Suggested Videos
Part 22 - Configure WCF service endpoint dynamically in code
Part 23 - Hosting WCF service
Part 24 - Self hosting a wcf service in console application
In this video, we will discuss hosting a wcf service using a windows forms application. Hosting a wcf service in windows forms application is very similar to hosting it in a console application. We discussed hosting a wcf service in a console application in Part 24. We will continue with the same example we worked with in Part 24.
Here are the steps to host the wcf service in a windows forms application.
Step 1: Right click on HelloService solution in solution explorer, and add new windows forms application with name = WindowsHost
Step 2: Right click on WindowsHost project in solution explorer, and add the Application Configuration file. This should add App.config file.
Step 3: Copy and paste the following configuration in App.config file
Step 4: Right click on the "References" folder under "WindowsHost" project and add a reference to System.ServiceModel assembly and HelloService project.
Step 5: Drag and drop 2 buttons and a label control on Form1.cs.
Set the following properties of button1
Name = btnStart
Text = Start
Set the following properties of button2
Name = btnStop
Text = Stop
Set the following properties of label1
Name = lblMessage
Text =
At this point Form1.cs should look as shown below.
Step 6: Double click on "Start" and "Stop" buttons to generate their respective "Click" event handlers.
Step 7: Generate "FormClosed" event handler method for Form1. To do this
a) Right click on Form1 and select "Properties" from the context menu
b) Click on "Events" icon in the properties window
c) Double click on FormClosed event
Step 8: Right click on Form1 and select "View Code" from the context menu. Copy and paste the following code
using System;
using System.Windows.Forms;
using System.ServiceModel;
namespace WindowsHost
{
public partial class Form1 : Form
{
private ServiceHost host;
public Form1()
{
InitializeComponent();
host = new ServiceHost(typeof(HelloService.HelloService));
host.Open();
btnStart.Enabled = false;
btnStop.Enabled = true;
lblMessage.Text = "Service Started";
}
private void btnStart_Click(object sender, EventArgs e)
{
host = new ServiceHost(typeof(HelloService.HelloService));
host.Open();
btnStart.Enabled = false;
btnStop.Enabled = true;
lblMessage.Text = "Service Started";
}
private void btnStop_Click(object sender, EventArgs e)
{
host.Close();
btnStart.Enabled = true;
btnStop.Enabled = false;
lblMessage.Text = "Service Stopped";
}
private void Form1_FormClosed(object sender, FormClosedEventArgs e)
{
host.Close();
btnStart.Enabled = true;
btnStop.Enabled = false;
}
}
}
Step 9: Right click on "WindowsHost" project in solution explorer and set it as startup project.
Step 10: Run the host by pressing CTRL + F5. At this point, the wcf service is up and running.
Step 11: Change the code in Form1.cs of WindowsClient project as shown below.
using System;
using System.Windows.Forms;
namespace WindowsClient
{
public partial class Form1 : Form
{
HelloService.HelloServiceClient client;
public Form1()
{
InitializeComponent();
client = new HelloService.HelloServiceClient();
}
private void button1_Click(object sender, EventArgs e)
{
try
{
if (client.State == System.ServiceModel.CommunicationState.Faulted)
client = new HelloService.HelloServiceClient();
label2.Text = client.GetMessage(textBox1.Text);
}
catch (Exception ex)
{
label2.Text = ex.Message;
}
}
}
}
The advantages and disadvantages of hosting a WCF service in a windows application is the same as hosting the wcf service in a Console application.
Part 22 - Configure WCF service endpoint dynamically in code
Part 23 - Hosting WCF service
Part 24 - Self hosting a wcf service in console application
In this video, we will discuss hosting a wcf service using a windows forms application. Hosting a wcf service in windows forms application is very similar to hosting it in a console application. We discussed hosting a wcf service in a console application in Part 24. We will continue with the same example we worked with in Part 24.
Here are the steps to host the wcf service in a windows forms application.
Step 1: Right click on HelloService solution in solution explorer, and add new windows forms application with name = WindowsHost
Step 2: Right click on WindowsHost project in solution explorer, and add the Application Configuration file. This should add App.config file.
Step 3: Copy and paste the following configuration in App.config file
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="mexBehavior">
<serviceMetadata httpGetEnabled="true" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service behaviorConfiguration="mexBehavior"
name="HelloService.HelloService">
<endpoint address="HelloService" binding="netTcpBinding"
contract="HelloService.IHelloService" />
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080" />
<add baseAddress="net.tcp://localhost:8090" />
</baseAddresses>
</host>
</service>
</services>
</system.serviceModel>
</configuration>
Step 4: Right click on the "References" folder under "WindowsHost" project and add a reference to System.ServiceModel assembly and HelloService project.
Step 5: Drag and drop 2 buttons and a label control on Form1.cs.
Set the following properties of button1
Name = btnStart
Text = Start
Set the following properties of button2
Name = btnStop
Text = Stop
Set the following properties of label1
Name = lblMessage
Text =
At this point Form1.cs should look as shown below.

Step 6: Double click on "Start" and "Stop" buttons to generate their respective "Click" event handlers.
Step 7: Generate "FormClosed" event handler method for Form1. To do this
a) Right click on Form1 and select "Properties" from the context menu
b) Click on "Events" icon in the properties window
c) Double click on FormClosed event
Step 8: Right click on Form1 and select "View Code" from the context menu. Copy and paste the following code
using System;
using System.Windows.Forms;
using System.ServiceModel;
namespace WindowsHost
{
public partial class Form1 : Form
{
private ServiceHost host;
public Form1()
{
InitializeComponent();
host = new ServiceHost(typeof(HelloService.HelloService));
host.Open();
btnStart.Enabled = false;
btnStop.Enabled = true;
lblMessage.Text = "Service Started";
}
private void btnStart_Click(object sender, EventArgs e)
{
host = new ServiceHost(typeof(HelloService.HelloService));
host.Open();
btnStart.Enabled = false;
btnStop.Enabled = true;
lblMessage.Text = "Service Started";
}
private void btnStop_Click(object sender, EventArgs e)
{
host.Close();
btnStart.Enabled = true;
btnStop.Enabled = false;
lblMessage.Text = "Service Stopped";
}
private void Form1_FormClosed(object sender, FormClosedEventArgs e)
{
host.Close();
btnStart.Enabled = true;
btnStop.Enabled = false;
}
}
}
Step 9: Right click on "WindowsHost" project in solution explorer and set it as startup project.
Step 10: Run the host by pressing CTRL + F5. At this point, the wcf service is up and running.
Step 11: Change the code in Form1.cs of WindowsClient project as shown below.
using System;
using System.Windows.Forms;
namespace WindowsClient
{
public partial class Form1 : Form
{
HelloService.HelloServiceClient client;
public Form1()
{
InitializeComponent();
client = new HelloService.HelloServiceClient();
}
private void button1_Click(object sender, EventArgs e)
{
try
{
if (client.State == System.ServiceModel.CommunicationState.Faulted)
client = new HelloService.HelloServiceClient();
label2.Text = client.GetMessage(textBox1.Text);
}
catch (Exception ex)
{
label2.Text = ex.Message;
}
}
}
}
The advantages and disadvantages of hosting a WCF service in a windows application is the same as hosting the wcf service in a Console application.
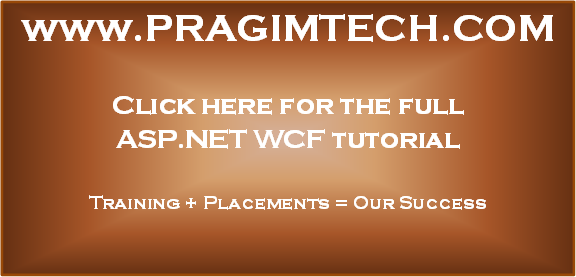
No comments:
Post a Comment
It would be great if you can help share these free resources