Suggested Videos
Part 66 - Angular httpclient error handling | Text | Slides
Part 67 - Handling angular resolver errors | Text | Slides
Part 68 - Angular httpclient post example | Text | Slides
In this video we will discuss updating data on the server using Angular HttpClient service.
We update data by issuing a PUT request. To issue a PUT request, we use HttpClient service put() method.
In employee.service.ts file, include the following updateEmployee() method
Please note: When an item is updated, by default we get the http status code 204 no content
Method to get an employee by id
Method to add a new employee
Modify canActivate() method in employee-details-guard.service.ts file as shown below.
Modify code in saveEmployee() method in create-employee.component.ts file as shown below.
Also modify code in getEmployee() method in create-employee.component.ts file to subscribe to the employee service
Modify ngOnInit() method in employee-details.component.ts
On the edit page you may get the following error. We get this error because the template is trying to bind to the name property before the server has returned the data, and the employee object is initialised. So that is the reason we cannot read name property from an undefined employee object.
Cannot read property 'name' of undefined
To fix this error, use the following *ngIf directive in create-employee.component.html file.. Now <div> element and it's children will be rendered only after the employee object is initialized.
Do the same thing in employee-details.component.html file.
Part 66 - Angular httpclient error handling | Text | Slides
Part 67 - Handling angular resolver errors | Text | Slides
Part 68 - Angular httpclient post example | Text | Slides
In this video we will discuss updating data on the server using Angular HttpClient service.
We update data by issuing a PUT request. To issue a PUT request, we use HttpClient service put() method.
In employee.service.ts file, include the following updateEmployee() method
baseUrl = 'http://localhost:3000/employees';
// When an update is peformed our server side service does not return
anything
// So we have set the return type to void.
updateEmployee(employee: Employee): Observable<void> {
// We are using the put() method to
issue a PUT request
// We are using template literal syntax
to build the url to which
// the request must be issued. To the
base URL we are appending
// id of the employee we want to
update. In addition to the URL,
// we also pass the updated employee
object, and Content-Type header
// as parameters to the PUT method
return this.httpClient.put<void>(`${this.baseUrl}/${employee.id}`, employee, {
headers: new HttpHeaders({
'Content-Type': 'application/json'
})
})
.pipe(catchError(this.handleError));
}
Please note: When an item is updated, by default we get the http status code 204 no content
Method to get an employee by id
getEmployee(id: number): Observable<Employee> {
return this.httpClient.get<Employee>(`${this.baseUrl}/${id}`)
.pipe(catchError(this.handleError));
}
Method to add a new employee
addEmployee(employee: Employee): Observable<Employee> {
return this.httpClient.post<Employee>(this.baseUrl, employee, {
headers: new HttpHeaders({
'Content-Type': 'application/json'
})
})
.pipe(catchError(this.handleError));
}
Modify canActivate() method in employee-details-guard.service.ts file as shown below.
canActivate(route:
ActivatedRouteSnapshot, state: RouterStateSnapshot)
: Observable<boolean> {
return this._employeeService.getEmployee(+route.paramMap.get('id'))
.pipe(
map(employee =>
{
const
employeeExists = !!employee;
if
(employeeExists) {
return
true;
} else
{
this._router.navigate(['notfound']);
return
false;
}
}),
catchError((err) =>
{
console.log(err);
return
Observable.of(false);
})
);
}
Modify code in saveEmployee() method in create-employee.component.ts file as shown below.
saveEmployee(empForm: NgForm): void {
if (this.employee.id == null) {
console.log(this.employee);
this._employeeService.addEmployee(this.employee).subscribe(
(data: Employee) => {
console.log(data);
empForm.reset();
this._router.navigate(['list']);
},
(error: any) => { console.log(error); }
);
} else {
this._employeeService.updateEmployee(this.employee).subscribe(
() => {
empForm.reset();
this._router.navigate(['list']);
},
(error: any) => { console.log(error); }
);
}
}
Also modify code in getEmployee() method in create-employee.component.ts file to subscribe to the employee service
private getEmployee(id: number) {
if (id === 0) {
this.employee = {
id: null, name: null, gender: null, contactPreference: null,
phoneNumber: null, email: '', dateOfBirth: null, department: null,
isActive: null, photoPath: null
};
this.createEmployeeForm.reset();
this.panelTitle = 'Create Employee';
} else {
this._employeeService.getEmployee(id).subscribe(
(employee) => { this.employee = employee; },
(err: any) => console.log(err)
);
this.panelTitle = 'Edit Employee';
}
}
Modify ngOnInit() method in employee-details.component.ts
ngOnInit() {
this._route.paramMap.subscribe(params => {
this._id = +params.get('id');
this._employeeService.getEmployee(this._id).subscribe(
(employee) => this.employee = employee,
(err: any) => console.log(err)
);
});
}
On the edit page you may get the following error. We get this error because the template is trying to bind to the name property before the server has returned the data, and the employee object is initialised. So that is the reason we cannot read name property from an undefined employee object.
Cannot read property 'name' of undefined
To fix this error, use the following *ngIf directive in create-employee.component.html file.. Now <div> element and it's children will be rendered only after the employee object is initialized.
<div class="panel panel-primary" *ngIf="employee">
Do the same thing in employee-details.component.html file.
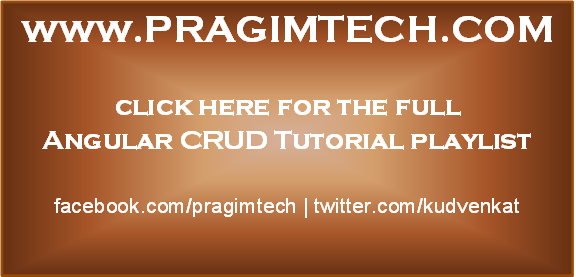
getting error on canActivate Type 'Observable' is not assignable to type 'Observable'.
ReplyDeleteType 'boolean | {}' is not assignable to type 'boolean'.
Type '{}' is not assignable to type 'boolean'.
// Changed in Angular 9: 'Observable.of()' is replaced by 'of()' => Import statement 'import { Observable, of } from 'rxjs';'
Deletereturn of(false);
Can you please give me link for How to reset password base on old password of users in Angular 6 . am not able to find this thing anywhere
ReplyDelete