Suggested Videos
Part 71 - Authorization in ASP.NET Core | Text | Slides
Part 72 - Redirect user to original url after login in asp.net core | Text | Slides
Part 73 - Open redirect vulnerability example | Text | Slides
In this video we will discuss how to implement client side validation in asp.net core.
Server Side Validation in ASP.NET Core
We discussed implementing, server side validation in Parts 42 and 43 of ASP.NET Core tutorial. Server side validation is implemented by using validation attributes such as Required, StringLength etc.
As the name implies, server side validation is done on the server. So there is a round trip between the client browser and the web server. The request has to be sent over the network to the web server for processing. This means if the network is slow or if the server is busy processing other requests, the end user may have to wait a few seconds and it also increases load on the server.
This validation can be performed on the client machine itself, which means there is no round trip to the server, no waiting time, client has instant feedback and the load on the server is also reduced to a great extent.
Server Side Validation Example
Consider the following login page
To make the Email and Password fields required, we decorate the respective model properties with the [Required] attribute. We discussed how to use these validation attributes and implement server side model validation in our previous videos in this series. These same validation attributes are also used to implement client side validation in asp.net core.
Client Side Validation
There are 2 approaches that you could take to implement client side validation in asp.net core.
Approach 1 : Write your own custom JavaScript code to implement client-side validation which is obviously tedious and time consuming.
Approach 2 : Use the unobtrusive client-side validation libraries provided by asp.net core.
With the second approach, we do not have to write a single line of code. All we have to do is include the following 3 scripts in the order specified.
You may use Library Manager to install client side libraries. Library Manager is discussed in Part 34 of ASP.NET Core tutorial.
What does "Unobtrusive Validation" Mean
Unobtrusive Validation allows us to take the already-existing server side validation attributes and use them to implement client-side validation. We do not have to write a single line of custom JavaScript code. All we need is the above 3 script files in the order specified.
The unobtrusive validation is done using the jquery.validate.unobtrusive.js library. This library is built on top of jquery.validate.js library, which in turns uses jQuery. This is the reason we need to import these 3 libraries in the order specified.
How does client side validation work in ASP.NET Core
The Email property is decorated with [Required] attribute. To generate input field for Email, we use asp.net core input tag helper
ASP.NET Core tag helpers work in combination with the model validation attributes and generate the following HTML. Notice in the generated HTML we have data-* attributes.
The data-* attributes allow us to add extra information to an HTML element. These data-* attributes carry all the information required to perform the client-side validation. It is the unobtrusive library (i.e jquery.validate.unobtrusive.js) that reads these data-val attributes and performs the client side validation.
Unobtrusive validation not working in ASP.NET Core
If the unobtrusive client-side validation is not working, make sure you check the following.
Make sure browser support for JavaScript is not disabled
Make sure the following client-side validation libraries are loaded in the order specified
If there's another reason why client side validation is not working, please leave it as a comment so it could help others.
Part 71 - Authorization in ASP.NET Core | Text | Slides
Part 72 - Redirect user to original url after login in asp.net core | Text | Slides
Part 73 - Open redirect vulnerability example | Text | Slides
In this video we will discuss how to implement client side validation in asp.net core.
Server Side Validation in ASP.NET Core
We discussed implementing, server side validation in Parts 42 and 43 of ASP.NET Core tutorial. Server side validation is implemented by using validation attributes such as Required, StringLength etc.
As the name implies, server side validation is done on the server. So there is a round trip between the client browser and the web server. The request has to be sent over the network to the web server for processing. This means if the network is slow or if the server is busy processing other requests, the end user may have to wait a few seconds and it also increases load on the server.
This validation can be performed on the client machine itself, which means there is no round trip to the server, no waiting time, client has instant feedback and the load on the server is also reduced to a great extent.
Server Side Validation Example
Consider the following login page
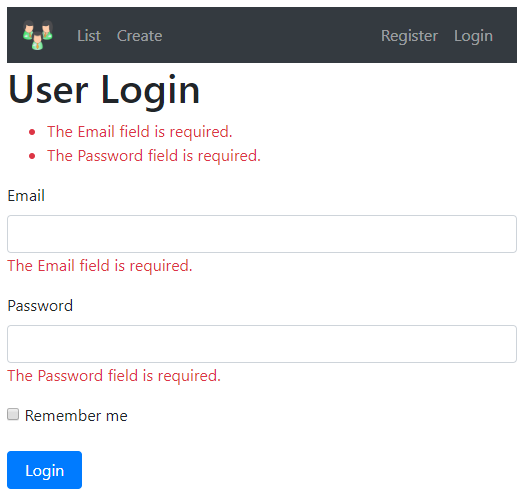
To make the Email and Password fields required, we decorate the respective model properties with the [Required] attribute. We discussed how to use these validation attributes and implement server side model validation in our previous videos in this series. These same validation attributes are also used to implement client side validation in asp.net core.
public class LoginViewModel
{
[Required]
public string Email { get; set; }
[Required]
public string Password
{ get; set; }
}
Client Side Validation
There are 2 approaches that you could take to implement client side validation in asp.net core.
Approach 1 : Write your own custom JavaScript code to implement client-side validation which is obviously tedious and time consuming.
Approach 2 : Use the unobtrusive client-side validation libraries provided by asp.net core.
With the second approach, we do not have to write a single line of code. All we have to do is include the following 3 scripts in the order specified.
<script src="~/lib/jquery/jquery.js"></script>
<script src="~/lib/jquery-validate/jquery.validate.js"></script>
<script src="~/lib/jquery-validation-unobtrusive/jquery.validate.unobtrusive.js"></script>
You may use Library Manager to install client side libraries. Library Manager is discussed in Part 34 of ASP.NET Core tutorial.
What does "Unobtrusive Validation" Mean
Unobtrusive Validation allows us to take the already-existing server side validation attributes and use them to implement client-side validation. We do not have to write a single line of custom JavaScript code. All we need is the above 3 script files in the order specified.
The unobtrusive validation is done using the jquery.validate.unobtrusive.js library. This library is built on top of jquery.validate.js library, which in turns uses jQuery. This is the reason we need to import these 3 libraries in the order specified.
How does client side validation work in ASP.NET Core
The Email property is decorated with [Required] attribute. To generate input field for Email, we use asp.net core input tag helper
<input asp-for="Email"/>
ASP.NET Core tag helpers work in combination with the model validation attributes and generate the following HTML. Notice in the generated HTML we have data-* attributes.
<input id="Email" name="Email" type="email" data-val="true"
data-val-required="The Email field is required." />
The data-* attributes allow us to add extra information to an HTML element. These data-* attributes carry all the information required to perform the client-side validation. It is the unobtrusive library (i.e jquery.validate.unobtrusive.js) that reads these data-val attributes and performs the client side validation.
Unobtrusive validation not working in ASP.NET Core
If the unobtrusive client-side validation is not working, make sure you check the following.
Make sure browser support for JavaScript is not disabled
Make sure the following client-side validation libraries are loaded in the order specified
- jquery.js
- jquery.validate.js
- jquery.validate.unobtrusive.js
If there's another reason why client side validation is not working, please leave it as a comment so it could help others.

Thanks a lot Sir, heads off for your effort.
ReplyDeleteHi Team,
ReplyDeleteWhen ever I'm trying to the jQuery-Validate library from client side library option, its not getting added to the folder wwwroot/lib/. Instead its show error as "Cannot restore. Multiple definitions for libraries: jquery-validate". Kindly help me out
How to implement client side validation for custom validation attributes.
ReplyDelete