Suggested Videos
Part 8 - Factory Design Pattern Introduction - Text - Slides
Part 9 - Factory Method Design Pattern - Text - Slides
Part 10 - Abstract Factory Design Pattern - Text - Slides
In this video we will learn
1. What is Builder Design Pattern
2. Implementation Guidelines
3. Builder design pattern in action
What is Builder Design Pattern : Builder Pattern belongs to creational pattern
Definition : "Separate the construction of a complex object from its representation so that the same construction process can create different representations"
Builder Pattern solves the situation of increasing constructor parameters and constructors of a given class by providing a step by step initialisation of Parameters. After step by step initialisation, it returns the resulting constructed Object at once.
Implementation Guidelines : We need to Choose Builder Design Pattern when
Business Requirement : Provide an option to choose and build configuration of the system which is allocated to the employees. The configuration options that user can choose are RAM, HDD, USB Mouse etc.
If you look at the illustrated diagram of Builder Design Pattern
Step 1 : Add SystemConfigurationDetails to the existing Employee table
Step 2 : Open EmployeePortal.edmx under the Models folder of the solution and update the model from the database (Right click on the model designer and choose update from database option)
Step 3 : Create BuildSystem Action method and generate a view out of it.
Step 4 : Create a new class and name it as computer system with RAM and HDDSize as the constructor parameters and Build as method that builds the system.
Step 5 : To the added BuildSystem view add dropdown lists to choose RAM and HDDSize.
Step 6 : Create an action method BuildSystem to build the system on Submit.
Step 7 : Run the application and Build a system for selected employee
Step 8 : Choose the configurations and click on create to build the system
Though we have achieved the business requirement. The current approach will not fit if we need to add more configurations to the current system and it gets complicated.
Also, if we need to enhance the current code itself to segregate the configurations differently between a laptop and desktop the current approach is not feasible and it becomes complicated.
In these kind of situations builder pattern comes to our rescue.
In the next video let’s discuss how we can address these problems using Builder design pattern.
Part 8 - Factory Design Pattern Introduction - Text - Slides
Part 9 - Factory Method Design Pattern - Text - Slides
Part 10 - Abstract Factory Design Pattern - Text - Slides
In this video we will learn
1. What is Builder Design Pattern
2. Implementation Guidelines
3. Builder design pattern in action
What is Builder Design Pattern : Builder Pattern belongs to creational pattern
Definition : "Separate the construction of a complex object from its representation so that the same construction process can create different representations"
Builder Pattern solves the situation of increasing constructor parameters and constructors of a given class by providing a step by step initialisation of Parameters. After step by step initialisation, it returns the resulting constructed Object at once.
Implementation Guidelines : We need to Choose Builder Design Pattern when
- We need to break up the construction of a complex object
- We need to create a complex object and it should be independent of the parts that make up the object
- The construction process must allow multiple representations of the same class
Business Requirement : Provide an option to choose and build configuration of the system which is allocated to the employees. The configuration options that user can choose are RAM, HDD, USB Mouse etc.
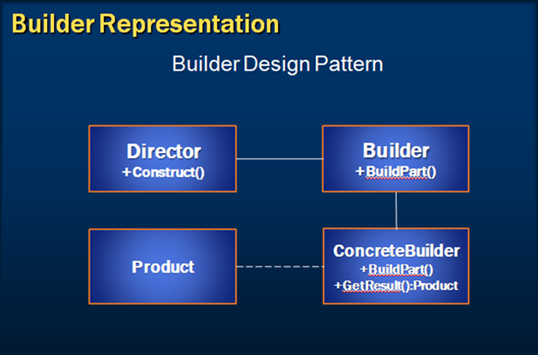
If you look at the illustrated diagram of Builder Design Pattern
- Builder : Defines a template for the steps to construct the product. To simplify, Builder Specifies an abstract interface for creating parts of a Product object.
- Concrete Builder : Implements the builder interface and provides an interface for getting the product.
- Director : Is responsible to constructs the object through the builder interface.
- Product : is the Main object that's constructed and Represents the complex object.
Step 1 : Add SystemConfigurationDetails to the existing Employee table
CREATE TABLE [dbo].[Employee]
(
[Id] INT IDENTITY (1, 1) NOT NULL,
[Name] VARCHAR (50) NOT NULL,
[JobDescription] VARCHAR (50) NOT NULL,
[Number] VARCHAR (50) NOT NULL,
[Department] VARCHAR (50) NOT NULL,
[HourlyPay] DECIMAL (18) NOT NULL,
[Bonus] DECIMAL (18) NOT NULL,
[EmployeeTypeID] INT NOT NULL,
[HouseAllowance] DECIMAL (18) NULL,
[MedicalAllowance]
DECIMAL (18) NULL,
[ComputerDetails] VARCHAR (250) NULL,
[SystemConfigruationDetails] VARCHAR (500) NULL,
PRIMARY KEY CLUSTERED ([Id] ASC),
CONSTRAINT [FK_Employee_EmployeeType]
FOREIGN KEY ([EmployeeTypeID])
REFERENCES [dbo].[Employee_Type] ([Id])
)
Step 2 : Open EmployeePortal.edmx under the Models folder of the solution and update the model from the database (Right click on the model designer and choose update from database option)
Step 3 : Create BuildSystem Action method and generate a view out of it.
[HttpGet]
public ActionResult
BuildSystem(int? employeeID)
{
return View(employeeID);
}
}
Step 4 : Create a new class and name it as computer system with RAM and HDDSize as the constructor parameters and Build as method that builds the system.
public
class ComputerSystem
{
private
string _RAM;
private
string _HDDSize;
public
ComputerSystem()
{
}
public
ComputerSystem(string RAM, string
HDD)
{
_RAM = RAM;
_HDDSize = HDD;
}
public string
Build()
{
StringBuilder
sb = new StringBuilder();
sb.Append(string.Format("
RAM: {0}", _RAM));
sb.Append(string.Format("
HDDSize: {0}", _HDDSize));
return
sb.ToString();
}
}
Step 5 : To the added BuildSystem view add dropdown lists to choose RAM and HDDSize.
Step 6 : Create an action method BuildSystem to build the system on Submit.
[HttpPost]
public ActionResult
BuildSystem(int employeeID, string RAM, string HDDSize)
{
Employee
employee = db.Employees.Find(employeeID);
ComputerSystem
computerSystem = new ComputerSystem(RAM, HDDSize);
employee.SystemConfigurationDetails
= computerSystem.Build();
db.Entry(employee).State
= EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
Step 7 : Run the application and Build a system for selected employee
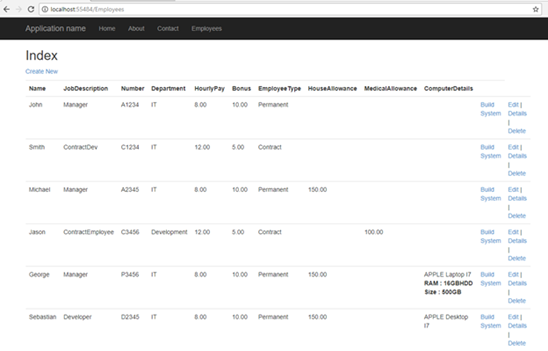
Step 8 : Choose the configurations and click on create to build the system
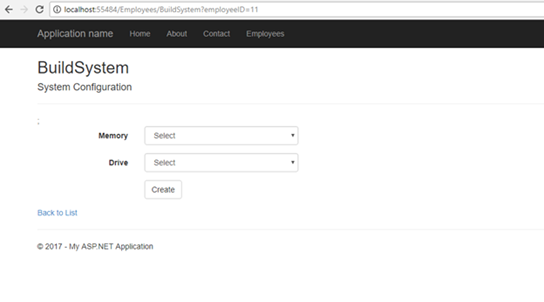
Though we have achieved the business requirement. The current approach will not fit if we need to add more configurations to the current system and it gets complicated.
Also, if we need to enhance the current code itself to segregate the configurations differently between a laptop and desktop the current approach is not feasible and it becomes complicated.
In these kind of situations builder pattern comes to our rescue.
In the next video let’s discuss how we can address these problems using Builder design pattern.
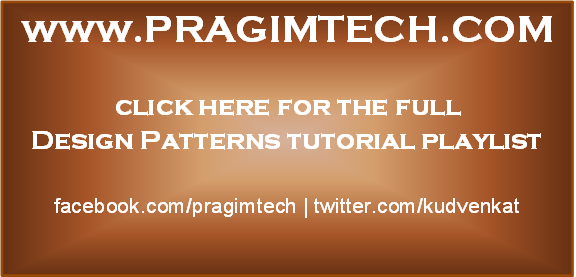
Hello Avish,
ReplyDeleteI followed your tutorial exactly. But at some reason the HDDSize is out of scope (null value) when I click create. How can I find the solution? I get no errors when I run the application.
Problem solved, here the solution:
ReplyDeleteChange "drive" to "HDDSize".
@Html.DropDownList("HDDSize",
new List()
{ ...... }
Hi, I have followed your tutorial but on "Step 3" when you have passed a parameter employeeID from controller to view page then how you have declared the model in view. Can you share view code?
ReplyDeleteBuildSystem.cshtml text code is not available in text verion
ReplyDeletePlease check new video, You will find the BuildSystem.cshtml text code.
Delete