Suggested Videos
Part 7 - Angular radio button checked by default | Text | Slides
Part 8 - Bootstrap checkbox in angular | Text | Slides
Part 9 - Angular bootstrap select list | Text | Slides
In this video, we will discuss, how to get the select list options from an array in the component class, instead of having them hard-coded in the HTML. This is continuation to Part 9. So, please watch Part 9 from Angular CRUD tutorial before proceeding.
Step 1 : Create the Department class.
Add a TypeScript file to the models folder. Name it department.model.ts. Copy and paste the following code. Notice the Department class has 2 properties - id and name of the department.
export class Department {
id: number;
name: string;
}
Step 2 : Import the Department class
Include the following import statement in create-employee.component.ts file
import { Department } from '../models/department.model';
Step 3 : Include the following array of departments in CreateEmployeeComponent class in create-employee.component.ts file
departments: Department[] = [
{ id: 1, name: 'Help Desk' },
{ id: 2, name: 'HR' },
{ id: 3, name: 'IT' },
{ id: 4, name: 'Payroll' }
];
Please note : The "Department" type is not required for the application to work, but it adds great value during development. Using it provides us intellisense, error checking and type saftey.
Step 4 : In create-employee.component.html file, modify the HTML that displays the "Department" dropdownlist as shown below.
Code explanation :
At this point, when we select a department, the respective department id is included in the Angular generated form model. Along the same lines, when we click the "Save" button the respective department id is logged to the console.Part 7 - Angular radio button checked by default | Text | Slides
Part 8 - Bootstrap checkbox in angular | Text | Slides
Part 9 - Angular bootstrap select list | Text | Slides
In this video, we will discuss, how to get the select list options from an array in the component class, instead of having them hard-coded in the HTML. This is continuation to Part 9. So, please watch Part 9 from Angular CRUD tutorial before proceeding.
Step 1 : Create the Department class.
Add a TypeScript file to the models folder. Name it department.model.ts. Copy and paste the following code. Notice the Department class has 2 properties - id and name of the department.
export class Department {
id: number;
name: string;
}
Step 2 : Import the Department class
Include the following import statement in create-employee.component.ts file
import { Department } from '../models/department.model';
Step 3 : Include the following array of departments in CreateEmployeeComponent class in create-employee.component.ts file
departments: Department[] = [
{ id: 1, name: 'Help Desk' },
{ id: 2, name: 'HR' },
{ id: 3, name: 'IT' },
{ id: 4, name: 'Payroll' }
];
Please note : The "Department" type is not required for the application to work, but it adds great value during development. Using it provides us intellisense, error checking and type saftey.
Step 4 : In create-employee.component.html file, modify the HTML that displays the "Department" dropdownlist as shown below.
<div
class="form-group">
<label for="department">Department</label>
<select id="department"
name="department"
[(ngModel)]="department"
class="form-control">
<option *ngFor="let
dept of departments" [value]="dept.id">
{{dept.name}}
</option>
</select>
</div>
Code explanation :
- On the "option" element we are using ngFor structural directive to loop over the array of departments we have in the "departments" property of the component class
- For each "Department" object in the "departments" array, we get an option.
- The option value is the department id and the display text is the department name
- Notice the square brackets around the [value] property. This is property binding in Angular. We discussed property binding in detail in Part 9 of Angular 2 tutorial. If you remove the square brackets the value for each option will be the literal text "dept.id" instead of the department id (1 or 2 or 3 etc.)
- To display the deprtment name we are using interpolation. We discussed interpolation in Part 8 of Angular 2 tutorial.
- Since ngFor is a structural directive there is an asterisk before it.
- Structural directives modify the DOM, i.e they add or remove the elements from DOM. Adding and removing elements from DOM is different from showing and hiding. We will discuss all these in detail in our upcoming videos.
Please note : It is important that we include the ngFor directive on the element that we want to be repeated. In our case we want an option element for each department we have in the array. So we included the ngFor directive on the option element. If we instead include the ngFor directive on the "div" element that has the bootstrap "form-group" class as shown below.
<div
class="form-group"
*ngFor="let dept of
departments">
<label for="department">Department</label>
<select id="department"
name="department"
[(ngModel)]="department"
class="form-control">
<option [value]="dept.id">
{{dept.name}}
</option>
</select>
</div>
We get 4 department dropdownlists. That is one for each department in the array. So it is important we include the ngFor directive on the right element.

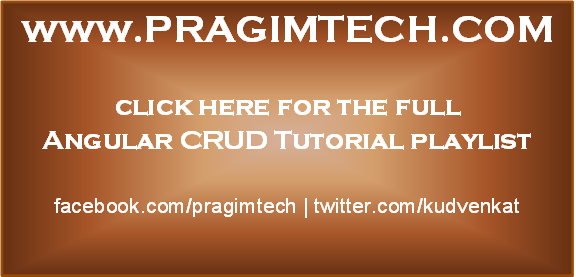
how to make deafult select
ReplyDelete"<select> id="department" class="form-control" name="department" [(ngModel)]="department"
Delete<option> value="0">--Select a Department--</option>
<option> *ngFor="let dept of departments" [value]="dept.id">{{dept.name}}</option>
<select"
Write the below code in create-employee.component.ts , which will select IT as default one with the value as 3.
Deletedepartment : number =3;
how to push element in array.
ReplyDeleteHow to Update Database Value using Dropdown Menu
ReplyDeletehow to hide and show div on select array dropdown value
ReplyDeletehow to call a webapi on change event of drop down
ReplyDeletecan u send me all html ? i need it
ReplyDelete