Suggested Videos
Part 10 - Abstract Factory Design Pattern - Text - Slides
Part 11 - Builder Desing Pattern Implementation - Text - Slides
Part 12 - Builder Design Pattern Implementation - Text - Slides
In this video we will discuss and implement
Business Requirement : Provide an option to choose and build configuration of the system which is allocated to the employees. The configuration options that user can choose are RAM, HDD, USB Mouse etc. Choose the system configurations based on the computer type that we need to build. For example, A laptop users can choose touch screen and the desktop users can configure keyboard and mouse.
Fluent Interface : The idea behind a fluent interface is that one can apply multiple properties to an object by connecting them with dots and without having to re-specify the object each time
C# uses fluent programming extensively in LINQ to build queries using the standard query operators. The implementation is based on extension methods
Fluent Interface Features : Fluent interface is a method for constructing object oriented APIs, where the readability of the source code is close to that of ordinary written prose
Fluent interface is normally implemented by using method cascading (concretely method chaining)
Fluent code is much more readable and allows to vary a product’s internal representation
Fluent Encapsulates code for construction and representation and Provides control over steps of an object construction process
Searching, Sorting, pagination, grouping with a blend of LINQ are some of the real world usage of fluent interface in combination with builder design pattern.
However, it’s not mandatory to implement fluent interfaces with builder design pattern however, the idea of this session is explore and integrate the fluent interface with builder design pattern.
Fluent Builder Implementation
Step 1 : Change the ISystemBuilder Interface methods to return ISystemBuilder
Part 10 - Abstract Factory Design Pattern - Text - Slides
Part 11 - Builder Desing Pattern Implementation - Text - Slides
Part 12 - Builder Design Pattern Implementation - Text - Slides
In this video we will discuss and implement
- Fluent Interface
- Enhance the Builder Implementation with Fluent Implementation
- Builder vs Abstract Factory/Factory
Business Requirement : Provide an option to choose and build configuration of the system which is allocated to the employees. The configuration options that user can choose are RAM, HDD, USB Mouse etc. Choose the system configurations based on the computer type that we need to build. For example, A laptop users can choose touch screen and the desktop users can configure keyboard and mouse.
Fluent Interface : The idea behind a fluent interface is that one can apply multiple properties to an object by connecting them with dots and without having to re-specify the object each time
C# uses fluent programming extensively in LINQ to build queries using the standard query operators. The implementation is based on extension methods
Fluent Interface Features : Fluent interface is a method for constructing object oriented APIs, where the readability of the source code is close to that of ordinary written prose
Fluent interface is normally implemented by using method cascading (concretely method chaining)
Fluent code is much more readable and allows to vary a product’s internal representation
Fluent Encapsulates code for construction and representation and Provides control over steps of an object construction process
Searching, Sorting, pagination, grouping with a blend of LINQ are some of the real world usage of fluent interface in combination with builder design pattern.
However, it’s not mandatory to implement fluent interfaces with builder design pattern however, the idea of this session is explore and integrate the fluent interface with builder design pattern.
Fluent Builder Implementation
Step 1 : Change the ISystemBuilder Interface methods to return ISystemBuilder
using System;
using
System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Web.Builder.IBuilder
{
public interface ISystemBuilder
{
ISystemBuilder
AddMemory(string memory);
ISystemBuilder
AddDrive(string size);
ISystemBuilder
AddKeyBoard(string type);
ISystemBuilder
AddMouse(string type);
ISystemBuilder
AddTouchScreen(string enabled);
ComputerSystem
GetSystem();
}
}
Step 2 : Implement the ISystemBuilder Interface methods in Laptop and Desktop Builder Classes
By returning the ISystemBuilder and by using this as the return statement we are indicating that after assigning the properties of the desktop or laptop we are returning back the same ISystemBuilder to allow the method chaining.
using System;
using
System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Builder.IBuilder;
namespace
Web.Builder.ConcreteBuilder
{
public class LaptopBuilder : ISystemBuilder
{
ComputerSystem laptop =
new ComputerSystem();
public ISystemBuilder
AddDrive(string size)
{
laptop.HDDSize =
size;
return this;
}
public ISystemBuilder
AddKeyBoard(string type)
{
return this;
}
public ISystemBuilder
AddMemory(string memory)
{
laptop.RAM =
memory;
return this;
}
public ISystemBuilder
AddMouse(string type)
{
return this;
}
public ISystemBuilder
AddTouchScreen(string enabled)
{
laptop.TouchScreen = enabled;
return this;
}
public ComputerSystem
GetSystem()
{
return laptop;
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Builder.IBuilder;
namespace
Web.Builder.ConcreteBuilder
{
public class DesktopBuilder : ISystemBuilder
{
ComputerSystem desktop
= new ComputerSystem();
public ISystemBuilder
AddDrive(string size)
{
desktop.HDDSize
= size;
return this;
}
public ISystemBuilder
AddKeyBoard(string type)
{
desktop.KeyBoard
= type;
return this;
}
public ISystemBuilder
AddMemory(string memory)
{
desktop.RAM =
memory;
return this;
}
public ISystemBuilder
AddMouse(string type)
{
desktop.Mouse =
type;
return this;
}
public ISystemBuilder
AddTouchScreen(string enabled)
{
return this;
}
public ComputerSystem
GetSystem()
{
return desktop;
}
}
}
Step 3 : Enhance the Configuration builder with Fluent Implementation
using System;
using
System.Collections.Generic;
using
System.Collections.Specialized;
using System.Linq;
using System.Web;
using Web.Builder.IBuilder;
namespace Web.Builder.Director
{
public class ConfigurationBuilder
{
public void BuildSystem(ISystemBuilder
systembuilder
, NameValueCollection collection)
{
systembuilder.AddDrive(collection["Drive"])
.AddMemory(collection["RAM"])
.AddMouse(collection["Mouse"])
.AddKeyBoard(collection["Keyboard"])
.AddTouchScreen(collection["TouchScreen"]);
}
}
}
Step 4 : Run the application and build the system. Notice that depending on the system type the respective system configurations are built using Fluent Builder implementation.
Builder Vs Factory and Abstract Factory
Builder design pattern encapsulates complex creation into a single method.
Builder design pattern focuses on construction of object in a step by step manner whereas Abstract factory pattern is used to solve problems related to the creation of families of products.
Builder design pattern returns the object after step by step construction of the complex object where as in Abstract factory or Factory pattern, we return the created product immediately.
To conclude, many application designs start out using Factory which is less complex and evolve towards Abstract Factory and Builder as the complexity increases with a demand of flexibility.
In the next video we will discuss Prototype design pattern.
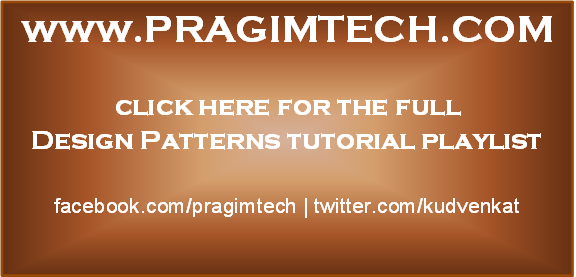
No comments:
Post a Comment
It would be great if you can help share these free resources