Suggested Videos
Part 17 - Model binding in angular template driven forms | Text | Slides
Part 18 - Angular email validation example | Text | Slides
Part 19 - Angular regular expression validation | Text | Slides
In this video we will discuss radio button validation in Angular with example.
Example : Gender is a required field. If one of the gender radio button is not checked, we want to validate and display "Gender is required" validation error message.
As soon as one of the "Gender" radio button is selected, the validation error message should disappear.
Here is the HTML that makes this possible.
Code Explanation :
Part 17 - Model binding in angular template driven forms | Text | Slides
Part 18 - Angular email validation example | Text | Slides
Part 19 - Angular regular expression validation | Text | Slides
In this video we will discuss radio button validation in Angular with example.
Example : Gender is a required field. If one of the gender radio button is not checked, we want to validate and display "Gender is required" validation error message.

As soon as one of the "Gender" radio button is selected, the validation error message should disappear.

Here is the HTML that makes this possible.
<div class="form-group" [class.has-error]="gender.invalid">
<label class="control-label">Gender</label>
<div class="form-control">
<label class="radio-inline">
<input type="radio" name="gender" required #gender="ngModel"
value="male" [(ngModel)]="employee.gender"> Male
</label>
<label class="radio-inline">
<input type="radio" name="gender" required #gender="ngModel"
value="female" [(ngModel)]="employee.gender"> Female
</label>
</div>
<span class="help-block" *ngIf="gender.invalid">
Gender is required
</span>
</div>
Code Explanation :
- Notice we have required attribute on both the radio buttons (Male and Female). This attribute makes the "Gender" field required.
- #gender="ngModel". This creates a template reference variable. We can now this variable (gender) to check if the field is invalid. Notice #gender="ngModel" is placed on the both the radio buttons.
- [class.has-error]="gender.invalid". This class binding adds the has-error bootstrap css class when the field is invalid and removes it when the field is valid. This class is used for styling the validation error messages.
- On the label element that displays the static text "Gender" we have "control-label" class. This class turns the text "Gender" to red when there is a validation error.
- *ngIf="gender.invalid". Notice the *ngIf structural directive on the span element. If the gender field is invalid the span element is added to the DOM, else it is removed. The Bootstrap help-block class on the span element is for styling.
The span element that displays the validation error message can also be coded as shown below. Notice, instead of using "gender.invalid" as the expression for *ngIf, we are using "gender.errors?.required". When the required validation fails, Angular attaches the required key to the errors collection property of the gender field. The key is removed if the field passes validation. So we can check for the existence of this key, to control the display of the validation error message.
<span class="help-block" *ngIf="gender.errors?.required">
Gender is required
</span>
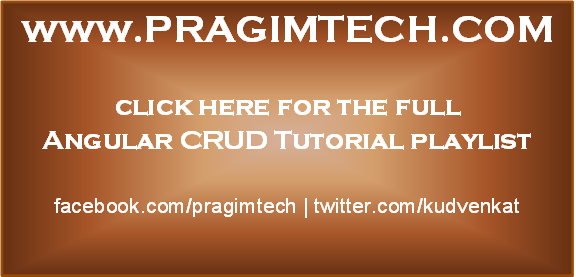
This comment has been removed by the author.
ReplyDeletePlease Make Ionic 4 Tutorials sir
ReplyDelete