Suggested Videos
Part 38 - Using objectdatasource control with detailsview
Part 39 - Working with detailsview without using datasource controls
Part 40 - asp.net detailsview insert update delete using sqldatasource control
In this video we will discuss about inserting, updating and deleting data using detailsview and objectdatasource control.
When the webform is loaded, the gridview control, should retrieve and display all the rows from tblEmployee table. As soon as I select a row, in the gridview control, then all the columns of the selected row, should be displayed in details view control. Then, we should be able to edit, delete and add a new employee using the DetailsView control, as shown in the image below.
We will be using tblEmployee table for this demo. If you need sql script to create this table, please refer to Part 37 by clicking here.
Step 1: Drag and drop a gridview control, a detailsview control and 2 objectdatasource controls on webform1.aspx.
Step 2: Add a class file, with name = EmployeeDataAccessLayer.cs to your project. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
namespace Demo
{
public class EmployeeBasic
{
public int Id { get; set; }
public string FirstName { get; set; }
public string City { get; set; }
}
public class Employee : EmployeeBasic
{
public string LastName { get; set; }
public string Gender { get; set; }
public DateTime DateOfBirth { get; set; }
public string Country { get; set; }
public int Salary { get; set; }
public DateTime DateOfJoining { get; set; }
public string MaritalStatus { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<EmployeeBasic> GetAllEmployeesBasicDetails()
{
List<EmployeeBasic> listEmployees = new List<EmployeeBasic>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select Id, FirstName, City from tblEmployee", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
EmployeeBasic employeeBasic = new EmployeeBasic();
employeeBasic.Id = Convert.ToInt32(rdr["Id"]);
employeeBasic.FirstName = rdr["FirstName"].ToString();
employeeBasic.City = rdr["City"].ToString();
listEmployees.Add(employeeBasic);
}
}
return listEmployees;
}
public static Employee GetEmployeesFullDetailsById(int Id)
{
Employee employee = new Employee();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select * from tblEmployee where Id = @Id", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@Id";
parameter.Value = Id;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
employee.Id = Convert.ToInt32(rdr["Id"]);
employee.FirstName = rdr["FirstName"].ToString();
employee.City = rdr["City"].ToString();
employee.LastName = rdr["LastName"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.DateOfBirth = Convert.ToDateTime(rdr["DateOfBirth"]);
employee.Country = rdr["Country"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
employee.DateOfJoining = Convert.ToDateTime(rdr["DateOfJoining"]);
employee.MaritalStatus = rdr["MaritalStatus"].ToString();
}
}
return employee;
}
public static void InsertEmployee(string firstName, string lastName,
string city, string gender, DateTime dateOfBirth, string country,
int salary, DateTime dateOfJoining, string maritalStatus)
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string insertQuery = "Insert into tblEmployee values (@FirstName, " +
"@LastName, @City, @Gender, @DateOfBirth, @Country, @Salary, " +
"@DateOfJoining, @MaritalStatus)";
SqlCommand cmd = new SqlCommand(insertQuery, con);
#region Parameters
SqlParameter parameter_FirstName = new SqlParameter();
parameter_FirstName.ParameterName = "@FirstName";
parameter_FirstName.Value = firstName;
cmd.Parameters.Add(parameter_FirstName);
SqlParameter parameter_LastName = new SqlParameter();
parameter_LastName.ParameterName = "@LastName";
parameter_LastName.Value = lastName;
cmd.Parameters.Add(parameter_LastName);
SqlParameter parameter_city = new SqlParameter();
parameter_city.ParameterName = "@City";
parameter_city.Value = city;
cmd.Parameters.Add(parameter_city);
SqlParameter parameter_Gender = new SqlParameter();
parameter_Gender.ParameterName = "@Gender";
parameter_Gender.Value = gender;
cmd.Parameters.Add(parameter_Gender);
SqlParameter parameter_DateOfBirth = new SqlParameter();
parameter_DateOfBirth.ParameterName = "@DateOfBirth";
parameter_DateOfBirth.Value = dateOfBirth;
cmd.Parameters.Add(parameter_DateOfBirth);
SqlParameter parameter_Country = new SqlParameter();
parameter_Country.ParameterName = "@Country";
parameter_Country.Value = country;
cmd.Parameters.Add(parameter_Country);
SqlParameter parameter_Salary = new SqlParameter();
parameter_Salary.ParameterName = "@Salary";
parameter_Salary.Value = salary;
cmd.Parameters.Add(parameter_Salary);
SqlParameter parameter_DateOfJoining = new SqlParameter();
parameter_DateOfJoining.ParameterName = "@DateOfJoining";
parameter_DateOfJoining.Value = dateOfJoining;
cmd.Parameters.Add(parameter_DateOfJoining);
SqlParameter parameter_MaritalStatus = new SqlParameter();
parameter_MaritalStatus.ParameterName = "@MaritalStatus";
parameter_MaritalStatus.Value = maritalStatus;
cmd.Parameters.Add(parameter_MaritalStatus);
#endregion
con.Open();
cmd.ExecuteNonQuery();
}
}
public static void UpdateEmployee(int id, string firstName, string lastName,
string city, string gender, DateTime dateOfBirth, string country,
int salary, DateTime dateOfJoining, string maritalStatus)
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string updateQuery = "Update tblEmployee set FirstName = @FirstName, " +
"LastName = @LastName, City = @City, Gender = @Gender, " +
"DateOfBirth = @DateOfBirth, Country = @Country, Salary = @Salary," +
"DateOfJoining = @DateOfJoining, MaritalStatus = @MaritalStatus where Id = @Id";
SqlCommand cmd = new SqlCommand(updateQuery, con);
#region Parameters
SqlParameter parameter_Id = new SqlParameter();
parameter_Id.ParameterName = "@Id";
parameter_Id.Value = id;
cmd.Parameters.Add(parameter_Id);
SqlParameter parameter_FirstName = new SqlParameter();
parameter_FirstName.ParameterName = "@FirstName";
parameter_FirstName.Value = firstName;
cmd.Parameters.Add(parameter_FirstName);
SqlParameter parameter_LastName = new SqlParameter();
parameter_LastName.ParameterName = "@LastName";
parameter_LastName.Value = lastName;
cmd.Parameters.Add(parameter_LastName);
SqlParameter parameter_city = new SqlParameter();
parameter_city.ParameterName = "@City";
parameter_city.Value = city;
cmd.Parameters.Add(parameter_city);
SqlParameter parameter_Gender = new SqlParameter();
parameter_Gender.ParameterName = "@Gender";
parameter_Gender.Value = gender;
cmd.Parameters.Add(parameter_Gender);
SqlParameter parameter_DateOfBirth = new SqlParameter();
parameter_DateOfBirth.ParameterName = "@DateOfBirth";
parameter_DateOfBirth.Value = dateOfBirth;
cmd.Parameters.Add(parameter_DateOfBirth);
SqlParameter parameter_Country = new SqlParameter();
parameter_Country.ParameterName = "@Country";
parameter_Country.Value = country;
cmd.Parameters.Add(parameter_Country);
SqlParameter parameter_Salary = new SqlParameter();
parameter_Salary.ParameterName = "@Salary";
parameter_Salary.Value = salary;
cmd.Parameters.Add(parameter_Salary);
SqlParameter parameter_DateOfJoining = new SqlParameter();
parameter_DateOfJoining.ParameterName = "@DateOfJoining";
parameter_DateOfJoining.Value = dateOfJoining;
cmd.Parameters.Add(parameter_DateOfJoining);
SqlParameter parameter_MaritalStatus = new SqlParameter();
parameter_MaritalStatus.ParameterName = "@MaritalStatus";
parameter_MaritalStatus.Value = maritalStatus;
cmd.Parameters.Add(parameter_MaritalStatus);
#endregion
con.Open();
cmd.ExecuteNonQuery();
}
}
public static void DeleteEmployee(int id)
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("delete from tblEmployee where Id = @Id", con);
SqlParameter parameter_Id = new SqlParameter();
parameter_Id.ParameterName = "@Id";
parameter_Id.Value = id;
cmd.Parameters.Add(parameter_Id);
con.Open();
cmd.ExecuteNonQuery();
}
}
}
}
Step 3: Build your solution, so that the employee data access layer class is compiled.
Step 4: Configure ObjectDataSource1 control to retrieve data from EmployeeDataAccessLayer. Use GetAllEmployeesBasicDetails() as the SELECT method.
Step 5: Asscociate ObjectDataSource1 control with Gridview1 control, and make sure "Enable Selection" checkbox is checked.
Step 6: Configure ObjectDataSource2 control to retrieve data from EmployeeDataAccessLayer. Use GetEmployeesFullDetailsById(int Id) as the SELECT method. The value for the parameter - Id should come from the selected row in GridView1 control. Also, select INSERT, UPDATE and DELETE methods.
INSERT Method - InsertEmployee()
UPDATE Method - UpdateEmployee()
DELETE Method - DeleteEmployee()
Step 7: Associate ObjectDataSource2 with DetailsView1 control and make sure the following checkboxes are selected.
Enable Inserting
Enable Editing
Enable Deleting
Step 8: Generate ItemInserted, ItemUpdated and ItemDeleted event handler methods for DetailsView1 control.
Step 9: Copy and paste the following code in webform1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Page_PreRender(object sender, EventArgs e)
{
if (GridView1.SelectedRow == null)
{
DetailsView1.Visible = false;
}
else
{
DetailsView1.Visible = true;
}
}
protected void DetailsView1_ItemInserted(object sender, DetailsViewInsertedEventArgs e)
{
GridView1.DataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemDeleted(object sender, DetailsViewDeletedEventArgs e)
{
GridView1.DataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemUpdated(object sender, DetailsViewUpdatedEventArgs e)
{
GridView1.DataBind();
GridView1.SelectRow(-1);
}
Step 10: Set GridView1, DataKeyNames to "Id"
DataKeyNames="Id"
Step 11: Run the application. Select a row in gridview, and click "Edit". Notice that Id is also editable. To make it non editable, set ReadOnly attribute to true.
Step 12: Click on "New" link button in detailsview to insert a new row. Notice that, the interface shows a textbox for Id column. This column in the database is identity column, and we don't have to supply a value for it. To make it invisible, set InsertVisible attribute to false.
Setp 13: Click on Edit, change a few values, and click "Update". Notice that none of the rows are updated. To solve this issue, set DataKeyNames="Id" on DetailsView1 control.
Part 38 - Using objectdatasource control with detailsview
Part 39 - Working with detailsview without using datasource controls
Part 40 - asp.net detailsview insert update delete using sqldatasource control
In this video we will discuss about inserting, updating and deleting data using detailsview and objectdatasource control.
When the webform is loaded, the gridview control, should retrieve and display all the rows from tblEmployee table. As soon as I select a row, in the gridview control, then all the columns of the selected row, should be displayed in details view control. Then, we should be able to edit, delete and add a new employee using the DetailsView control, as shown in the image below.
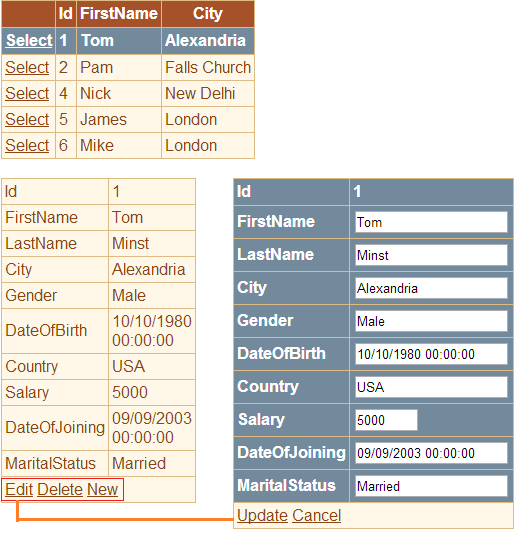
We will be using tblEmployee table for this demo. If you need sql script to create this table, please refer to Part 37 by clicking here.
Step 1: Drag and drop a gridview control, a detailsview control and 2 objectdatasource controls on webform1.aspx.
Step 2: Add a class file, with name = EmployeeDataAccessLayer.cs to your project. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
namespace Demo
{
public class EmployeeBasic
{
public int Id { get; set; }
public string FirstName { get; set; }
public string City { get; set; }
}
public class Employee : EmployeeBasic
{
public string LastName { get; set; }
public string Gender { get; set; }
public DateTime DateOfBirth { get; set; }
public string Country { get; set; }
public int Salary { get; set; }
public DateTime DateOfJoining { get; set; }
public string MaritalStatus { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<EmployeeBasic> GetAllEmployeesBasicDetails()
{
List<EmployeeBasic> listEmployees = new List<EmployeeBasic>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select Id, FirstName, City from tblEmployee", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
EmployeeBasic employeeBasic = new EmployeeBasic();
employeeBasic.Id = Convert.ToInt32(rdr["Id"]);
employeeBasic.FirstName = rdr["FirstName"].ToString();
employeeBasic.City = rdr["City"].ToString();
listEmployees.Add(employeeBasic);
}
}
return listEmployees;
}
public static Employee GetEmployeesFullDetailsById(int Id)
{
Employee employee = new Employee();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select * from tblEmployee where Id = @Id", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@Id";
parameter.Value = Id;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
employee.Id = Convert.ToInt32(rdr["Id"]);
employee.FirstName = rdr["FirstName"].ToString();
employee.City = rdr["City"].ToString();
employee.LastName = rdr["LastName"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.DateOfBirth = Convert.ToDateTime(rdr["DateOfBirth"]);
employee.Country = rdr["Country"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
employee.DateOfJoining = Convert.ToDateTime(rdr["DateOfJoining"]);
employee.MaritalStatus = rdr["MaritalStatus"].ToString();
}
}
return employee;
}
public static void InsertEmployee(string firstName, string lastName,
string city, string gender, DateTime dateOfBirth, string country,
int salary, DateTime dateOfJoining, string maritalStatus)
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string insertQuery = "Insert into tblEmployee values (@FirstName, " +
"@LastName, @City, @Gender, @DateOfBirth, @Country, @Salary, " +
"@DateOfJoining, @MaritalStatus)";
SqlCommand cmd = new SqlCommand(insertQuery, con);
#region Parameters
SqlParameter parameter_FirstName = new SqlParameter();
parameter_FirstName.ParameterName = "@FirstName";
parameter_FirstName.Value = firstName;
cmd.Parameters.Add(parameter_FirstName);
SqlParameter parameter_LastName = new SqlParameter();
parameter_LastName.ParameterName = "@LastName";
parameter_LastName.Value = lastName;
cmd.Parameters.Add(parameter_LastName);
SqlParameter parameter_city = new SqlParameter();
parameter_city.ParameterName = "@City";
parameter_city.Value = city;
cmd.Parameters.Add(parameter_city);
SqlParameter parameter_Gender = new SqlParameter();
parameter_Gender.ParameterName = "@Gender";
parameter_Gender.Value = gender;
cmd.Parameters.Add(parameter_Gender);
SqlParameter parameter_DateOfBirth = new SqlParameter();
parameter_DateOfBirth.ParameterName = "@DateOfBirth";
parameter_DateOfBirth.Value = dateOfBirth;
cmd.Parameters.Add(parameter_DateOfBirth);
SqlParameter parameter_Country = new SqlParameter();
parameter_Country.ParameterName = "@Country";
parameter_Country.Value = country;
cmd.Parameters.Add(parameter_Country);
SqlParameter parameter_Salary = new SqlParameter();
parameter_Salary.ParameterName = "@Salary";
parameter_Salary.Value = salary;
cmd.Parameters.Add(parameter_Salary);
SqlParameter parameter_DateOfJoining = new SqlParameter();
parameter_DateOfJoining.ParameterName = "@DateOfJoining";
parameter_DateOfJoining.Value = dateOfJoining;
cmd.Parameters.Add(parameter_DateOfJoining);
SqlParameter parameter_MaritalStatus = new SqlParameter();
parameter_MaritalStatus.ParameterName = "@MaritalStatus";
parameter_MaritalStatus.Value = maritalStatus;
cmd.Parameters.Add(parameter_MaritalStatus);
#endregion
con.Open();
cmd.ExecuteNonQuery();
}
}
public static void UpdateEmployee(int id, string firstName, string lastName,
string city, string gender, DateTime dateOfBirth, string country,
int salary, DateTime dateOfJoining, string maritalStatus)
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string updateQuery = "Update tblEmployee set FirstName = @FirstName, " +
"LastName = @LastName, City = @City, Gender = @Gender, " +
"DateOfBirth = @DateOfBirth, Country = @Country, Salary = @Salary," +
"DateOfJoining = @DateOfJoining, MaritalStatus = @MaritalStatus where Id = @Id";
SqlCommand cmd = new SqlCommand(updateQuery, con);
#region Parameters
SqlParameter parameter_Id = new SqlParameter();
parameter_Id.ParameterName = "@Id";
parameter_Id.Value = id;
cmd.Parameters.Add(parameter_Id);
SqlParameter parameter_FirstName = new SqlParameter();
parameter_FirstName.ParameterName = "@FirstName";
parameter_FirstName.Value = firstName;
cmd.Parameters.Add(parameter_FirstName);
SqlParameter parameter_LastName = new SqlParameter();
parameter_LastName.ParameterName = "@LastName";
parameter_LastName.Value = lastName;
cmd.Parameters.Add(parameter_LastName);
SqlParameter parameter_city = new SqlParameter();
parameter_city.ParameterName = "@City";
parameter_city.Value = city;
cmd.Parameters.Add(parameter_city);
SqlParameter parameter_Gender = new SqlParameter();
parameter_Gender.ParameterName = "@Gender";
parameter_Gender.Value = gender;
cmd.Parameters.Add(parameter_Gender);
SqlParameter parameter_DateOfBirth = new SqlParameter();
parameter_DateOfBirth.ParameterName = "@DateOfBirth";
parameter_DateOfBirth.Value = dateOfBirth;
cmd.Parameters.Add(parameter_DateOfBirth);
SqlParameter parameter_Country = new SqlParameter();
parameter_Country.ParameterName = "@Country";
parameter_Country.Value = country;
cmd.Parameters.Add(parameter_Country);
SqlParameter parameter_Salary = new SqlParameter();
parameter_Salary.ParameterName = "@Salary";
parameter_Salary.Value = salary;
cmd.Parameters.Add(parameter_Salary);
SqlParameter parameter_DateOfJoining = new SqlParameter();
parameter_DateOfJoining.ParameterName = "@DateOfJoining";
parameter_DateOfJoining.Value = dateOfJoining;
cmd.Parameters.Add(parameter_DateOfJoining);
SqlParameter parameter_MaritalStatus = new SqlParameter();
parameter_MaritalStatus.ParameterName = "@MaritalStatus";
parameter_MaritalStatus.Value = maritalStatus;
cmd.Parameters.Add(parameter_MaritalStatus);
#endregion
con.Open();
cmd.ExecuteNonQuery();
}
}
public static void DeleteEmployee(int id)
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("delete from tblEmployee where Id = @Id", con);
SqlParameter parameter_Id = new SqlParameter();
parameter_Id.ParameterName = "@Id";
parameter_Id.Value = id;
cmd.Parameters.Add(parameter_Id);
con.Open();
cmd.ExecuteNonQuery();
}
}
}
}
Step 3: Build your solution, so that the employee data access layer class is compiled.
Step 4: Configure ObjectDataSource1 control to retrieve data from EmployeeDataAccessLayer. Use GetAllEmployeesBasicDetails() as the SELECT method.
Step 5: Asscociate ObjectDataSource1 control with Gridview1 control, and make sure "Enable Selection" checkbox is checked.
Step 6: Configure ObjectDataSource2 control to retrieve data from EmployeeDataAccessLayer. Use GetEmployeesFullDetailsById(int Id) as the SELECT method. The value for the parameter - Id should come from the selected row in GridView1 control. Also, select INSERT, UPDATE and DELETE methods.
INSERT Method - InsertEmployee()
UPDATE Method - UpdateEmployee()
DELETE Method - DeleteEmployee()
Step 7: Associate ObjectDataSource2 with DetailsView1 control and make sure the following checkboxes are selected.
Enable Inserting
Enable Editing
Enable Deleting
Step 8: Generate ItemInserted, ItemUpdated and ItemDeleted event handler methods for DetailsView1 control.
Step 9: Copy and paste the following code in webform1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Page_PreRender(object sender, EventArgs e)
{
if (GridView1.SelectedRow == null)
{
DetailsView1.Visible = false;
}
else
{
DetailsView1.Visible = true;
}
}
protected void DetailsView1_ItemInserted(object sender, DetailsViewInsertedEventArgs e)
{
GridView1.DataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemDeleted(object sender, DetailsViewDeletedEventArgs e)
{
GridView1.DataBind();
GridView1.SelectRow(-1);
}
protected void DetailsView1_ItemUpdated(object sender, DetailsViewUpdatedEventArgs e)
{
GridView1.DataBind();
GridView1.SelectRow(-1);
}
Step 10: Set GridView1, DataKeyNames to "Id"
DataKeyNames="Id"
Step 11: Run the application. Select a row in gridview, and click "Edit". Notice that Id is also editable. To make it non editable, set ReadOnly attribute to true.
Step 12: Click on "New" link button in detailsview to insert a new row. Notice that, the interface shows a textbox for Id column. This column in the database is identity column, and we don't have to supply a value for it. To make it invisible, set InsertVisible attribute to false.
Setp 13: Click on Edit, change a few values, and click "Update". Notice that none of the rows are updated. To solve this issue, set DataKeyNames="Id" on DetailsView1 control.
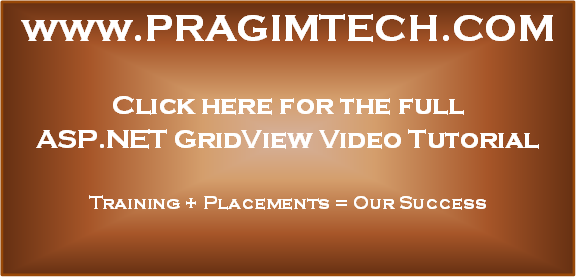
The Update doesn't work for me (as well as delete):
ReplyDeleteHere's what i get:
Description: An unhandled exception occurred during the execution of the current web request. Please review the stack trace for more information about the error and where it originated in the code.
Exception Details: MySql.Data.MySqlClient.MySqlException: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'where Id = 58379310' at line 1
Source Error:
Line 184:
Line 185: con2.Open();
Line 186: cmd2.ExecuteNonQuery();
Line 187: }
Line 188: }