Suggested Videos
Part 33 - Merging cells in gridview footer row
Part 34 - Drilldown and display hierarchical data in gridview using sqldatasource control
Part 35 - Drilldown and display hierarchical data in gridview using objectdatasource control
In this video, we will discuss about drilling down and displaying hierarchical data in gridview controls without using any datasource control. We will be using the following 3 database tables for this demo.
1. tblContinents
2. tblCountries
3. tblCities
1. When the page loads, a gridview should display the list of all continents available in tblContinents table
2. When a "Select" button is clicked against a continent, all the countries belonging to the selected continent should be displayed in another gridview.
3. Along the same lines, when a "Select" button is clicked against a country, all the cities belonging to the selected country should be displayed in another gridview.
Use sql script from Part 34 by clicking here to create and populate the required tables.
To retrieve continents, countries and cities data and display in gridview without using datasource controls, we need to create respective data access layer class files. We discussed about this in Part 35. Click here if you need this code.
Now drag and drop 3 gridview controls on webform1.aspx.
Configure GridView1, to include 2 TemplateFields and 1 BoundField.
1. The first TemplateField should contain an ItemTemplate, which includes a linkbutton, that the user can click.
2. Set the CommandName property of the LinkButton to SelectCountries.
3. The second TemplateField should contain an ItemTemplate, which includes a Label control, that binds to ContinentId column.
4. The BoundField get it's data from ContinentName column.
5. Set AutoGenerateColumns property of GridView1 to false.
Configure GridView2, to include 2 TemplateFields and 2 BoundFields.
1. The first TemplateField should contain an ItemTemplate, which includes a linkbutton, that the user can click.
2. Set the CommandName property of the LinkButton to SelectCities.
3. The first TemplateField should contain an ItemTemplate, which includes a Label control, that binds to CountryId coulmn.
4. The first BoundField get it's data from CountryName column.
5. The second BoundField get it's data from ContinentId column.
6. Set AutoGenerateColumns property of GridView2 to false.
7. Set GridView2 property, DataKeyNames="CountryId".
GridView3 does not require any additonal configuration. Just the HTML as shown below.
Now generate RowCommand event handler methods for GridView1 and GridView2. At this point the HTML on WebForm1.aspx should be as shown below.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false"
onrowcommand="GridView1_RowCommand">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton CommandName="SelectCountries"
ID="btnSelectCountries"
runat="server">Select</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Continent Id">
<ItemTemplate>
<asp:Label ID="Label1" runat="server"
Text='<%# Bind("ContinentId") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="ContinentName"
HeaderText="Continent Name" />
</Columns>
</asp:GridView>
<asp:GridView ID="GridView2" runat="server" AutoGenerateColumns="false"
onrowcommand="GridView2_RowCommand" DataKeyNames="CountryId">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton CommandName="SelectCities" ID="btnSelectCities"
runat="server">Select</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Country Id">
<ItemTemplate>
<asp:Label ID="Label2" runat="server"
Text='<%# Bind("CountryId") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="CountryName" HeaderText="Country Name" />
<asp:BoundField DataField="ContinentId" HeaderText="Continent Id" />
</Columns>
</asp:GridView>
<asp:GridView ID="GridView3" runat="server">
</asp:GridView>
Copy and paste the following code in webform1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = ContinentDataAccessLayer.GetAllContinents();
GridView1.DataBind();
}
}
protected void GridView1_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "SelectCountries")
{
int rowIndex = ((GridViewRow)((LinkButton)e.CommandSource).NamingContainer).RowIndex;
Label lblContinent = (Label)GridView1.Rows[rowIndex].FindControl("Label1");
int continentId = Convert.ToInt32(lblContinent.Text);
GridView2.DataSource = CountryDataAccessLayer.GetCountriesByContinent(continentId);
GridView2.DataBind();
GridView1.SelectRow(rowIndex);
if (GridView2.SelectedValue != null)
{
GridView3.DataSource = CityDataAccessLayer.GetCitiesByCountryId(Convert.ToInt32(GridView2.SelectedValue));
GridView3.DataBind();
}
}
}
protected void GridView2_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "SelectCities")
{
int rowIndex = ((GridViewRow)((LinkButton)e.CommandSource).NamingContainer).RowIndex;
Label lblCountry = (Label)GridView2.Rows[rowIndex].FindControl("Label2");
int countryId = Convert.ToInt32(lblCountry.Text);
GridView3.DataSource = CityDataAccessLayer.GetCitiesByCountryId(countryId);
GridView3.DataBind();
GridView2.SelectRow(rowIndex);
}
}
Part 33 - Merging cells in gridview footer row
Part 34 - Drilldown and display hierarchical data in gridview using sqldatasource control
Part 35 - Drilldown and display hierarchical data in gridview using objectdatasource control
In this video, we will discuss about drilling down and displaying hierarchical data in gridview controls without using any datasource control. We will be using the following 3 database tables for this demo.
1. tblContinents
2. tblCountries
3. tblCities
1. When the page loads, a gridview should display the list of all continents available in tblContinents table
2. When a "Select" button is clicked against a continent, all the countries belonging to the selected continent should be displayed in another gridview.
3. Along the same lines, when a "Select" button is clicked against a country, all the cities belonging to the selected country should be displayed in another gridview.

Use sql script from Part 34 by clicking here to create and populate the required tables.
To retrieve continents, countries and cities data and display in gridview without using datasource controls, we need to create respective data access layer class files. We discussed about this in Part 35. Click here if you need this code.
Now drag and drop 3 gridview controls on webform1.aspx.
Configure GridView1, to include 2 TemplateFields and 1 BoundField.
1. The first TemplateField should contain an ItemTemplate, which includes a linkbutton, that the user can click.
2. Set the CommandName property of the LinkButton to SelectCountries.
3. The second TemplateField should contain an ItemTemplate, which includes a Label control, that binds to ContinentId column.
4. The BoundField get it's data from ContinentName column.
5. Set AutoGenerateColumns property of GridView1 to false.
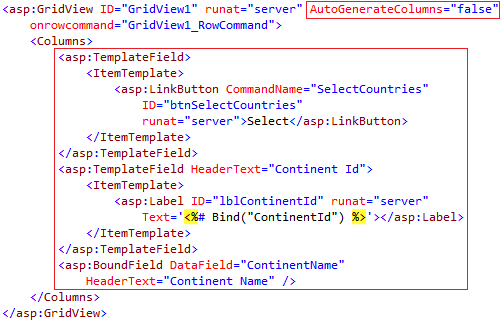
Configure GridView2, to include 2 TemplateFields and 2 BoundFields.
1. The first TemplateField should contain an ItemTemplate, which includes a linkbutton, that the user can click.
2. Set the CommandName property of the LinkButton to SelectCities.
3. The first TemplateField should contain an ItemTemplate, which includes a Label control, that binds to CountryId coulmn.
4. The first BoundField get it's data from CountryName column.
5. The second BoundField get it's data from ContinentId column.
6. Set AutoGenerateColumns property of GridView2 to false.
7. Set GridView2 property, DataKeyNames="CountryId".

GridView3 does not require any additonal configuration. Just the HTML as shown below.

Now generate RowCommand event handler methods for GridView1 and GridView2. At this point the HTML on WebForm1.aspx should be as shown below.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false"
onrowcommand="GridView1_RowCommand">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton CommandName="SelectCountries"
ID="btnSelectCountries"
runat="server">Select</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Continent Id">
<ItemTemplate>
<asp:Label ID="Label1" runat="server"
Text='<%# Bind("ContinentId") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="ContinentName"
HeaderText="Continent Name" />
</Columns>
</asp:GridView>
<asp:GridView ID="GridView2" runat="server" AutoGenerateColumns="false"
onrowcommand="GridView2_RowCommand" DataKeyNames="CountryId">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton CommandName="SelectCities" ID="btnSelectCities"
runat="server">Select</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Country Id">
<ItemTemplate>
<asp:Label ID="Label2" runat="server"
Text='<%# Bind("CountryId") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="CountryName" HeaderText="Country Name" />
<asp:BoundField DataField="ContinentId" HeaderText="Continent Id" />
</Columns>
</asp:GridView>
<asp:GridView ID="GridView3" runat="server">
</asp:GridView>
Copy and paste the following code in webform1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = ContinentDataAccessLayer.GetAllContinents();
GridView1.DataBind();
}
}
protected void GridView1_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "SelectCountries")
{
int rowIndex = ((GridViewRow)((LinkButton)e.CommandSource).NamingContainer).RowIndex;
Label lblContinent = (Label)GridView1.Rows[rowIndex].FindControl("Label1");
int continentId = Convert.ToInt32(lblContinent.Text);
GridView2.DataSource = CountryDataAccessLayer.GetCountriesByContinent(continentId);
GridView2.DataBind();
GridView1.SelectRow(rowIndex);
if (GridView2.SelectedValue != null)
{
GridView3.DataSource = CityDataAccessLayer.GetCitiesByCountryId(Convert.ToInt32(GridView2.SelectedValue));
GridView3.DataBind();
}
}
}
protected void GridView2_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "SelectCities")
{
int rowIndex = ((GridViewRow)((LinkButton)e.CommandSource).NamingContainer).RowIndex;
Label lblCountry = (Label)GridView2.Rows[rowIndex].FindControl("Label2");
int countryId = Convert.ToInt32(lblCountry.Text);
GridView3.DataSource = CityDataAccessLayer.GetCitiesByCountryId(countryId);
GridView3.DataBind();
GridView2.SelectRow(rowIndex);
}
}
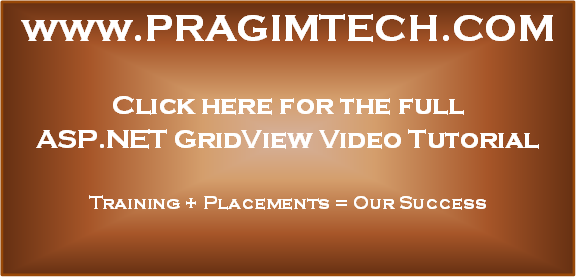
if i select a particular person record in gridview,then will it possible to show their complete details in detail view along with their photo
ReplyDeleteHi Malavika, details view concepts are discussed in Parts 37 to 41 in asp.net gridview tutorial. Please click here to access asp.net gridview tutorial. I hope you will find them useful.
DeleteHi Venkat,
ReplyDeleteI am using .net framework 3.5.
could not find the 'gridview.selectrow(rowindex)' method in onrowcommand event, instead I am getting SelectedRow method. is there any way around to select the row in onrowcommand event in .net 3.5?
Thanks in advance.