept
in linqQuery)
{
Console.WriteLine(dept.Name);
foreach (Employee emp in dept.Employees)
{
Console.WriteLine("\t" + emp.FirstName +
" " + emp.LastName);
}
}
}
Again, run the application, and notice that there is only one query which retrieves all the departments and their related employee entities.
Now let's do the same thing with the web application example we worked with in Part 8.
Using DataLoadOptions to eager load related entities in LINQ to SQL :
using (SampleDataContext dbContext = new SampleDataContext())
{
dbContext.Log = Response.Output;
DataLoadOptions loadOptions = new DataLoadOptions();
loadOptions.LoadWith<Department>(d
=> d.Employees);
dbContext.LoadOptions = loadOptions;
gvDepartments.DataSource = dbContext.Departments;
gvDepartments.DataBind();
}
Using Projection to eager load related entities in LINQ to SQL :
using (SampleDataContext dbContext = new SampleDataContext())
{
dbContext.Log = Response.Output;
gvDepartments.DataSource = from dept in
dbContext.Departments
select new { Name = dept.Name,
Employees = dept.Employees };
gvDepartments.DataBind();
}
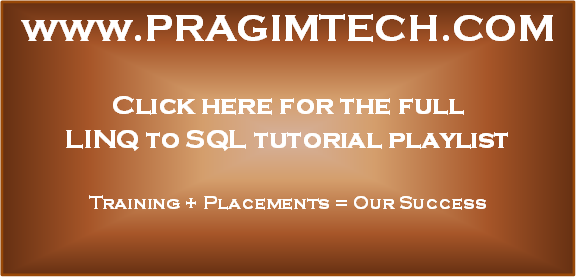
No comments:
Post a Comment
It would be great if you can help share these free resources