Suggested Videos
Part 19 - Creating and throwing strongly typed SOAP faults
Part 20 - Centralize exception handling in WCF
Part 21 - Bindings in WCF
We can configure an endpoint for WCF service in 2 ways
1. Declaratively using the configuration file
2. Dynamically in code
First let's look at configuring an endpoint for the HelloService declaratively using the config file.
The above configuration
1. Configures HelloService to be available at the address net.tcp://localhost:8090/HelloService
2. Makes use of netTcpBinding
3. Configures Service Behavior to enable metadata exchange for clients to generate proxy classes
Now let's look at adding the service endpoint dynamically in code. Here are the steps
Step 1: First delete the sections from the config file that are highlighted with yellow colour.
Step 2: In the Main() method in Program.cs file(in the Host project), include the lines highlighted with yellow color.
public static void Main()
{
using (System.ServiceModel.ServiceHost host = new
System.ServiceModel.ServiceHost(typeof(HelloService.HelloService)))
{
host.Description.Behaviors.Add(new ServiceMetadataBehavior { HttpGetEnabled = true });
host.AddServiceEndpoint(typeof(HelloService.IHelloService),
new NetTcpBinding(), "HelloService");
host.Open();
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
Please make sure to include the following using declarations in Program.cs file.
using System;
using System.ServiceModel;
using System.ServiceModel.Description;
Part 19 - Creating and throwing strongly typed SOAP faults
Part 20 - Centralize exception handling in WCF
Part 21 - Bindings in WCF
We can configure an endpoint for WCF service in 2 ways
1. Declaratively using the configuration file
2. Dynamically in code
First let's look at configuring an endpoint for the HelloService declaratively using the config file.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="mexBehaviour">
<serviceMetadata httpGetEnabled="true" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service name="HelloService.HelloService" behaviorConfiguration="mexBehaviour">
<endpoint address="HelloService" binding="netTcpBinding" contract="HelloService.IHelloService">
</endpoint>
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080/" />
<add baseAddress="net.tcp://localhost:8090"/>
</baseAddresses>
</host>
</service>
</services>
</system.serviceModel>
</configuration>
The above configuration
1. Configures HelloService to be available at the address net.tcp://localhost:8090/HelloService
2. Makes use of netTcpBinding
3. Configures Service Behavior to enable metadata exchange for clients to generate proxy classes
Now let's look at adding the service endpoint dynamically in code. Here are the steps
Step 1: First delete the sections from the config file that are highlighted with yellow colour.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="mexBehaviour">
<serviceMetadata httpGetEnabled="true" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service name="HelloService.HelloService" behaviorConfiguration="mexBehaviour">
<endpoint address="HelloService" binding="netTcpBinding" contract="HelloService.IHelloService">
</endpoint>
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080/" />
<add baseAddress="net.tcp://localhost:8090"/>
</baseAddresses>
</host>
</service>
</services>
</system.serviceModel>
</configuration>
Step 2: In the Main() method in Program.cs file(in the Host project), include the lines highlighted with yellow color.
public static void Main()
{
using (System.ServiceModel.ServiceHost host = new
System.ServiceModel.ServiceHost(typeof(HelloService.HelloService)))
{
host.Description.Behaviors.Add(new ServiceMetadataBehavior { HttpGetEnabled = true });
host.AddServiceEndpoint(typeof(HelloService.IHelloService),
new NetTcpBinding(), "HelloService");
host.Open();
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
Please make sure to include the following using declarations in Program.cs file.
using System;
using System.ServiceModel;
using System.ServiceModel.Description;
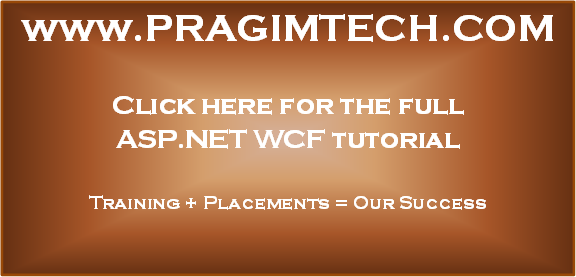
Hi,
ReplyDeleteYour videos have been very helpful! I’m kind of lost with being able to dynamically set the WCF service endpoint address on the client app. Did I miss it in one of your other videos? If not, can you provide some assistance?
Thank you Sir.