Suggested Videos
Part 20 - Angular radio button validation | Text | Slides
Part 21 - Add required attribute dynamically in angular | Text | Slides
Part 22 - Angular checkbox validation | Text | Slides
In this video, we will discuss Dropdown list validation in Angular with example.
Example : We want to make "Department" Dropdownlist a required field. If a department is not selected, we want to validate and display "Department is required" validation error message. As soon as a department is checked, the validation error message should disappear.
Consider the following HTML :
Code Explanation :
In the component class (create-employee.component.ts), initialise department property with a value of '-1'. This will ensure that, when the "Department" dropdownlist is loaded, the first default option 'Select Department' is selected.
employee: Employee = {
id: null,
name: null,
gender: null,
contactPreference: null,
phoneNumber: null,
email: '',
dateOfBirth: null,
department: '-1',
isActive: null,
photoPath: null
};
At this point, view the page in the browser. The dropdownlist REQUIRED validation does not work as expected. The default first option, 'Select Department' is treated as a valid department selection. We will discuss, how to fix this in our next video.
Part 20 - Angular radio button validation | Text | Slides
Part 21 - Add required attribute dynamically in angular | Text | Slides
Part 22 - Angular checkbox validation | Text | Slides
In this video, we will discuss Dropdown list validation in Angular with example.
Example : We want to make "Department" Dropdownlist a required field. If a department is not selected, we want to validate and display "Department is required" validation error message. As soon as a department is checked, the validation error message should disappear.

Consider the following HTML :
<div class="form-group"
[class.has-error]="department.touched
&& department.invalid">
<label for="department" class="control-label">Department</label>
<select id="department" required #department="ngModel"
name="department" [(ngModel)]="employee.department"
class="form-control">
<option *ngFor="let dept of departments" [value]="dept.id">
{{dept.name}}
</option>
</select>
<span class="help-block"
*ngIf="department.touched && department.invalid">
Department is required
</span>
</div>
Code Explanation :
- The required attribute makes "Department" field required.
- #department="ngModel". This creates a template reference variable. We can now this variable (department) to check if the field is invalid, touched, dirty etc.
- [class.has-error]="department.touched && department.invalid". This class binding adds the has-error bootstrap css class when the field is invalid and touched and removes it when the field is valid. This class is used for styling the validation error messages.
- On the label element that displays the static text "Department" we have "control-label" class. This class turns the text "Department" to red when there is a validation error.
- *ngIf="department.touched && department.invalid". Notice the *ngIf structural directive on the span element. If the "Department" field fails required validation and touched, the span element is added to the DOM, else it is removed. The Bootstrap help-block class on the span element is for styling.
At this point, the dropdown list validation works as expected. However, in most of the real world applications, you might see one of the following options as the first option in a dropdown list.
- Please select
- Select Department
- etc...
Modify the HTML to include "Select Department" as the first option. Notice the value of this option is set to '-1', to indicate that it is not a valid department selection. The change is highlighted in YELLOW.
<div
class="form-group"
[class.has-error]="department.touched
&&
department.invalid">
<label for="department"
class="control-label">
Department
</label>
<select id="department"
required #department="ngModel"
name="department"
[(ngModel)]="employee.department"
class="form-control">
<option value="-1">Select
Department</option>
<option *ngFor="let
dept of departments" [value]="dept.id">
{{dept.name}}
</option>
</select>
<span class="help-block"
*ngIf="department.touched
&&
department.invalid">
Department is required
</span>
</div>
In the component class (create-employee.component.ts), initialise department property with a value of '-1'. This will ensure that, when the "Department" dropdownlist is loaded, the first default option 'Select Department' is selected.
employee: Employee = {
id: null,
name: null,
gender: null,
contactPreference: null,
phoneNumber: null,
email: '',
dateOfBirth: null,
department: '-1',
isActive: null,
photoPath: null
};
At this point, view the page in the browser. The dropdownlist REQUIRED validation does not work as expected. The default first option, 'Select Department' is treated as a valid department selection. We will discuss, how to fix this in our next video.
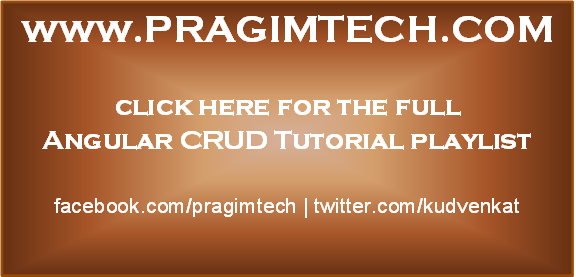
No comments:
Post a Comment
It would be great if you can help share these free resources