Suggested Videos
Part 20 - Create form in asp.net core razor pages | Text | Slides
Part 21 - ASP.NET Core razor pages client side validation | Text | Slides
Part 22 - Delete operation in asp.net core razor pages | Text | Slides
In this video we will discuss Partial Views in ASP.NET Core.
What is a partial view
ASP.NET Core partial view example
Consider the following Employee List (/Employees/Index) razor page.
Employee List razor page (/Employees/Index)
We have the following code on this page
Now, consider the following Delete razor page (/Employees/Delete)
Delete razor page (/Employees/Delete)
Notice, the code to display Employee Name, and Photo is duplicated even on this page (in red colour).
Use partial view to reuse code
In general, code duplication is not good. The code that displays Employee Name, Photo and three buttons (View, Edit and Delete) can be encapsulated into a partial view. This partial view can then be reused on any number of pages, even from a layout page.
Partial view naming convention
A partial view file name usually begins with an underscore (_). It's a naming convention. Just by looking at the leading underscore in the name we can easily say this is a partial view and not a regular razor view or page. We named our partial view _DisplayEmployeePartial.cshtml
Partial view discovery
If the partial view is placed in a specific folder in the Pages folder, then only the views or pages in that specific folder can use the partial view. If you want to use the partial view from the pages or views from a different sub-folder, place it in the Shared folder.
Rendering partial view
To render partial view, use the <partial> tag helper. Use the model attribute to pass Model data. If you want to pass any other additional data you can also use the ViewData dictionary.
Rendering partial view from Employee List razor page (/Employees/Index)
Rendering partial view from Delete razor page (/Employees/Delete)
Part 20 - Create form in asp.net core razor pages | Text | Slides
Part 21 - ASP.NET Core razor pages client side validation | Text | Slides
Part 22 - Delete operation in asp.net core razor pages | Text | Slides
In this video we will discuss Partial Views in ASP.NET Core.
What is a partial view
- Just like a razor page or an MVC view, a partial view also has a .cshtml extension.
- Partial view enables code reuse.
- Encapsulates HTML and C# code that can be reused on multiple razor pages or view.
ASP.NET Core partial view example
Consider the following Employee List (/Employees/Index) razor page.

Employee List razor page (/Employees/Index)
We have the following code on this page
- The code to loop through the list of employees
- The code to display Employee Name, Photo and the 3 buttons - View, Edit and Delete.
@page
@model
RazorPagesTutorial.Pages.Employees.IndexModel
@{
ViewData["Title"] = "Index";
}
<style>
.btn {
width: 75px;
}
</style>
<h1>Employees</h1>
<div class="card-deck">
@foreach (var employee in Model.Employees)
{
var photoPath = "~/images/" + (employee.PhotoPath
?? "noimage.jpg");
<div class="card m-3" style="min-width: 18rem; max-width:30.5%;">
<div class="card-header">
<h3>@employee.Name</h3>
</div>
<img class="card-img-top imageThumbnail" src="@photoPath"
asp-append-version="true" />
<div class="card-footer text-center">
<a asp-page="/Employees/Details" asp-route-ID="@employee.Id"
class="btn btn-primary
m-1">View</a>
<a asp-page="/Employees/Edit" asp-route-ID="@employee.Id"
class="btn btn-primary
m-1">Edit</a>
<a asp-page="/Employees/Delete" asp-route-ID="@employee.Id"
class="btn btn-danger
m-1">Delete</a>
</div>
</div>
}
</div>
Now, consider the following Delete razor page (/Employees/Delete)
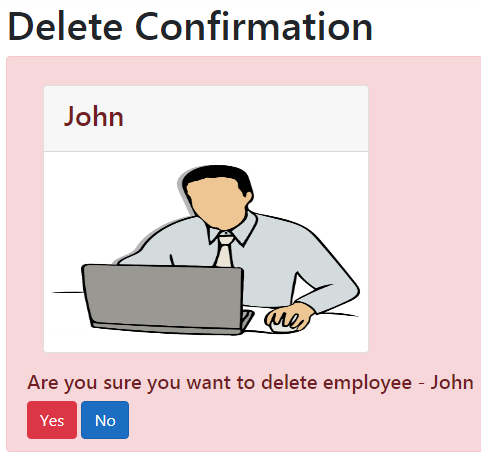
Delete razor page (/Employees/Delete)
Notice, the code to display Employee Name, and Photo is duplicated even on this page (in red colour).
@page "{id}"
@model
RazorPagesTutorial.Pages.Employees.DeleteModel
@{
ViewData["Title"] = "Delete";
var photoPath = "~/images/" +
(Model.Employee.PhotoPath ?? "noimage.jpg");
}
<h1>Delete Confirmation</h1>
<div class="alert alert-danger">
<div class="card m-3" style="min-width: 18rem; max-width:30.5%;">
<div class="card-header">
<h3>@Model.Employee.Name</h3>
</div>
<img class="card-img-top
imageThumbnail" src="@photoPath"
asp-append-version="true" />
</div>
<h5>Are you sure you want
to delete employee - @Model.Employee.Name</h5>
<form method="post">
<button type="submit" class="btn
btn-danger">Yes</button>
<a asp-page="/Employees/Index" class="btn
btn-primary">No</a>
</form>
</div>
Use partial view to reuse code
In general, code duplication is not good. The code that displays Employee Name, Photo and three buttons (View, Edit and Delete) can be encapsulated into a partial view. This partial view can then be reused on any number of pages, even from a layout page.
Partial view naming convention
A partial view file name usually begins with an underscore (_). It's a naming convention. Just by looking at the leading underscore in the name we can easily say this is a partial view and not a regular razor view or page. We named our partial view _DisplayEmployeePartial.cshtml
Partial view discovery
If the partial view is placed in a specific folder in the Pages folder, then only the views or pages in that specific folder can use the partial view. If you want to use the partial view from the pages or views from a different sub-folder, place it in the Shared folder.

@model Employee
@{
var photoPath = "~/images/" + (Model.PhotoPath ??
"noimage.jpg");
//
View, Edit and Delete buttons must not be displayed on the Delete razor
//
page. They should be displayed only on the Employee List razor pages
//
These pages will pass true or false using ViewData dictionary
bool showButtons = (bool)ViewData["ShowButtons"];
}
<div class="card m-3" style="min-width: 18rem; max-width:30.5%;">
<div class="card-header">
<h3>@Model.Name</h3>
</div>
<img class="card-img-top
imageThumbnail" src="@photoPath"
asp-append-version="true" />
@if (showButtons)
{
<div class="card-footer
text-center">
<a asp-page="/Employees/Details" asp-route-ID="@Model.Id"
class="btn btn-primary m-1">View</a>
<a asp-page="/Employees/Edit" asp-route-ID="@Model.Id"
class="btn btn-primary m-1">Edit</a>
<a asp-page="/Employees/Delete" asp-route-ID="@Model.Id"
class="btn btn-danger m-1">Delete</a>
</div>
}
</div>
Rendering partial view
To render partial view, use the <partial> tag helper. Use the model attribute to pass Model data. If you want to pass any other additional data you can also use the ViewData dictionary.
@{
ViewData["ShowButtons"] = true;
}
<partial name="_DisplayEmployeePartial" model="employee" view-data="ViewData" />
Rendering partial view from Employee List razor page (/Employees/Index)
@page
@model
RazorPagesTutorial.Pages.Employees.IndexModel
@{
ViewData["Title"] = "Index";
//
Pass true using ViewData to tell the partial view
//
to display View, Edit and Delete buttons
ViewData["ShowButtons"] = true;
}
<style>
.btn {
width: 75px;
}
</style>
<h1>Employees</h1>
<div class="card-deck">
@foreach (var employee in Model.Employees)
{
//
Render partial view passing it the model data and ViewData dictionary
<partial name="_DisplayEmployeePartial" model="employee" view-data="ViewData" />
}
</div>
Rendering partial view from Delete razor page (/Employees/Delete)
@page "{id}"
@model
RazorPagesTutorial.Pages.Employees.DeleteModel
@{
ViewData["Title"] = "Delete";
//
Pass true using ViewData to tell the partial view
//
to display View, Edit and Delete buttons
ViewData["ShowButtons"] = false;
}
<h1>Delete Confirmation</h1>
<div class="alert alert-danger">
@*Render partial
view passing it the model data and ViewData dictionary*@
<partial name="_DisplayEmployeePartial" model="Model.Employee" view-data="ViewData" />
<h5>Are you sure you want
to delete employee - @Model.Employee.Name</h5>
<form method="post">
<button type="submit" class="btn
btn-danger">Yes</button>
<a asp-page="/Employees/Index" class="btn
btn-primary">No</a>
</form>
</div>

No comments:
Post a Comment
It would be great if you can help share these free resources