Suggested Videos
Part 21 - Bindings in WCF
Part 22 - Configure WCF service endpoint dynamically in code
Part 23 - Hosting WCF service
In this video, we will discuss hosting a wcf service using a console application. Hosting a wcf service in any managed .net application is called as self hosting. Console applications, WPF applications, WinForms applications are all examples of managed .net applications.
Here are the steps to create and slef host a wcf service in a console application
Step 1: Create a new class library project with name = HelloService
Step 2: Delete class1.cs file that is auto-generated and a new WCF service with name = HelloService.
Step 3: Copy and paste the following code in IHelloService.cs file
using System.ServiceModel;
namespace HelloService
{
[ServiceContract]
public interface IHelloService
{
[OperationContract]
string GetMessage(string name);
}
}
Step 4: Copy and paste the following code in HelloService.cs file
namespace HelloService
{
public class HelloService : IHelloService
{
public string GetMessage(string name)
{
return "Hello " + name;
}
}
}
Step 5: To host the WCF service, right click on HelloService solution in Solution explorer and add new Console Application with name = Host
Step 6: Right click on the "References" folder under "Host" project and add a reference to System.ServiceModel assembly and HelloService project.
Step 7: Copy and paste the following code in Program.cs file
using System;
using System.ServiceModel;
namespace Host
{
class Program
{
public static void Main()
{
using (ServiceHost host = new ServiceHost(typeof(HelloService.HelloService)))
{
host.Open();
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
}
}
Step 8: Right click on "Host" project and add Application Configuration file. Copy and paste the following code in App.config file.
Step 9: Right click on "Host" project in solution explorer and set it as startup project.
Step 10: Run the host by pressing CTRL + F5.
Step 11: Create a new windows forms application with name = WindowsClient
Step 12: Design the wia hreform as shown in the image below
Part 21 - Bindings in WCF
Part 22 - Configure WCF service endpoint dynamically in code
Part 23 - Hosting WCF service
In this video, we will discuss hosting a wcf service using a console application. Hosting a wcf service in any managed .net application is called as self hosting. Console applications, WPF applications, WinForms applications are all examples of managed .net applications.
Here are the steps to create and slef host a wcf service in a console application
Step 1: Create a new class library project with name = HelloService
Step 2: Delete class1.cs file that is auto-generated and a new WCF service with name = HelloService.
Step 3: Copy and paste the following code in IHelloService.cs file
using System.ServiceModel;
namespace HelloService
{
[ServiceContract]
public interface IHelloService
{
[OperationContract]
string GetMessage(string name);
}
}
Step 4: Copy and paste the following code in HelloService.cs file
namespace HelloService
{
public class HelloService : IHelloService
{
public string GetMessage(string name)
{
return "Hello " + name;
}
}
}
Step 5: To host the WCF service, right click on HelloService solution in Solution explorer and add new Console Application with name = Host
Step 6: Right click on the "References" folder under "Host" project and add a reference to System.ServiceModel assembly and HelloService project.
Step 7: Copy and paste the following code in Program.cs file
using System;
using System.ServiceModel;
namespace Host
{
class Program
{
public static void Main()
{
using (ServiceHost host = new ServiceHost(typeof(HelloService.HelloService)))
{
host.Open();
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
}
}
Step 8: Right click on "Host" project and add Application Configuration file. Copy and paste the following code in App.config file.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="mexBehavior">
<serviceMetadata httpGetEnabled="true" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service behaviorConfiguration="mexBehavior"
name="HelloService.HelloService">
<endpoint address="HelloService" binding="netTcpBinding"
contract="HelloService.IHelloService" />
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080"/>
<add baseAddress="net.tcp://localhost:8090"/>
</baseAddresses>
</host>
</service>
</services>
</system.serviceModel>
</configuration>
Step 9: Right click on "Host" project in solution explorer and set it as startup project.
Step 10: Run the host by pressing CTRL + F5.
Step 11: Create a new windows forms application with name = WindowsClient
Step 12: Design the wia hreform as shown in the image below

Step 13: Right click on "References" folder in WindowsClient project and select add service reference option. Type "http://localhost:8080/" in the address textbox and click "Go" button. Type "HelloService" in the "Namespace" textbox and click "OK" button.
Step 14: Double click on the button control on the windows form to generate button Click event handler. Copy and paste the following code in Form1.cs file.
using System;
using System.Windows.Forms;
namespace WindowsClient
{
public partial class Form1 : Form
{
HelloService.HelloServiceClient client;
public Form1()
{
InitializeComponent();
client = new HelloService.HelloServiceClient();
}
private void button1_Click(object sender, EventArgs e)
{
label2.Text = client.GetMessage(textBox1.Text);
}
}
}
Advantages of self hosting a wcf service in a console application
1. Very easy to setup. Specify the configuration in app.config file and with a few lines of code we have the service up and running.
2. Easy to debug as we don't have to attach a separate process that hosts the wcf service.
3. Supports all bindings and transport protocols.
4. Very flexible to control the lifetime of the services through the Open() and Close() methods of ServiceHost.
Disadvantages of self hosting a wcf service in a console application
1. The service is available for the clients only when the service host is running.
2. Self hosting does not support automatic message based activation that we get when hosted within IIS.
3. Custom code required.
In general, self-hosting is only suitable during the development and demonstration phase and not for hosting live wcf services.
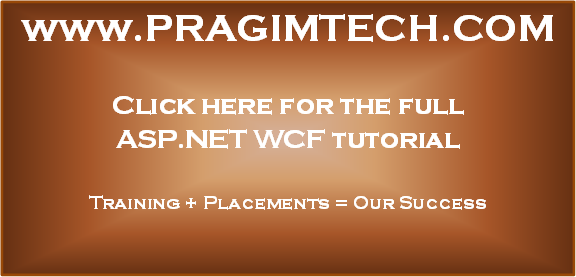
Its very nice to see these precious videos.
ReplyDeleteVenkat, I have doubt regarding WCF service authorization.
it would be appreciable if u can post some related doc/vid.
Can you give more data as to why you say this?
ReplyDelete"In general, self-hosting is only suitable during the development and demonstration phase and not for hosting live wcf services."
Please can you post on Universal Contract consumed with console application client side?
ReplyDeleteThese instructions are beautifully clear. Actually easier to follow than the original video which was enormously complicated by talking us through the intricacies of the App.config editor. I built the app twice - once from the video and once from these instructions. Unfortunately, both times I was unable to proceed. The app throws an exception at host.Open() in Program.cs telling me:
ReplyDelete"Unhandled Exception: System.ServiceModel.AddressAccessDeniedException: HTTP could not register URL http://+:8080/. Your process does not have access rights to this namespace"
Actually, I got slightly further building from these instructions, as upon building my Windows Defender firewall asked if I wanted to allow the app access. I said yes, of course. But the only consequence was that now, instead of breaking in the code itself, the exception gets printed out on screen.
I am probably years too late (It is now May 2020) to get any help on this - but, you never know.
In any case, thank you for the great tutorials. I am learning a lot.
Run application with admin rights.
Deleteclient.GetMessage(textBox1.Text) telling me 'System.ServiceModel.Security.SecurityNegotiationException' occurred in mscorlib.dll
ReplyDeleteAdditional information: A call to SSPI failed, see inner exception. any help is appreciated.