Suggested Videos
Part 33 - Pass data from parent to child component in angular | Text | Slides
Part 34 - Angular component input property change detection | Text | Slides
Part 35 - Angular input change detection using property setter | Text | Slides
In our previous 2 videos we discussed 2 approaches (ngOnChanges and Property Setter) to detect and react to input property changes in Angular. In this video we will discuss the difference between these 2 approaches and when to use one over the other.
Both these approaches have their own use cases. Your software requirement determines which approach to choose. Let us understand this with an example.
Let us say your child component has 5 input properties. If any of the input properties change, then your requirement is to log those changes. This can be very easily achieved using ngOnChanges life cycle hook. The ngOnChanges life cycle hook is invoked when any of the input properties change.
Each input property that has changed will be attached to the SimpleChanges object using the property name as the key. So if you have 5 input properties, and if 3 out of those 5 properties change, then those 3 properties will be attached to the SimpleChanges object using the property name as the key.
So in short, with ngOnChanges you have access to all input property changes at one place.
The following code logs all the input property changes to the browser console.
To achieve this exact same thing (i.e logging if any of the 5 input properties change) with a property setter, is a bit tedious because you have to have that logging code in every property setter. So if you want to capture multiple property changes, I prefer ngOnChanges life cycle hook as we get all the changes instead of just the changes related to a single property. On the other hand, if you are interested in a single property, then I would use a property setter instead.
ngOnChanges
Part 33 - Pass data from parent to child component in angular | Text | Slides
Part 34 - Angular component input property change detection | Text | Slides
Part 35 - Angular input change detection using property setter | Text | Slides
In our previous 2 videos we discussed 2 approaches (ngOnChanges and Property Setter) to detect and react to input property changes in Angular. In this video we will discuss the difference between these 2 approaches and when to use one over the other.
Both these approaches have their own use cases. Your software requirement determines which approach to choose. Let us understand this with an example.
Let us say your child component has 5 input properties. If any of the input properties change, then your requirement is to log those changes. This can be very easily achieved using ngOnChanges life cycle hook. The ngOnChanges life cycle hook is invoked when any of the input properties change.
Each input property that has changed will be attached to the SimpleChanges object using the property name as the key. So if you have 5 input properties, and if 3 out of those 5 properties change, then those 3 properties will be attached to the SimpleChanges object using the property name as the key.
So in short, with ngOnChanges you have access to all input property changes at one place.
The following code logs all the input property changes to the browser console.
ngOnChanges(changes: SimpleChanges) {
for (const propName of
Object.keys(changes)) {
const change = changes[propName];
const from =
JSON.stringify(change.previousValue);
const
to = JSON.stringify(change.currentValue);
console.log(propName + ' changed from '
+ from + ' to ' + to);
}
}
To achieve this exact same thing (i.e logging if any of the 5 input properties change) with a property setter, is a bit tedious because you have to have that logging code in every property setter. So if you want to capture multiple property changes, I prefer ngOnChanges life cycle hook as we get all the changes instead of just the changes related to a single property. On the other hand, if you are interested in a single property, then I would use a property setter instead.
private
_employeeId: number;
@Input()
set employeeId(val:
number) {
console.log('employeeId changed from '
+ JSON.stringify(this._employeeId)
+ ' to ' +
JSON.stringify(val));
this._employeeId = val;
}
get employeeId(): number
{
return this._employeeId;
}
private
_employee: Employee;
@Input()
set employee(val:
Employee) {
console.log('employee changed from '
+ JSON.stringify(this._employee)
+ ' to ' +
JSON.stringify(val));
this._employee = val;
}
get employee(): Employee
{
return this._employee;
}
ngOnChanges
- We get all the changes instead of just the changes related to a single property
- Useful when multiple properties change
- Property setter is specific to a given property, so we only get changes of that specific property
- Useful when you want to keep track of a single property
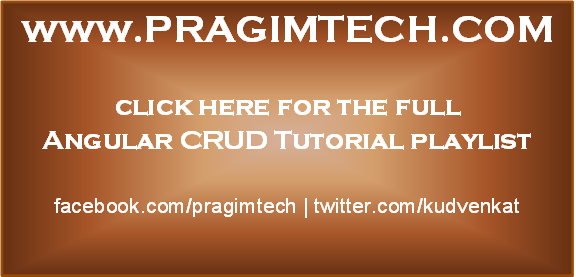
No comments:
Post a Comment
It would be great if you can help share these free resources