Suggested Videos
Part 13 - Angular ngif directive | Text | Slides
Part 14 - Angular disable browser validation | Text | Slides
Part 15 - Angular form validation | Text | Slides
In this video we will discuss
This is continuation to Part 15. Please watch Part 15 from Angular CRUD tutorial before proceeding.
Here is what we want to do. If the "Full Name" field is not valid we want to style the input field with a red border. The associated label text should also turn red and "Full Name is required" validation error message should be displayed.
Once we type something in the Full Name field, and when it becomes valid, the validation message and the red broder should disappear and also the label text should return to it's normal black colourcolour.
We will be using the Bootstrap framework for styling validation error messages. If you are new to Bootstrap, please check out our Bootstrap tutorial by clicking here.
We discussed Bootstrap form validation states in Part 23 of Bootstrap tutorial. We will use the following Bootstrap classes for styling validation error messages.
Code explanation :
Let's enhance this a bit more. Some users does not like to see the validation error messages, even before they had the opportunity to touch the form field. So what we want to do is,
To take this to the next level, we can style a valid field with a different colour. Here is what I mean.
As you can see these angular validation properties (valid, touched, dirty etc.) provide lot of power and flexibility when validating form fields and displaying validation error messages.
How to disable Submit button if the form is not valid : To disable the "Save" button when the form is not valid, bind the invalid property of the employeeForm template variable to the disabled property of the button.
Part 13 - Angular ngif directive | Text | Slides
Part 14 - Angular disable browser validation | Text | Slides
Part 15 - Angular form validation | Text | Slides
In this video we will discuss
- How to display validation error messages to the user
- Style the error messages using Bootstrap
- How to disable Submit button if the form is not valid
This is continuation to Part 15. Please watch Part 15 from Angular CRUD tutorial before proceeding.
Here is what we want to do. If the "Full Name" field is not valid we want to style the input field with a red border. The associated label text should also turn red and "Full Name is required" validation error message should be displayed.

Once we type something in the Full Name field, and when it becomes valid, the validation message and the red broder should disappear and also the label text should return to it's normal black colourcolour.

We will be using the Bootstrap framework for styling validation error messages. If you are new to Bootstrap, please check out our Bootstrap tutorial by clicking here.
We discussed Bootstrap form validation states in Part 23 of Bootstrap tutorial. We will use the following Bootstrap classes for styling validation error messages.
- has-error
- control-label
- help-block
Modify the "Full name" input filed as shown below.
<div class="form-group" [class.has-error]="fullNameControl.invalid">
<label for="fullName" class="control-label">Full Name</label>
<input id="fullName" required type="text" class="form-control" name="fullName"
[(ngModel)]="fullName" #fullNameControl="ngModel">
<span class="help-block" *ngIf="fullNameControl.invalid">
Full Name is required
</span>
</div>
Code explanation :
- [class.has-error]="fullNameControl.invalid. This is class binding in angular. If the invalid property returns true, then the Bootstrap class has-error is added to the div element, if it is false then the class is removed.
- On the "Full Name" label element we applied control-label Bootstrap class. This class turns the label text to red if there is a validation error.
- *ngIf="fullNameControl.invalid". The *ngIf structural directive on the span element adds or removes the validation error message depending on the invalid property value. If the invalid property is true, then the validation error message is displayed, otherwise it is removed. Also, notice we are using the Bootstrap help-block class on the span element for styling.
At this point, save the changes and view the page in the browser. Notice when the form initially loads, we see the validation error message Full Name is required and it is also styled as expected. As we soon as we start typing, the error goes away. When we delete everything that we have typed, the error appears again. So, it's working as expected.
Let's enhance this a bit more. Some users does not like to see the validation error messages, even before they had the opportunity to touch the form field. So what we want to do is,
- Do not display any validation error messages when the form is initially loaded.
- When the user touches the field, and if he leaves the field without typing in the value, then we want to display the validation error message.
<div class="form-group"
[class.has-error]="fullNameControl.invalid
&& fullNameControl.touched">
<label for="fullName" class="control-label">Full Name</label>
<input id="fullName" required type="text" class="form-control" name="fullName"
[(ngModel)]="fullName" #fullNameControl="ngModel">
<span class="help-block"
*ngIf="fullNameControl.invalid && fullNameControl.touched">
Full Name is required
</span>
</div>
To take this to the next level, we can style a valid field with a different colour. Here is what I mean.

- When the form first loads, the Full Name and it's label are black in colour and the validation error message is not displayed
- When the user touches the field and leaves it without typing anything, the colour changes to red and the validation error message is displayed
- If the user types something, the field is valid, so we want a green border and the label text should also turn green.
To achieve this we can use the Bootstrap has-success class as shown below. As you can see, the has-success class is added when valid property is true and it is removed when it is false.
<div class="form-group"
[class.has-error]="fullNameControl.invalid
&& fullNameControl.touched"
[class.has-success]="fullNameControl.valid">
As you can see these angular validation properties (valid, touched, dirty etc.) provide lot of power and flexibility when validating form fields and displaying validation error messages.
How to disable Submit button if the form is not valid : To disable the "Save" button when the form is not valid, bind the invalid property of the employeeForm template variable to the disabled property of the button.
<button class="btn btn-primary" type="submit"
[disabled]="employeeForm.invalid">Save</button>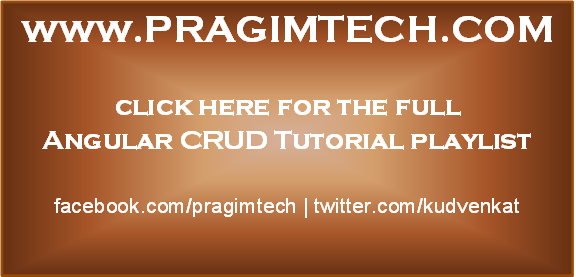
Hi, I have implemented upto binding with fields of model. But its not working for me. As its giving me the error stating that : ERROR Error: If ngModel is used within a form tag, either the name attribute must be set or the form control must be defined as 'standalone' in ngModelOptions. Example 1: input [(ngModel)]="person.firstName" name="first" Example 2: input [(ngModel)]="person.firstName" [ngModelOptions]="{standalone: true}"
ReplyDeletename attribute should always be used along with [(ngModel)].
ReplyDelete