Suggested Videos
Part 53 - Create observable from array | Text | Slides
Part 54 - Angular resolve guard | Text | Slides
Part 55 - Angular router navigation events | Text | Slides
In this video we will discuss how to display a loading indicator if there is a delay when navigating from one route to another route in an angular application. This is continuation to Part 55. Please watch Part 55 from Angular CRUD tutorial before proceeding.
At the moment in our application, when we navigate to the LIST route, it will take 2 seconds to pre-fetch data required for the LIST route. This is because, we have a route resolver configured on the LIST route. We implemented this route resolver in Part 54 of Angular CRUD tutorial.
During the 2 seconds wait time, while the route resolve guard is busy retrieving the required data, we want to display a loading indicator, so the user knows the application is busy processing the request and he does not end up clicking on the link multiple times.
To implement the loading indicator, we are going to make use of the Angular Router Navigation events. We discussed these events in our previous video. These navigation events range from when the navigation starts and ends to many points in between. When the navigation starts, we want to show the loading indicator, and when the navigation ends, hide the loading indicator.
To be able to react and execute some code in response to the router navigation events, subscribe to the Angular router events observable.
Step 1 : Modify the code in Root Component (AppComponent) in app.component.ts as shown below.
Step 2 : Bind to the showLoadingIndicator property in the view template of our root component i.e AppComponent in app.component.html file.
Step 3 : We are using CSS animations to get the effect of a loading spinner. Place the following CSS in app.component.css file
The following website has different loading spinners.
https://loading.io/css/
We have to slightly modify some of the CSS properties to be able to use them on our list page. I made the following changes.
Part 53 - Create observable from array | Text | Slides
Part 54 - Angular resolve guard | Text | Slides
Part 55 - Angular router navigation events | Text | Slides
In this video we will discuss how to display a loading indicator if there is a delay when navigating from one route to another route in an angular application. This is continuation to Part 55. Please watch Part 55 from Angular CRUD tutorial before proceeding.
At the moment in our application, when we navigate to the LIST route, it will take 2 seconds to pre-fetch data required for the LIST route. This is because, we have a route resolver configured on the LIST route. We implemented this route resolver in Part 54 of Angular CRUD tutorial.
During the 2 seconds wait time, while the route resolve guard is busy retrieving the required data, we want to display a loading indicator, so the user knows the application is busy processing the request and he does not end up clicking on the link multiple times.

To implement the loading indicator, we are going to make use of the Angular Router Navigation events. We discussed these events in our previous video. These navigation events range from when the navigation starts and ends to many points in between. When the navigation starts, we want to show the loading indicator, and when the navigation ends, hide the loading indicator.
To be able to react and execute some code in response to the router navigation events, subscribe to the Angular router events observable.
Step 1 : Modify the code in Root Component (AppComponent) in app.component.ts as shown below.
import { Component, } from '@angular/core';
// Import the Router and navigation events
import {
Router, NavigationStart,
NavigationEnd,
NavigationCancel,
NavigationError, Event
} from '@angular/router';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'app';
// We will use this property to show or
hide
// the loading indicator
showLoadingIndicator = true;
// Inject the Angular Router
constructor(private _router: Router) {
// Subscribe to the router events
observable
this._router.events.subscribe((routerEvent: Event) => {
// On NavigationStart, set
showLoadingIndicator to ture
if (routerEvent instanceof NavigationStart) {
this.showLoadingIndicator = true;
}
// On NavigationEnd or NavigationError
or NavigationCancel
// set showLoadingIndicator to false
if (routerEvent instanceof NavigationEnd ||
routerEvent instanceof NavigationError ||
routerEvent instanceof NavigationCancel) {
this.showLoadingIndicator = false;
}
});
}
}
Step 2 : Bind to the showLoadingIndicator property in the view template of our root component i.e AppComponent in app.component.html file.
<div class="container">
<nav class="navbar navbar-default">
<ul class="nav navbar-nav">
<li>
<a routerLink="list" queryParamsHandling="preserve">List</a>
</li>
<li>
<a routerLink="create">Create</a>
</li>
<li>
<a [routerLink]="['employees',2]">
Get Employee
with Id 2
</a>
</li>
</ul>
</nav>
<router-outlet>
</router-outlet>
<!-- Bind to showLoadingIndicator
property in the component class -->
<div *ngIf="showLoadingIndicator" class="spinner"></div>
</div>
Step 3 : We are using CSS animations to get the effect of a loading spinner. Place the following CSS in app.component.css file
.spinner {
border: 16px solid silver;
border-top: 16px solid #337AB7;
border-radius: 50%;
width: 80px;
height: 80px;
animation: spin 700ms linear infinite;
top:50%;
left:50%;
position: absolute;
}
@keyframes spin {
0% { transform: rotate(0deg) }
100% { transform: rotate(-360deg) }
}
The following website has different loading spinners.
https://loading.io/css/
We have to slightly modify some of the CSS properties to be able to use them on our list page. I made the following changes.
- Removed display property
- Changed position property value from relative to fixed
- Add top and left properties and set them to 50%
- Changed background property value to #337AB7;

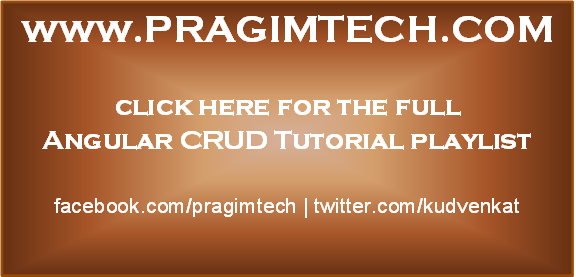
Dear Sir ..Kindly tell me about routes.
ReplyDeletei mean when i login redirect to home
there 3 nav bars admin panel .account,manufacture.
when i click on one of them .my navbars hide and i want navbar remain .. what should i do
hi sir you are binding the spinner for links but i need it for events, could you please provide me an example if possible
ReplyDelete