Suggested Videos
Part 19 - Angular regular expression validation | Text | Slides
Part 20 - Angular radio button validation | Text | Slides
Part 21 - Add required attribute dynamically in angular | Text | Slides
In this video we will discuss checkbox validation in Angular with example.
We want to make the following "Is Active" check box a required field. If the checkbox is not checked, we want to validate and display "Is Active is required" validation error message. As soon as the checkbox is checked, the validation error message should disappear.
Consider the following HTML
Code Explanation :
What if the employee is terminated or resigned? In that case we do not want the checkbox to be checked. But at the moment, the required validator is forcing us to have the checkbox checked. To fix this modify the required attribute as shown below. Notice, we are binding a boolean expression to the required attribute. If the expression is true the required validator is attached, otherwise it is removed.
[required]="employee.isActive==null"
With this change
Part 19 - Angular regular expression validation | Text | Slides
Part 20 - Angular radio button validation | Text | Slides
Part 21 - Add required attribute dynamically in angular | Text | Slides
In this video we will discuss checkbox validation in Angular with example.
We want to make the following "Is Active" check box a required field. If the checkbox is not checked, we want to validate and display "Is Active is required" validation error message. As soon as the checkbox is checked, the validation error message should disappear.

Consider the following HTML
<div class="form-group" [class.has-error]="isActive.invalid
&& isActive.touched">
<div class="form-control">
<label class="checkbox-inline control-label">
<input type="checkbox" required name="isActive"
#isActive="ngModel" [(ngModel)]="employee.isActive">
Is Active
</label>
</div>
<span class="help-block"
*ngIf="isActive.errors?.required && isActive.touched">
Is Active is required
</span>
</div>
Code Explanation :
- The required attribute makes "Is Active" field required.
- #isActive="ngModel". This creates a template reference variable. We can now this variable (isActive) to check if the field is invalid, touched, dirty etc.
- [class.has-error]="isActive.invalid && isActive.touched". This class binding adds the has-error bootstrap css class when the field is invalid and touched and removes it when the field is valid. This class is used for styling the validation error messages.
- On the label element that displays the static text "Is Active" we have "control-label" class. This class turns the text "Is Active" to red when there is a validation error.
- *ngIf="isActive.errors?.required && isActive.touched". Notice the *ngIf structural directive on the span element. If the "Is Active" field fails required validation and touched, the span element is added to the DOM, else it is removed. The Bootstrap help-block class on the span element is for styling.
At this point,
- If you tab into the checkbox control and leave it, without checking it, you will see the validation error message
- If you select the checkbox box, the error goes away
- If you unselecet the checkbox, the required validation error appears again
This implementation of the checkbox validation is useful, when you want to force the user to select a checkbox. For example, on many web sites, you might have seen a checkbox with the following text. Only when you agree by checking that checkbox, you will be able to proceed. Otherwise you will have to cancel that specific action.
I Agree to the terms and conditionsWhat if the employee is terminated or resigned? In that case we do not want the checkbox to be checked. But at the moment, the required validator is forcing us to have the checkbox checked. To fix this modify the required attribute as shown below. Notice, we are binding a boolean expression to the required attribute. If the expression is true the required validator is attached, otherwise it is removed.
[required]="employee.isActive==null"
With this change
- When the form first loads, isActive property on the employee object is null. So the required attribute is attached to the checkbox.
- If we tab into the checkbox and levae it without selecting it, we see the required validation error message as expected
- If we select the checkbox box, the error goes away
- If we unselecet the checkbox, notice we don't get the required validation. This is because, when the checkbox is unchecked, the value of isActive property on the employee object is false and not NULL. So the boolean expression bound to the required attribute returns false. Hence the required attribute is removed from the checkbox field and we do not see the required validation error.
At the moment, the user interface is confusing. If the employee you are creating is not active, you have to first check the (Is Active) checkbox and then un-check it. To make this less confusing there 2 options for us.
- Remove the required validator on the (Is Active) checkbox, and treat NULL as false.
- Use 2 radio buttons (Yes or No), instead of a single checkbox.
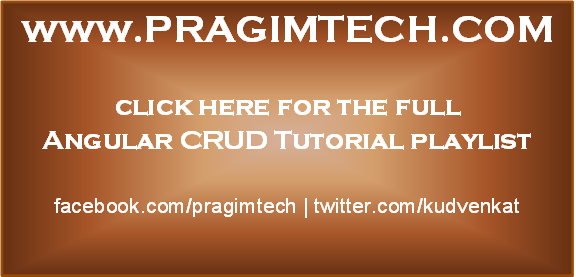
No comments:
Post a Comment
It would be great if you can help share these free resources