Suggested Videos
Part 35 - Drilldown and display hierarchical data in gridview using objectdatasource control
Part 36 - Drilldown and display hierarchical data in gridview without using datasource controls
Part 37 - Detailsview in asp.net
In Part 37 of asp.net gridview tutorial, we discussed about using sqldatasource control with detailsview. In this video, we will discuss about using objectdatasource. We will be using tblEmployee table for this demo. If you need sql script to create this table, please refere to Part 37 by clicking here.
In a gridview control, I just want to show 3 columns (Id, FirstName and City) from tblEmployee table. As soon as I select a row, in the gridview control, then all the columns of the selected row, should be displayed in details view control as shown below.
To use objectdatasource controls, to retrieve employee data, we need to create employee data access layer.
Step 1: Add a class file with name EmployeeDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
namespace Demo
{
public class EmployeeBasic
{
public int Id { get; set; }
public string FirstName { get; set; }
public string City { get; set; }
}
public class Employee : EmployeeBasic
{
public string LastName { get; set; }
public string Gender { get; set; }
public DateTime DateOfBirth { get; set; }
public string Country { get; set; }
public int Salary { get; set; }
public DateTime DateOfJoining { get; set; }
public string MaritalStatus { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<EmployeeBasic> GetAllEmployeesBasicDetails()
{
List<EmployeeBasic> listEmployees = new List<EmployeeBasic>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select Id, FirstName, City from tblEmployee", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
EmployeeBasic employeeBasic = new EmployeeBasic();
employeeBasic.Id = Convert.ToInt32(rdr["Id"]);
employeeBasic.FirstName = rdr["FirstName"].ToString();
employeeBasic.City = rdr["City"].ToString();
listEmployees.Add(employeeBasic);
}
}
return listEmployees;
}
public static Employee GetAllEmployeesFullDetailsById(int Id)
{
Employee employee = new Employee();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select * from tblEmployee where Id = @Id", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@Id";
parameter.Value = Id;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
employee.Id = Convert.ToInt32(rdr["Id"]);
employee.FirstName = rdr["FirstName"].ToString();
employee.City = rdr["City"].ToString();
employee.LastName = rdr["LastName"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.DateOfBirth = Convert.ToDateTime(rdr["DateOfBirth"]);
employee.Country = rdr["Country"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
employee.DateOfJoining = Convert.ToDateTime(rdr["DateOfJoining"]);
employee.MaritalStatus = rdr["MaritalStatus"].ToString();
}
}
return employee;
}
}
}
Step 2: Build your solution, so that the employee data access layer class is compiled.
Step 3: Drag and drop a gridview and a detailsview control on webform1.aspx
Step 4: Drag and drop 2 objectdatasource controls
Step 5: Configure ObjectDataSource1 control to retrieve data from EmployeeDataAccessLayer. Use GetAllEmployeesBasicDetails() as the SELECT method.
Step 6: Asscociate ObjectDataSource1 control with Gridview1 control.
Step 7: Configure ObjectDataSource2 control to retrieve data from EmployeeDataAccessLayer. Use GetAllEmployeesFullDetailsById(int Id) as the SELECT method. The value for the parameter - Id should come fromt the selected row in GridView1 control.
Step 8: Associate ObjectDataSource2 with DetailsView1 control.
Step 9: Flip the webform to HTML source mode and set
GridView1 DataKeyNames property to "Id"
At this stage the HTML of your webform should be as shown below.
<asp:GridView ID="GridView1" runat="server"
DataKeyNames="Id" DataSourceID="ObjectDataSource1"
AutoGenerateColumns="False">
<Columns>
<asp:CommandField ShowSelectButton="True" />
<asp:BoundField DataField="Id" HeaderText="Id"
SortExpression="Id" />
<asp:BoundField DataField="FirstName"
HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="City" HeaderText="City"
SortExpression="City" />
</Columns>
</asp:GridView>
<br />
<asp:DetailsView ID="DetailsView1" runat="server"
AutoGenerateRows="False" Width="125px"
DataSourceID="ObjectDataSource2" Height="50px">
<Fields>
<asp:BoundField DataField="Id" HeaderText="Id"
SortExpression="Id" />
<asp:BoundField DataField="FirstName"
HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="City" HeaderText="City"
SortExpression="City" />
<asp:BoundField DataField="LastName"
HeaderText="LastName"
SortExpression="LastName" />
<asp:BoundField DataField="Gender"
HeaderText="Gender"
SortExpression="Gender" />
<asp:BoundField DataField="DateOfBirth"
HeaderText="DateOfBirth"
SortExpression="DateOfBirth" />
<asp:BoundField DataField="Country"
HeaderText="Country"
SortExpression="Country" />
<asp:BoundField DataField="Salary"
HeaderText="Salary"
SortExpression="Salary" />
<asp:BoundField DataField="DateOfJoining"
HeaderText="DateOfJoining"
SortExpression="DateOfJoining" />
<asp:BoundField DataField="MaritalStatus"
HeaderText="MaritalStatus"
SortExpression="MaritalStatus" />
</Fields>
</asp:DetailsView>
<br />
<asp:ObjectDataSource ID="ObjectDataSource1" runat="server"
SelectMethod="GetAllEmployeesBasicDetails"
TypeName="Demo.EmployeeDataAccessLayer">
</asp:ObjectDataSource>
<asp:ObjectDataSource ID="ObjectDataSource2" runat="server"
SelectMethod="GetAllEmployeesFullDetailsById"
TypeName="Demo.EmployeeDataAccessLayer">
<SelectParameters>
<asp:ControlParameter ControlID="GridView1" Name="Id"
PropertyName="SelectedValue" Type="Int32" />
</SelectParameters>
</asp:ObjectDataSource>
Now set the colour scheme of GridView1 and DetailsView1 to BrownSugar and run the application. DetailsView is rendered without any employee data. This is because we don't have any employee row selected in GridView1 intially. To correct this issue copy and paste the following code in webform1.aspx.cs
protected void Page_PreRender(object sender, EventArgs e)
{
if (GridView1.SelectedRow == null)
{
DetailsView1.Visible = false;
}
else
{
DetailsView1.Visible = true;
}
}
Part 35 - Drilldown and display hierarchical data in gridview using objectdatasource control
Part 36 - Drilldown and display hierarchical data in gridview without using datasource controls
Part 37 - Detailsview in asp.net
In Part 37 of asp.net gridview tutorial, we discussed about using sqldatasource control with detailsview. In this video, we will discuss about using objectdatasource. We will be using tblEmployee table for this demo. If you need sql script to create this table, please refere to Part 37 by clicking here.
In a gridview control, I just want to show 3 columns (Id, FirstName and City) from tblEmployee table. As soon as I select a row, in the gridview control, then all the columns of the selected row, should be displayed in details view control as shown below.

To use objectdatasource controls, to retrieve employee data, we need to create employee data access layer.
Step 1: Add a class file with name EmployeeDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
namespace Demo
{
public class EmployeeBasic
{
public int Id { get; set; }
public string FirstName { get; set; }
public string City { get; set; }
}
public class Employee : EmployeeBasic
{
public string LastName { get; set; }
public string Gender { get; set; }
public DateTime DateOfBirth { get; set; }
public string Country { get; set; }
public int Salary { get; set; }
public DateTime DateOfJoining { get; set; }
public string MaritalStatus { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<EmployeeBasic> GetAllEmployeesBasicDetails()
{
List<EmployeeBasic> listEmployees = new List<EmployeeBasic>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select Id, FirstName, City from tblEmployee", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
EmployeeBasic employeeBasic = new EmployeeBasic();
employeeBasic.Id = Convert.ToInt32(rdr["Id"]);
employeeBasic.FirstName = rdr["FirstName"].ToString();
employeeBasic.City = rdr["City"].ToString();
listEmployees.Add(employeeBasic);
}
}
return listEmployees;
}
public static Employee GetAllEmployeesFullDetailsById(int Id)
{
Employee employee = new Employee();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new
SqlCommand("Select * from tblEmployee where Id = @Id", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@Id";
parameter.Value = Id;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
employee.Id = Convert.ToInt32(rdr["Id"]);
employee.FirstName = rdr["FirstName"].ToString();
employee.City = rdr["City"].ToString();
employee.LastName = rdr["LastName"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.DateOfBirth = Convert.ToDateTime(rdr["DateOfBirth"]);
employee.Country = rdr["Country"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
employee.DateOfJoining = Convert.ToDateTime(rdr["DateOfJoining"]);
employee.MaritalStatus = rdr["MaritalStatus"].ToString();
}
}
return employee;
}
}
}
Step 2: Build your solution, so that the employee data access layer class is compiled.
Step 3: Drag and drop a gridview and a detailsview control on webform1.aspx
Step 4: Drag and drop 2 objectdatasource controls
Step 5: Configure ObjectDataSource1 control to retrieve data from EmployeeDataAccessLayer. Use GetAllEmployeesBasicDetails() as the SELECT method.
Step 6: Asscociate ObjectDataSource1 control with Gridview1 control.
Step 7: Configure ObjectDataSource2 control to retrieve data from EmployeeDataAccessLayer. Use GetAllEmployeesFullDetailsById(int Id) as the SELECT method. The value for the parameter - Id should come fromt the selected row in GridView1 control.
Step 8: Associate ObjectDataSource2 with DetailsView1 control.
Step 9: Flip the webform to HTML source mode and set
GridView1 DataKeyNames property to "Id"
At this stage the HTML of your webform should be as shown below.
<asp:GridView ID="GridView1" runat="server"
DataKeyNames="Id" DataSourceID="ObjectDataSource1"
AutoGenerateColumns="False">
<Columns>
<asp:CommandField ShowSelectButton="True" />
<asp:BoundField DataField="Id" HeaderText="Id"
SortExpression="Id" />
<asp:BoundField DataField="FirstName"
HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="City" HeaderText="City"
SortExpression="City" />
</Columns>
</asp:GridView>
<br />
<asp:DetailsView ID="DetailsView1" runat="server"
AutoGenerateRows="False" Width="125px"
DataSourceID="ObjectDataSource2" Height="50px">
<Fields>
<asp:BoundField DataField="Id" HeaderText="Id"
SortExpression="Id" />
<asp:BoundField DataField="FirstName"
HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="City" HeaderText="City"
SortExpression="City" />
<asp:BoundField DataField="LastName"
HeaderText="LastName"
SortExpression="LastName" />
<asp:BoundField DataField="Gender"
HeaderText="Gender"
SortExpression="Gender" />
<asp:BoundField DataField="DateOfBirth"
HeaderText="DateOfBirth"
SortExpression="DateOfBirth" />
<asp:BoundField DataField="Country"
HeaderText="Country"
SortExpression="Country" />
<asp:BoundField DataField="Salary"
HeaderText="Salary"
SortExpression="Salary" />
<asp:BoundField DataField="DateOfJoining"
HeaderText="DateOfJoining"
SortExpression="DateOfJoining" />
<asp:BoundField DataField="MaritalStatus"
HeaderText="MaritalStatus"
SortExpression="MaritalStatus" />
</Fields>
</asp:DetailsView>
<br />
<asp:ObjectDataSource ID="ObjectDataSource1" runat="server"
SelectMethod="GetAllEmployeesBasicDetails"
TypeName="Demo.EmployeeDataAccessLayer">
</asp:ObjectDataSource>
<asp:ObjectDataSource ID="ObjectDataSource2" runat="server"
SelectMethod="GetAllEmployeesFullDetailsById"
TypeName="Demo.EmployeeDataAccessLayer">
<SelectParameters>
<asp:ControlParameter ControlID="GridView1" Name="Id"
PropertyName="SelectedValue" Type="Int32" />
</SelectParameters>
</asp:ObjectDataSource>
Now set the colour scheme of GridView1 and DetailsView1 to BrownSugar and run the application. DetailsView is rendered without any employee data. This is because we don't have any employee row selected in GridView1 intially. To correct this issue copy and paste the following code in webform1.aspx.cs
protected void Page_PreRender(object sender, EventArgs e)
{
if (GridView1.SelectedRow == null)
{
DetailsView1.Visible = false;
}
else
{
DetailsView1.Visible = true;
}
}
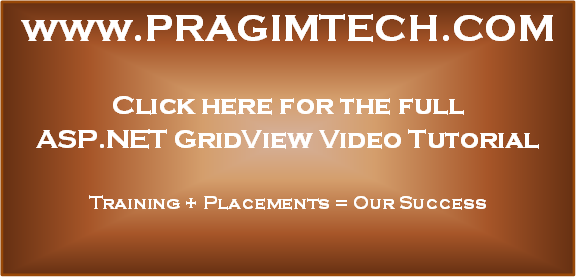
No comments:
Post a Comment
It would be great if you can help share these free resources