Suggested Videos
Part 18 - Angular email validation example | Text | Slides
Part 19 - Angular regular expression validation | Text | Slides
Part 20 - Angular radio button validation | Text | Slides
In this video we will discuss, how to add required attribute dynamically in template driven forms. In our upcoming videos we will discuss how to do the same in reactive forms.
Example : Consider the following 3 fields.
If "Email" is selected as the "Contact Preference", then "Email" input field is required.
If "Phone" is selected as the "Contact Preference", then "Phone" input field is required.
Contact Preference radio buttons HTML :
Code Explanation :
Code explanation :
Part 18 - Angular email validation example | Text | Slides
Part 19 - Angular regular expression validation | Text | Slides
Part 20 - Angular radio button validation | Text | Slides
In this video we will discuss, how to add required attribute dynamically in template driven forms. In our upcoming videos we will discuss how to do the same in reactive forms.
Example : Consider the following 3 fields.

If "Email" is selected as the "Contact Preference", then "Email" input field is required.

If "Phone" is selected as the "Contact Preference", then "Phone" input field is required.
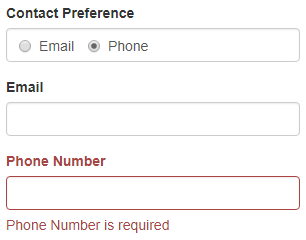
Contact Preference radio buttons HTML :
<div
class="form-group"
[class.has-error]="contactPreference.invalid">
<label class="control-label">Contact
Preference</label>
<div class="form-control">
<label class="radio-inline">
<input type="radio"
required #contactPreference="ngModel"
name="contactPreference"
value="email"
[(ngModel)]="employee.contactPreference">
Email
</label>
<label class="radio-inline">
<input type="radio"
required #contactPreference="ngModel"
name="contactPreference"
value="phone"
[(ngModel)]="employee.contactPreference">
Phone
</label>
</div>
<span class="help-block"
*ngIf="contactPreference.errors?.required">
Contact Preference is required
</span>
<!-- Delete the below line after you see
the selected value in the browser -->
Contact Preference Selected Value : {{ contactPreference.value }}
</div>
Code Explanation :
- We made the contact preference field required, by including required attribute on both email and phone radio buttons.
- At this point view the page in the browser and notice the selected radio button value. When email radio button is selected we see the selected value as email, and when phone radio button is selected, we see the selected value as phone.
- We will use this selected contact preference radio button value (contactPreference.value) to dynamically add or remove required attribute to phone and email input fields.
Email input field HTML :
<div
class="form-group"
[class.has-error]="email.invalid">
<label for="email"
class="control-label">Email</label>
<input id="email"
[required]="contactPreference.value=='email'"
type="text"
class="form-control"
pattern="^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$"
[(ngModel)]="employee.email"
#email="ngModel"
name="email">
<span class="help-block"
*ngIf="email.errors?.required">
Email is required
</span>
<span class="help-block"
*ngIf="email.errors?.pattern
&& email.touched">
Email is Invalid
</span>
</div>
Code explanation :
- Notice the required attribute. We assigned it a boolean expression (contactPreference.value=='email').
- This boolean expression dynamically adds or removes required attribute to the email field depending on whether email contact preference radio button is selected or not.
<div
class="form-group"
[class.has-error]="phoneNumber.invalid">
<label for="phoneNumber"
class="control-label">Phone
Number</label>
<input id="phoneNumber"
[required]="contactPreference.value=='phone'"
#phoneNumber="ngModel"
class="form-control"
type="text"
name="phoneNumber"
[(ngModel)]="employee.phoneNumber">
<span class="help-block"
*ngIf="phoneNumber.errors?.required">
Phone Number is required
</span>
</div>
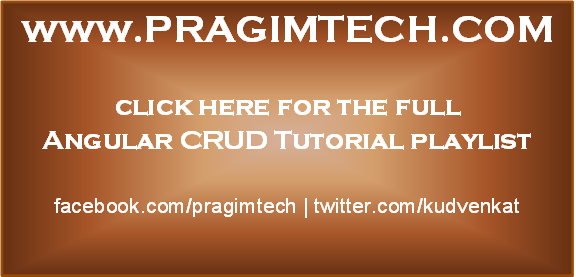
Hi, I watched all video of Angular CRUD, it's fine but I want to login page and login with social sites also, please upload login page with angular 2. Thanks
ReplyDelete