Suggested Videos
Part 16 - Structural Design Patterns Introduction - Text - Slides
Part 17 - Adapter Design Pattern - Text - Slides
Part 18 - Bridge Design Pattern - Text - Slides
In this video we will discuss
Composite Design Pattern : As per the GOF definition, the Composite Pattern, "Compose objects into tree structures to represent part-whole hierarchies. Composite let clients treat individual objects and compositions of objects uniformly.
This means, the composite pattern describes a group of objects that is treated the same way as a single instance of the same type of object.
Implementation Guidelines : We need to Choose Composite Design Pattern when
Client
Hence we can change this representation slightly in a new form as shown below. If we compare previous and the current one notice that we have changed the component methods to have only the one which can be implemented by both leaf and composite nodes.
The following are the steps to implement Composite design pattern
Step 1 : Create an interface IEmployee
Step 2 : Create Employee Class and implement the interface component
Step 3 : Create Manager Class which is the composite and implement the interface component and add the subordinates as shown below.
Step 4 : Above mentioned classes are created under the below folder structure. Again it’s not mandatory to follow this folder structure as we are just doing this for representation purpose.
Step 5 : Switch to the client which is main program and implement the below code
Step 6 : Run the application and notice the below output which implements the composition of employees.
Part 16 - Structural Design Patterns Introduction - Text - Slides
Part 17 - Adapter Design Pattern - Text - Slides
Part 18 - Bridge Design Pattern - Text - Slides
In this video we will discuss
- What is Composite Design Pattern
- Implementation Guidelines of Composite design pattern
- And will take a look at simple example to implement this pattern
Composite Design Pattern : As per the GOF definition, the Composite Pattern, "Compose objects into tree structures to represent part-whole hierarchies. Composite let clients treat individual objects and compositions of objects uniformly.
This means, the composite pattern describes a group of objects that is treated the same way as a single instance of the same type of object.
Implementation Guidelines : We need to Choose Composite Design Pattern when
- Represent part-whole hierarchies of objects.
- Clients to ignore the difference between compositions of objects and individual objects.

Client
- Clients use the Component interface to interact with objects in the
- is the abstraction for all components, including composite ones
- Represents leaf objects in the composition
- Implements all Component methods
- Represents a composite Component (Please note that composite components have children and they could be composite child’s or leafs )
- Implements all Component methods, generally by delegating them to its children
Hence we can change this representation slightly in a new form as shown below. If we compare previous and the current one notice that we have changed the component methods to have only the one which can be implemented by both leaf and composite nodes.

The following are the steps to implement Composite design pattern
Step 1 : Create an interface IEmployee
using System;
using
System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
CompositeDesignPattern.Component
{
//Component
public interface IEmployee
{
void GetDetails(int indentation);
}
}
Step 2 : Create Employee Class and implement the interface component
using
CompositeDesignPattern.Component;
using System;
using
System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
CompositeDesignPattern.Leaf
{
public class Employee : IEmployee
{
public Employee(string name, string dept)
{
this.Name = name;
this.Department =
dept;
}
public string Name { get; set; }
public string Department { get; set; }
public void GetDetails(int indentation)
{
Console.WriteLine(string.Format("{0}-
Name:{1}, Dept:{2} (Leaf) ",
new String('-', indentation),
this.Name.ToString(),
this.Department));
}
}
}
Step 3 : Create Manager Class which is the composite and implement the interface component and add the subordinates as shown below.
using
CompositeDesignPattern.Component;
using System;
using
System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
CompositeDesignPattern.Composite
{
public class Manager : IEmployee
{
public
List<IEmployee> SubOrdinates;
public Manager(string name, string dept)
{
this.Name = name;
this.Department =
dept;
SubOrdinates = new
List<IEmployee>();
}
public string Name { get; set; }
public string Department { get; set; }
public void GetDetails(int indentation)
{
Console.WriteLine();
Console.WriteLine(string.Format("{0}+
Name:{1}, " +
"Dept:{2} - Manager(Composite)",
new String('-', indentation),
this.Name.ToString(),
this.Department));
foreach (IEmployee component in SubOrdinates)
{
component.GetDetails(indentation + 1);
}
}
}
}
Step 4 : Above mentioned classes are created under the below folder structure. Again it’s not mandatory to follow this folder structure as we are just doing this for representation purpose.

Step 5 : Switch to the client which is main program and implement the below code
using
CompositeDesignPattern.Component;
using CompositeDesignPattern.Composite;
using
CompositeDesignPattern.Leaf;
using System;
using
System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CompositeDesignPattern
{
class Program
{
static void Main(string[] args)
{
IEmployee John =
new Employee("John", "IT");
IEmployee Mike =
new Employee("Mike", "IT");
IEmployee Jason
= new Employee("Jason", "HR");
IEmployee Eric =
new Employee("Eric", "HR");
IEmployee Henry
= new Employee("Henry", "HR");
IEmployee James
= new Manager("James", "IT")
{ SubOrdinates =
{ John, Mike } };
IEmployee Philip
= new Manager("Philip", "HR")
{ SubOrdinates =
{ Jason, Eric, Henry } };
IEmployee Bob = new Manager("Bob", "Head")
{ SubOrdinates =
{ James, Philip } };
James.GetDetails(1);
Console.ReadLine();
}
}
}
Step 6 : Run the application and notice the below output which implements the composition of employees.

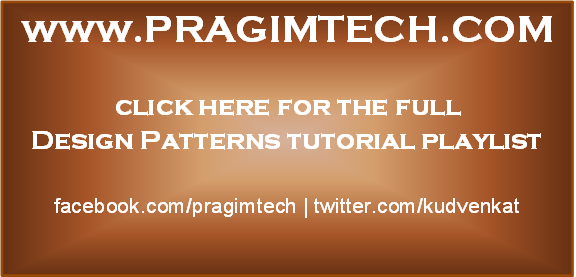
Please add some video related to ASP.NET CORE
ReplyDeleteNice, but you could improve your explanations with correct UML class diagrams. For instance I do not see the reference from 'composite' to the 'Component'. Also the comment is not using the standardised diagram
ReplyDelete