Suggested Videos
Part 32 - Displaying GridView in a GridView
Part 33 - Merging cells in gridview footer row
Part 34 - Drilldown and display hierarchical data in an asp.net gridview using sqldatasource control
In this video, we will discuss about drilling down and displaying hierarchical data in gridview controls using objectdatasource control. We will be using the following 3 database tables for this demo.
1. tblContinents
2. tblCountries
3. tblCities
1. When the page loads, a gridview should display the list of all continents available in tblContinents table
2. When a "Select" button is clicked against a continent, all the countries belonging to the selected continent should be displayed in another gridview.
3. Along the same lines, when a "Select" button is clicked against a country, all the cities belonging to the selected country should be displayed in another gridview.
Use sql script from Part 34 by clicking here to create and populate the required tables.
To use objectdatasource controls, to retrieve continents, countries and cities data, we need to create respective data access layer class files.
Step 1: Add a class file with name ContinentDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Continent
{
public int ContinentId { get; set; }
public string ContinentName { get; set; }
}
public class ContinentDataAccessLayer
{
public static List<Continent> GetAllContinents()
{
List<Continent> listContinents = new List<Continent>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("Select * from tblContinents", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Continent continent = new Continent();
continent.ContinentId = Convert.ToInt32(rdr["ContinentId"]);
continent.ContinentName = rdr["ContinentName"].ToString();
listContinents.Add(continent);
}
}
return listContinents;
}
}
}
Step 2: Add a class file with name CountryDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Country
{
public int CountryId { get; set; }
public string CountryName { get; set; }
public int ContinentId { get; set; }
}
public class CountryDataAccessLayer
{
public static List<Country> GetCountriesByContinent(int ContinentId)
{
List<Country> listCountries = new List<Country>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("Select * from tblCountries where ContinentId = @ContinentId", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@ContinentId";
parameter.Value = ContinentId;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Country country = new Country();
country.CountryId = Convert.ToInt32(rdr["CountryId"]);
country.CountryName = rdr["CountryName"].ToString();
country.ContinentId = Convert.ToInt32(rdr["ContinentId"]);
listCountries.Add(country);
}
}
return listCountries;
}
}
}
Step 3: Add a class file with name CityDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class City
{
public int CityId { get; set; }
public string CityName { get; set; }
public int CountryId { get; set; }
}
public class CityDataAccessLayer
{
public static List<City> GetCitiesByCountryId(int CountryId)
{
List<City> listCities = new List<City>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("Select * from tblCities where CountryId = @CountryId", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@CountryId";
parameter.Value = CountryId;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
City city = new City();
city.CityId = Convert.ToInt32(rdr["CityId"]);
city.CityName = rdr["CityName"].ToString();
city.CountryId = Convert.ToInt32(rdr["CountryId"]);
listCities.Add(city);
}
}
return listCities;
}
}
}
Step 4: Build your solution, so that the respective data access layer classes are compiled.
Step 5: Drag and drop 3 gridview controls and 3 Objectdatasource controls on webform1.aspx.
Step 6: Configure ObjectDataSource1 control to retrieve data from ContinentDataAccessLayer. Use GetAllContinents() as the SELECT method.
Step 7: Asscociate ObjectDataSource1 control with Gridview1 control.
Setp 8: From GridView1 - GridView Tasks pane, please make sure "Enable selection" checkbox is checked.
Step 9: Configure ObjectDataSource2 control to retrieve data from CountryDataAccessLayer. Use GetCountriesByContinent(int ContinentId) as the SELECT method. The value for the parameter - ContinentId should come fromt the selected row in GridView1 control.
Step 10: Asscociate ObjectDataSource2 control with Gridview2 control.
Step 11: From GridView2 - GridView Tasks pane, please make sure "Enable selection" checkbox is checked.
Step 12: Configure ObjectDataSource3 control to retrieve data from CityDataAccessLayer. Use GetCitiesByCountryId(int CountryId) as the SELECT method. The value for the parameter - CountryId should come fromt the selected row in GridView2 control.
Step 13: Asscociate ObjectDataSource3 control with Gridview3 control.
Step 14: Flip the webform to HTML source mode and set
GridView1 DataKeyNames property to "ContinentId"
GridView2 DataKeyNames property to "CountryId"
At this stage the HTML of your webform should be as shown below.
Part 32 - Displaying GridView in a GridView
Part 33 - Merging cells in gridview footer row
Part 34 - Drilldown and display hierarchical data in an asp.net gridview using sqldatasource control
In this video, we will discuss about drilling down and displaying hierarchical data in gridview controls using objectdatasource control. We will be using the following 3 database tables for this demo.
1. tblContinents
2. tblCountries
3. tblCities
1. When the page loads, a gridview should display the list of all continents available in tblContinents table
2. When a "Select" button is clicked against a continent, all the countries belonging to the selected continent should be displayed in another gridview.
3. Along the same lines, when a "Select" button is clicked against a country, all the cities belonging to the selected country should be displayed in another gridview.

Use sql script from Part 34 by clicking here to create and populate the required tables.
To use objectdatasource controls, to retrieve continents, countries and cities data, we need to create respective data access layer class files.
Step 1: Add a class file with name ContinentDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Continent
{
public int ContinentId { get; set; }
public string ContinentName { get; set; }
}
public class ContinentDataAccessLayer
{
public static List<Continent> GetAllContinents()
{
List<Continent> listContinents = new List<Continent>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("Select * from tblContinents", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Continent continent = new Continent();
continent.ContinentId = Convert.ToInt32(rdr["ContinentId"]);
continent.ContinentName = rdr["ContinentName"].ToString();
listContinents.Add(continent);
}
}
return listContinents;
}
}
}
Step 2: Add a class file with name CountryDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Country
{
public int CountryId { get; set; }
public string CountryName { get; set; }
public int ContinentId { get; set; }
}
public class CountryDataAccessLayer
{
public static List<Country> GetCountriesByContinent(int ContinentId)
{
List<Country> listCountries = new List<Country>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("Select * from tblCountries where ContinentId = @ContinentId", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@ContinentId";
parameter.Value = ContinentId;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Country country = new Country();
country.CountryId = Convert.ToInt32(rdr["CountryId"]);
country.CountryName = rdr["CountryName"].ToString();
country.ContinentId = Convert.ToInt32(rdr["ContinentId"]);
listCountries.Add(country);
}
}
return listCountries;
}
}
}
Step 3: Add a class file with name CityDataAccessLayer.cs. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class City
{
public int CityId { get; set; }
public string CityName { get; set; }
public int CountryId { get; set; }
}
public class CityDataAccessLayer
{
public static List<City> GetCitiesByCountryId(int CountryId)
{
List<City> listCities = new List<City>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlCommand cmd = new SqlCommand("Select * from tblCities where CountryId = @CountryId", con);
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@CountryId";
parameter.Value = CountryId;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
City city = new City();
city.CityId = Convert.ToInt32(rdr["CityId"]);
city.CityName = rdr["CityName"].ToString();
city.CountryId = Convert.ToInt32(rdr["CountryId"]);
listCities.Add(city);
}
}
return listCities;
}
}
}
Step 4: Build your solution, so that the respective data access layer classes are compiled.
Step 5: Drag and drop 3 gridview controls and 3 Objectdatasource controls on webform1.aspx.
Step 6: Configure ObjectDataSource1 control to retrieve data from ContinentDataAccessLayer. Use GetAllContinents() as the SELECT method.
Step 7: Asscociate ObjectDataSource1 control with Gridview1 control.
Setp 8: From GridView1 - GridView Tasks pane, please make sure "Enable selection" checkbox is checked.
Step 9: Configure ObjectDataSource2 control to retrieve data from CountryDataAccessLayer. Use GetCountriesByContinent(int ContinentId) as the SELECT method. The value for the parameter - ContinentId should come fromt the selected row in GridView1 control.
Step 10: Asscociate ObjectDataSource2 control with Gridview2 control.
Step 11: From GridView2 - GridView Tasks pane, please make sure "Enable selection" checkbox is checked.
Step 12: Configure ObjectDataSource3 control to retrieve data from CityDataAccessLayer. Use GetCitiesByCountryId(int CountryId) as the SELECT method. The value for the parameter - CountryId should come fromt the selected row in GridView2 control.
Step 13: Asscociate ObjectDataSource3 control with Gridview3 control.
Step 14: Flip the webform to HTML source mode and set
GridView1 DataKeyNames property to "ContinentId"
GridView2 DataKeyNames property to "CountryId"
At this stage the HTML of your webform should be as shown below.

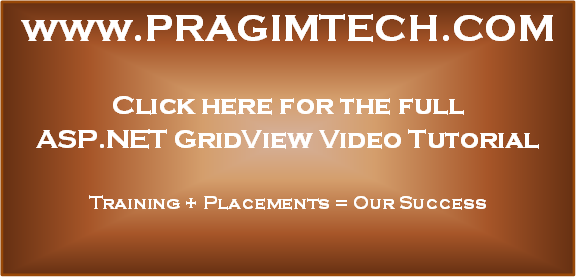
No comments:
Post a Comment
It would be great if you can help share these free resources