Suggested Videos
Part 21 - Add required attribute dynamically in angular | Text | Slides
Part 22 - Angular checkbox validation | Text | Slides
Part 23 - Angular select list validation | Text | Slides
In this video we will discuss how to validate a select element if it has a custom option like one of the following.
Consider the following example :
Code explanation :
<option disabled [ngValue]="null">Select Department</option>
In a real world application, most of the time we load the SELECT list options from a database table. In some case we may also load the default option (like SELECT DEPARTMENT etc) also from the database. In this case the default option value may not be NULL. Depending on your use case it could be -1, or SELECT or something else. So in scenarios like this, built-in required validator does not work with the SELECT element. To make it work, we have to implement our own custom required validator. We will discuss how to do this in our next video.
Part 21 - Add required attribute dynamically in angular | Text | Slides
Part 22 - Angular checkbox validation | Text | Slides
Part 23 - Angular select list validation | Text | Slides
In this video we will discuss how to validate a select element if it has a custom option like one of the following.
- Please Select
- Select Department
- Etc...
Consider the following example :
<div class="form-group"
[class.has-error]="department.touched
&& department.invalid">
<label for="department" class="control-label">Department</label>
<select required #department="ngModel" name="department"
[(ngModel)]="employee.department" id="department"
class="form-control">
<option [ngValue]="null">Select Department</option>
<option *ngFor="let dept of departments" [value]="dept.id">
{{dept.name}}
</option>
</select>
<span class="help-block"
*ngIf="department.touched && department.errors?.required">
Department is required
</span>
</div>
Code explanation :
- <option [ngValue]="null">Select Department</option>. Notice we are using ngValue instead of value. If you use value, null is treated as a string and not as a null. Hence the required validation does not work. Along with using ngValue, also make sure you set the department property on the employee model object to null.
- <option *ngFor="let dept of departments" [value]="dept.id">{{dept.name}}</option>. Here we are using value instead of ngValue, because we just want the selected department id as a string. If you want the department object itself instead of just the department id string, then use ngValue.
- <option *ngFor="let dept of departments" [ngValue]="dept">{{dept.name}}</option>. In this example we are using ngValue and binding it to the dept object. If we select a department now, we get the selected department object.
"department": { "id": 3, "name": "IT" } - Use the disabled attribute, if you do not want the user to be able to select the "Select Department" option.
<option disabled [ngValue]="null">Select Department</option>
<option disabled [ngValue]="null">Select Department</option>
In a real world application, most of the time we load the SELECT list options from a database table. In some case we may also load the default option (like SELECT DEPARTMENT etc) also from the database. In this case the default option value may not be NULL. Depending on your use case it could be -1, or SELECT or something else. So in scenarios like this, built-in required validator does not work with the SELECT element. To make it work, we have to implement our own custom required validator. We will discuss how to do this in our next video.
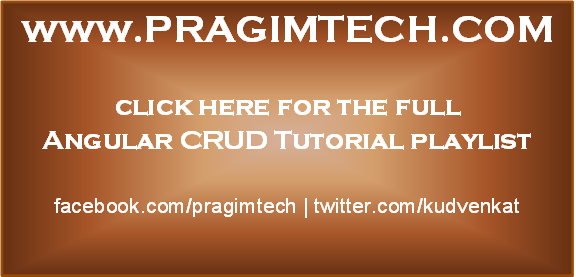
Is it possible to show "select department" text in drop down by default when form load. because now its empty text.
ReplyDelete