Suggested Videos
Part 16 - SOAP faults in WCF
Part 17 - Unhandled exceptions in WCF
Part 18 - Throwing fault exceptions from a WCF service
This is continuation to Part 18, please watch Part 18 before proceeding.
In Part 18, we discussed throwing a generic SOAP fault using FaultException class. Instead of throwing a generic SOAP fault, we can create strongly typed SOAP faults and throw them. Creating our own strongly typed SOAP faults allow us to include any additional custom information about the exception that has occurred. Here are the steps to create a strongly typed SOAP fault.
Step 1: Riglt click on the CalcualtorService and add a class file with name = DivideByZeroFault.cs. Copy and paste the following code. DivideByZeroFault is a strongly typed fault.
using System.Runtime.Serialization;
namespace CalculatorService
{
[DataContract]
public class DivideByZeroFault
{
[DataMember]
public string Error { get; set; }
[DataMember]
public string Details { get; set; }
}
}
Step 2: Make the following changes to ICalculatorService.cs. Notice the change highlighted in yellow. We have used FaultContract attribute to specify that the Divide() method can throw DivideByZeroFault.
using System.ServiceModel;
namespace CalculatorService
{
[ServiceContract]
public interface ICalculatorService
{
[FaultContract(typeof(DivideByZeroFault))]
[OperationContract]
int Divide(int Numerator, int Denominator);
}
}
Step 3: Copy and paste the following code in CalculatorService.cs. Notice that instead of throwing a generic SOAP fault, we are throwing a strongly typed SOAP fault using FaultException<T> class, where <T> is DivideByZeroFault.
using System.ServiceModel;
using System;
namespace CalculatorService
{
public class CalculatorService : ICalculatorService
{
public int Divide(int Numerator, int Denominator)
{
try
{
return Numerator / Denominator;
}
catch (DivideByZeroException ex)
{
DivideByZeroFault divideByZeroFault = new DivideByZeroFault();
divideByZeroFault.Error = ex.Message;
divideByZeroFault.Details = "Denominator cannot be ZERO";
throw new FaultException<DivideByZeroFault>(divideByZeroFault);
}
}
}
}
As we have changed the WCF service, update the service reference in the client application.
Step 4: Finally in the client application, modify the catch block as shown below.
catch (FaultException<CalculatorService.DivideByZeroFault> faultException)
{
label1.Text = faultException.Detail.Error + " - " + faultException.Detail.Details;
}
So in short, to create a strongly typed SOAP fault
1. Create a class that represents your SOAP fault. Decorate the class with DataContract attribute and the properties with DataMember attribute.
2. In the service data contract, use FaultContractAttribute to specify which operations can throw which SOAP faults.
3. In the service implementation create an instance of the strongly typed SOAP fault and throw it using FaultException<T>.
Part 16 - SOAP faults in WCF
Part 17 - Unhandled exceptions in WCF
Part 18 - Throwing fault exceptions from a WCF service
This is continuation to Part 18, please watch Part 18 before proceeding.
In Part 18, we discussed throwing a generic SOAP fault using FaultException class. Instead of throwing a generic SOAP fault, we can create strongly typed SOAP faults and throw them. Creating our own strongly typed SOAP faults allow us to include any additional custom information about the exception that has occurred. Here are the steps to create a strongly typed SOAP fault.
Step 1: Riglt click on the CalcualtorService and add a class file with name = DivideByZeroFault.cs. Copy and paste the following code. DivideByZeroFault is a strongly typed fault.
using System.Runtime.Serialization;
namespace CalculatorService
{
[DataContract]
public class DivideByZeroFault
{
[DataMember]
public string Error { get; set; }
[DataMember]
public string Details { get; set; }
}
}
Step 2: Make the following changes to ICalculatorService.cs. Notice the change highlighted in yellow. We have used FaultContract attribute to specify that the Divide() method can throw DivideByZeroFault.
using System.ServiceModel;
namespace CalculatorService
{
[ServiceContract]
public interface ICalculatorService
{
[FaultContract(typeof(DivideByZeroFault))]
[OperationContract]
int Divide(int Numerator, int Denominator);
}
}
Step 3: Copy and paste the following code in CalculatorService.cs. Notice that instead of throwing a generic SOAP fault, we are throwing a strongly typed SOAP fault using FaultException<T> class, where <T> is DivideByZeroFault.
using System.ServiceModel;
using System;
namespace CalculatorService
{
public class CalculatorService : ICalculatorService
{
public int Divide(int Numerator, int Denominator)
{
try
{
return Numerator / Denominator;
}
catch (DivideByZeroException ex)
{
DivideByZeroFault divideByZeroFault = new DivideByZeroFault();
divideByZeroFault.Error = ex.Message;
divideByZeroFault.Details = "Denominator cannot be ZERO";
throw new FaultException<DivideByZeroFault>(divideByZeroFault);
}
}
}
}
As we have changed the WCF service, update the service reference in the client application.
Step 4: Finally in the client application, modify the catch block as shown below.
catch (FaultException<CalculatorService.DivideByZeroFault> faultException)
{
label1.Text = faultException.Detail.Error + " - " + faultException.Detail.Details;
}
So in short, to create a strongly typed SOAP fault
1. Create a class that represents your SOAP fault. Decorate the class with DataContract attribute and the properties with DataMember attribute.
2. In the service data contract, use FaultContractAttribute to specify which operations can throw which SOAP faults.
3. In the service implementation create an instance of the strongly typed SOAP fault and throw it using FaultException<T>.
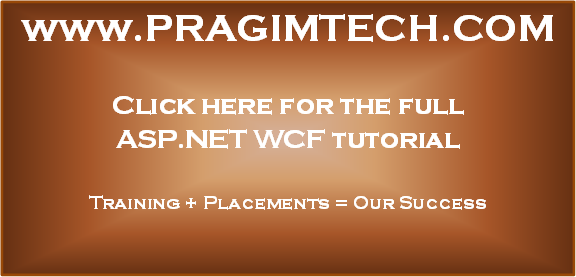
I receive error stating, "The creator of this fault did not specify a Reason". Should I add FaultReason parameter to the FaultException method? Even after adding it, I still get an error as, "FaultException`1 was unhandled by user code".
ReplyDelete
Deletetry this one will help you
catch (FaultException ex)
{
DivideByZeroFault lDivideByZeroFault = new DivideByZeroFault();
lDivideByZeroFault.Error = ex.Message;
lDivideByZeroFault.Details = "Denominator cannot be ZERO";
throw new FaultException(lDivideByZeroFault);
}