Suggested Videos
Part 39 - Working with detailsview without using datasource controls
Part 40 - Detailsview insert update delete using sqldatasource control
Part 41 - Detailsview insert update delete using objectdatasource control
In this video we will discuss about inserting, updating and deleting data from detailsview without using any datasource control.
When the webform is loaded, the gridview control, should retrieve and display all the rows from tblEmployee table. As soon as I select a row, in the gridview control, then all the columns of the selected row, should be displayed in details view control. Then, we should be able to edit, delete and add a new employee using the DetailsView control, as shown in the image below.
We will be using tblEmployee table for this demo. If you need sql script to create this table, please refer to Part 37 by clicking here.
We implemented EmployeeDataAccessLayer with methods to Select, Insert, Update and Delete data in Part 41. We will be using EmployeeDataAccessLayer for this demo, if you need the code, please refer to Part 41 by clicking here.
Step 1: Drag and drop "GridView" control on WebForm1.aspx
Step 2: On GridView1, set
DataKeyNames=Id
AutoGenerateSelectButton="true"
Step 3: Generate event handler method for SelectedIndexChanged event of GridView1 control
Step 4: Drag and drop "DetailsView" control on WebForm1.aspx
Step 5: On DetailsView1, set
DataKeyNames=Id
AutoGenerateRows="False"
AutoGenerateSelectButton="True"
AutoGenerateDeleteButton="True"
AutoGenerateEditButton="True"
AutoGenerateInsertButton="True"
Setp 6: Add 10 BoundField's and set DataField and HeaderText accordingly.
Setp 7: Set ReadOnly="True" and InsertVisible="False" on the bound field that display, Id column value.
Step 8: Generate event handler method for the following events of DetailsView1 control.
ModeChanging
ItemInserting
ItemDeleting
ItemUpdating
Step 9: Copy and paste the following code in webform1.aspx.cs file
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridViewDataBind();
}
}
private void GridViewDataBind()
{
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployeesBasicDetails();
GridView1.DataBind();
}
protected void GridView1_SelectedIndexChanged(object sender, EventArgs e)
{
DetailsViewDataBind();
}
private void DetailsViewDataBind()
{
if (GridView1.SelectedDataKey != null)
{
DetailsView1.Visible = true;
List<Employee> listEmployee = new List<Employee>();
listEmployee.Add(EmployeeDataAccessLayer.GetEmployeesFullDetailsById((int)GridView1.SelectedDataKey.Value));
DetailsView1.DataSource = listEmployee;
DetailsView1.DataBind();
}
else
{
DetailsView1.Visible = false;
}
}
protected void DetailsView1_ModeChanging(object sender, DetailsViewModeEventArgs e)
{
DetailsView1.ChangeMode(e.NewMode);
DetailsViewDataBind();
}
protected void DetailsView1_ItemInserting(object sender, DetailsViewInsertEventArgs e)
{
EmployeeDataAccessLayer.InsertEmployee(e.Values["FirstName"].ToString(),
e.Values["LastName"].ToString(), e.Values["City"].ToString(),
e.Values["Gender"].ToString(), Convert.ToDateTime(e.Values["DateOfBirth"]),
e.Values["Country"].ToString(), Convert.ToInt32(e.Values["Salary"]),
Convert.ToDateTime(e.Values["DateOfJoining"]), e.Values["MaritalStatus"].ToString());
DetailsView1.ChangeMode(DetailsViewMode.ReadOnly);
GridViewDataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemDeleting(object sender, DetailsViewDeleteEventArgs e)
{
EmployeeDataAccessLayer.DeleteEmployee((int)GridView1.SelectedDataKey.Value);
GridViewDataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemUpdating(object sender, DetailsViewUpdateEventArgs e)
{
int employeeId = (int)GridView1.SelectedDataKey.Value;
EmployeeDataAccessLayer.UpdateEmployee(employeeId,
e.NewValues["FirstName"].ToString(), e.NewValues["LastName"].ToString(),
e.NewValues["City"].ToString(), e.NewValues["Gender"].ToString(),
Convert.ToDateTime(e.NewValues["DateOfBirth"]), e.NewValues["Country"].ToString(),
Convert.ToInt32(e.NewValues["Salary"]), Convert.ToDateTime(e.NewValues["DateOfJoining"]),
e.NewValues["MaritalStatus"].ToString());
DetailsView1.ChangeMode(DetailsViewMode.ReadOnly);
GridViewDataBind();
GridView1.SelectRow(-1);
}
Part 39 - Working with detailsview without using datasource controls
Part 40 - Detailsview insert update delete using sqldatasource control
Part 41 - Detailsview insert update delete using objectdatasource control
In this video we will discuss about inserting, updating and deleting data from detailsview without using any datasource control.
When the webform is loaded, the gridview control, should retrieve and display all the rows from tblEmployee table. As soon as I select a row, in the gridview control, then all the columns of the selected row, should be displayed in details view control. Then, we should be able to edit, delete and add a new employee using the DetailsView control, as shown in the image below.
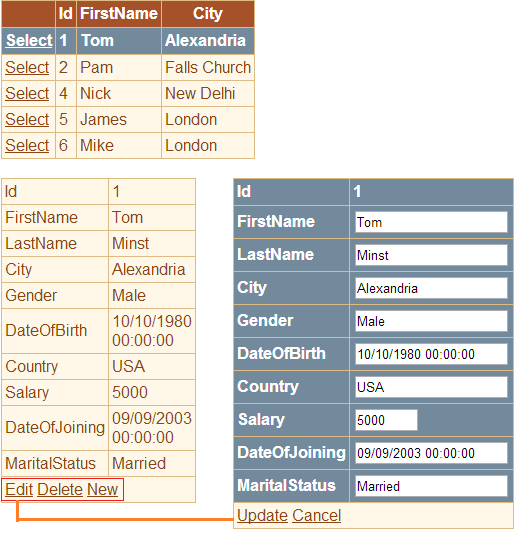
We will be using tblEmployee table for this demo. If you need sql script to create this table, please refer to Part 37 by clicking here.
We implemented EmployeeDataAccessLayer with methods to Select, Insert, Update and Delete data in Part 41. We will be using EmployeeDataAccessLayer for this demo, if you need the code, please refer to Part 41 by clicking here.
Step 1: Drag and drop "GridView" control on WebForm1.aspx
Step 2: On GridView1, set
DataKeyNames=Id
AutoGenerateSelectButton="true"
Step 3: Generate event handler method for SelectedIndexChanged event of GridView1 control
Step 4: Drag and drop "DetailsView" control on WebForm1.aspx
Step 5: On DetailsView1, set
DataKeyNames=Id
AutoGenerateRows="False"
AutoGenerateSelectButton="True"
AutoGenerateDeleteButton="True"
AutoGenerateEditButton="True"
AutoGenerateInsertButton="True"
Setp 6: Add 10 BoundField's and set DataField and HeaderText accordingly.
Setp 7: Set ReadOnly="True" and InsertVisible="False" on the bound field that display, Id column value.
Step 8: Generate event handler method for the following events of DetailsView1 control.
ModeChanging
ItemInserting
ItemDeleting
ItemUpdating
Step 9: Copy and paste the following code in webform1.aspx.cs file
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridViewDataBind();
}
}
private void GridViewDataBind()
{
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployeesBasicDetails();
GridView1.DataBind();
}
protected void GridView1_SelectedIndexChanged(object sender, EventArgs e)
{
DetailsViewDataBind();
}
private void DetailsViewDataBind()
{
if (GridView1.SelectedDataKey != null)
{
DetailsView1.Visible = true;
List<Employee> listEmployee = new List<Employee>();
listEmployee.Add(EmployeeDataAccessLayer.GetEmployeesFullDetailsById((int)GridView1.SelectedDataKey.Value));
DetailsView1.DataSource = listEmployee;
DetailsView1.DataBind();
}
else
{
DetailsView1.Visible = false;
}
}
protected void DetailsView1_ModeChanging(object sender, DetailsViewModeEventArgs e)
{
DetailsView1.ChangeMode(e.NewMode);
DetailsViewDataBind();
}
protected void DetailsView1_ItemInserting(object sender, DetailsViewInsertEventArgs e)
{
EmployeeDataAccessLayer.InsertEmployee(e.Values["FirstName"].ToString(),
e.Values["LastName"].ToString(), e.Values["City"].ToString(),
e.Values["Gender"].ToString(), Convert.ToDateTime(e.Values["DateOfBirth"]),
e.Values["Country"].ToString(), Convert.ToInt32(e.Values["Salary"]),
Convert.ToDateTime(e.Values["DateOfJoining"]), e.Values["MaritalStatus"].ToString());
DetailsView1.ChangeMode(DetailsViewMode.ReadOnly);
GridViewDataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemDeleting(object sender, DetailsViewDeleteEventArgs e)
{
EmployeeDataAccessLayer.DeleteEmployee((int)GridView1.SelectedDataKey.Value);
GridViewDataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemUpdating(object sender, DetailsViewUpdateEventArgs e)
{
int employeeId = (int)GridView1.SelectedDataKey.Value;
EmployeeDataAccessLayer.UpdateEmployee(employeeId,
e.NewValues["FirstName"].ToString(), e.NewValues["LastName"].ToString(),
e.NewValues["City"].ToString(), e.NewValues["Gender"].ToString(),
Convert.ToDateTime(e.NewValues["DateOfBirth"]), e.NewValues["Country"].ToString(),
Convert.ToInt32(e.NewValues["Salary"]), Convert.ToDateTime(e.NewValues["DateOfJoining"]),
e.NewValues["MaritalStatus"].ToString());
DetailsView1.ChangeMode(DetailsViewMode.ReadOnly);
GridViewDataBind();
GridView1.SelectRow(-1);
}
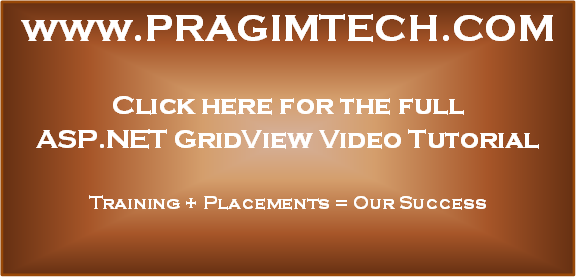
No comments:
Post a Comment
It would be great if you can help share these free resources